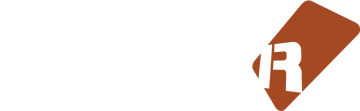
Welcome
... to the Renoise Lua Scripting Guide! This book is intended for developers who want to write their own scripts & tools for Renoise.
If you are only interested in downloading tools for Renoise and not in developing your own tools, please have a look at the Renoise Tools Page.
If you want to develop your own tools for Renoise, just keep reading.
Possibilities
Before you delve into writing your own scripts and tools, it worth considering what is even possible to do with them. In general, you can automate and manage different aspects Renoise, add or modify song data using algorithms or create interfaces to connect with other software or hardware. You can see some examples below about what you can access inside Renoise, for a complete reference, check out the API Definitions.
Process song data
- Generate, modify or filter notes, patterns or phrases
- Access volume, delays and panning values
- Write effect commands and automation
- Rearrange the pattern matrix and more
Manage tracks and instruments
- Create and modify instruments or tracks
- Add built-in DSP devices to tracks or FX chains and set their parameters
- Generate modulation chains on instruments
- Load samples and configure them
- Manage slices and keyzones
Sample generation and mangling
- Generate new samples and change existing ones
- Chop samples and create slices and intruments based on custom rules
- Implement offline audio effects
Custom graphical interfaces
- Use built-in sliders, buttons, switches and more to compose a GUI
- Listen to keyboard and mouse events to define custom behaviour
- Use bitmaps to create unique buttons
- Hook up the interface to the rest of your tool
Controller support
- Write logic for bidirectional communication between Renoise and MIDI controllers
- Implement custom functionality for MIDI control surfaces
- Control Renoise via customized OSC messages
- Exchange information with other software like VJ tools or web services
Interactions
There are a few ways tool creators can make the functionality they provide available for users, below is a brief summary of the most used methods.
- Define keybindings that can be assigned to shortcuts and executed from certain contexts in Renoise
- Add new entries to menus like the Tools menu or one of the right-click context menus
- Create custom views that do things on button presses, slider drags and so on
- Listen to MIDI, OSC or WebSocket messages to execute actions
- React to events inside Renoise like "do something any time a new song is loaded"
Limitations
Finally, let's look at what is not possible via tools.
-
You cannot override the existing behaviour of Renoise. You can add functionality on top of what's already there, but you can't disable or change how the built-in features work.
For example, you can create a completely custom GUI to compose patterns in a novel way but you cannot change how the built-in pattern editor works or looks like. Similarly, you can add a new shortcut that does something extra to the selected sample block or places new slices but you can't modify how the built-in autoslicing works. -
You cannot write real-time DSP code like synths, effects or modulators (except for scripts inside the Formula device), which is not using the Renoise Tool API. If you want to design your own synths and effects you should look into plugin development (using DISTRHO, nih-plug etc.), you could also use existing plugins that allow you to build your own DSP patches (like plugdata or Cardinal). Of course you can generate and modify samples using your tool, but it will have to be implemented as offline rendering instead of real-time processing.
Setting up your development environment
By default Renoise has all scripting utilities hidden to keep it simple for users who don't wish to mess around with code. If you want to write scripts, the first thing you have to do is to enable the hidden development tools.
- For a quick test you can launch the Renoise executable with the
--scripting-dev
argument - To have this mode enabled by default, you'll have to edit your
Config.xml
file inside Renoise's preferences folder. Search for the<ShowScriptingDevelopmentTools>
property and set it totrue
. To reveal the Config.xml path, click on the Help / Show the Preferences Folder... menu entry in Renoise.
Once scripting is enabled, you'll have the following entries inside the Tools menu on the top bar.
- Scripting Terminal & Editor - This will open the debugging console used to test things and see your tool's output
- Reload All Tools - This will force a reload of all installed and running tools. It can be useful when adding new tools by hand or when changing them.
Lua
Tools in Renoise are written using the Lua programming language. Lua is a dynamic language with a friendly syntax and good performance. While Renoise itself is written in C++, it has an API layer that exposes all sorts of aspects of the tracker so that tools can implement new functionality without the dangers of crashing Renoise itself or having to worry about low level programming challenges.
note
Teaching you programming is out of the scope of this guide but you can check out the book Programming in Lua to get a complete overview of the language. That said, you will probably be able to pick up a lot of things just by reading through this guide and checking out the examples. If you are the kind of person who learns best by doing stuff, getting straight into tinkering might be right up your alley.
Language Server
Luckily Lua has a language server called LuaLS which - when paired with the definitions for the Renoise API - can help you write code by providing useful hints about functions and variables, autocompletion and code diagnostics. You can even annotate your own code to keep things more explicit and well documented. While you can absolutely write Lua and create tools without any of this, we highly recommend setting it up.
If you are using VSCode you will have to do three things
- Install the Lua extension by sumneko
- Download the Renoise API definitions from Github
- Configure VSCode so that LuaLS knows where to find the definition files. For this you will have to either put this into your workspace's settings in your project folder (as
.vscode/settings.json
), or the global User Settings JSON file. Make sure to fill the paths below according to where you've extracted the previously downloaded definitions.
{
"Lua.workspace.library": [ "PATH/TO/RENOISE_DEFINITION_FOLDER" ],
"Lua.runtime.plugin": "PATH/TO/RENOISE_DEFINITION_FOLDER/plugin.lua"
}
For configuring other editors, you can check out the official docs about installation
Anatomy of a tool
There are a few things that all tools must conform to for Renoise to successfully recognize and load them.
- A tool is either a folder or a zip file with the extension
.xrnx
- It has a lua script named
main.lua
which contains the tool's program - And a
manifest.xml
file that has version info, description and more
com.name_of_creator.name_of_tool.xrnx
├── manifest.xml
└── main.lua
note
You'll see that names of folders for most tools follow reverse domain notation, but don't worry, you don't have to actually own a domain to create and share your tools. Just use whatever nickname you want, just make sure it's not already taken by other devs to avoid confusion.
If needed, you can split your tool into multiple files and use the "require" function to load them inside your main script, but first you will be fine just using a single main script.
Some tools will also make use of other resources like icons, text files or audio samples, these should all be placed in the same folder (or any subfolders inside it).
Let's look at a basic tool to see what goes into these two files. You can find more elaborate examples by browsing the example tools.
Manifest
The manifest is a short XML file with the name manifest.xml
. It contains a few tag pairs like <Tag>...</Tag>
and some text between them, Renoise reads these and loads your tool based on the information it finds inside.
Here is an entire manifest file from a HelloWorld tool:
<?xml version="1.0" encoding="UTF-8"?>
<RenoiseScriptingTool doc_version="0">
<ApiVersion>6</ApiVersion>
<Id>com.renoise.HelloWorld</Id>
<Version>1.0</Version>
<Author>your name [your_email@address.com]</Author>
<Name>Hello World</Name>
<Category>Tool Development</Category>
<Description>
This tool is for printing "Hello World!" to the terminal when loaded.
</Description>
<Homepage>http://scripting.renoise.com</Homepage>
<Icon>icon.png</Icon>
<Platform>Windows, Mac, Linux</Platform>
</RenoiseScriptingTool>
Let's go through what each of these tags mean and what you should put inside them.
<?xml>
is the header for the XML file, this will stay the same across all tools<RenoiseScriptionTool doc_version="0">
tells Renoise that this XML describes a tool, this won't change either<ApiVersion>
is the version of the Renoise API your tool is using, this should be6
for the latest version<Id>
should match the folder name of your tool exactly without the.xrnx
at the end<Version>
is the version of your tool, whenever you release a new update you should increase this. It is best to follow standard semantic versioning conventions here.<Author>
contains your name and contact information, whenever your tool crashes, this information is going to be provided for the user alongside the crash message, you should use some contact where you can accept possbile bug reports or questions<Name>
the human readable name of your tool, it can be anything you want and you can change it anytime you feel like it<Category>
the category for your tool, which will be used to sort your tool on the official Tools page if you ever decide to submit it there<Description>
a short description of your tool which will be displayed inside the Tool Browser in Renoise and on the Tools page<Homepage>
your website's address if you have any, you could also put your forum topic or git repository here if you want<Icon>
the path to an optional icon to your tool<Platform>
a list of platforms your tool supports, as long as you're only using the Renoise API, your tools will be automatically cross-platform, but once you do something OS specific you should communicate that here
main.lua
Now that we have a manifest file, we can get to the exciting part of printing a message to the Renoise console. The contents of the main.lua
file will just have a single line for now.
print("Hello world!")
Done! Now that you wrote your first tool you probably want to install and test it.
Installing tools
You can install any tool by dragging its folder (or a zip file with the .xrnx
extension and the tool's contents) onto Renoise. You can also copy the folder manually into your Tools folder.
note
When creating a zip file you should only zip the contents of the folder, not the folder itself
After you've installed a tool, it will be activated automatically. When in doubt, click the Tools / Reload All Tools.
Debugging
Scripting Terminal
By default, all print
s from all tools are redirected to the Renoise Scripting Terminal. So the easiest way to debug a tool is to add debug prints to your tool.
Since the prints are redirected only when the terminal is open, it doesn't hurt to keep them in distributed tools, but the prints do create some overhead, so remove them if that's relevant - before releasing your tool.
In addition to the standard Lua print
, the Renoise APi offers two other print functions that can help with debugging:
oprint(some_object)
prints all methods/properties of API objects (or your own class objects), e.g.oprint(renoise.song())
.rprint(some_table)
prints tables recursively, which is handy for printing the layout of the renoise module/class, e.g.rprint(renoise)
Autoreloading Tools
When working with Renoise's Scripting Editor, saving a script will automatically reload the tool that belongs to the file. This way you can simply change your files and immediately see/test the changes. When changing any files that are part of the "Libraries" folder, all scripts will get reloaded.
When working with an external text editor, you can enable the following debug option somewhere in the tool's main.lua file:
_AUTO_RELOAD_DEBUG = function()
-- do tests like showing a dialog, prompts whatever, or simply do nothing
end
As soon as you save your script outside of Renoise, and then focus Renoise again (alt-tab to Renoise, for example), your script will instantly get reloaded and the notifier is called.
If you don't need a notifier to be called each time the script reloads, you can also simply set _AUTO_RELOAD_DEBUG to true:
_AUTO_RELOAD_DEBUG = true
Remote Debugging
If printing isn't enough, there is also remote debugging support built into Renoise via Remdebug.
Remdebug is a command line based remote debugger for Lua that comes with Renoise.
Prerequisites
To use the debugger you will need:
-
Renoise's "remdebug" module, which can be found in "Scripts/Libraries/remdebug" (no installation required - included in Renoise)
-
Lua support on your system's command-line with the Lua "socket" module See http://w3.impa.br/~diego/software/luasocket/
Overview
The debugger will be controlled via a command-line Lua interpreter, outside
of Renoise via the remdebug/controller.lua script. To start a local debug
session from within Renoise you can use the function debug.start()
:
-- Opens a debugger controller in a new terminal/cmd window and
-- attaches the debugger to this script. Immediately breaks execution.
debug.start()
You can add this anywhere in any script that runs in Renoise. This will work in a tool's main.lua main() body, just like a local function that you include. It also works in the TestPad.lua script that is used in Renoise's Scripting Editor.
Step By Step Guide
Let's debug the following small test script, paste into RENOISE_PREFERENCES/Scripts/TestPad.lua:
debug.start()
local function sum(a, b)
return a + b
end
local c = sum(1, 2)
print(c)
debug.stop()
-
Launch Renoise's scripting editor, open "TestPad.lua", and hit the "Execute" button to run the script.
If Lua is correctly installed on your system, and remdebug was found, Renoise should be frozen now, with a terminal window opened showing something like:
> "Lua Remote Debugger"
> "Paused at file RENOISE_PREFERENCES_FOLDER/Scripts/TestPad.lua line 5"
>
> 1 debug.start()
> 2
> 3 local function sum(a, b)
> 4 return a + b
> 5*** end
> 6
> 7 local c = sum(1, 2)
> 8 print(c)
> 9
> 10 debug.stop()
>
>>
-
To step through the code, you can use the "s" and "n" commands in the terminal. Let's do so by entering "s" (Return) until we've reached line 8. Anything you type into the debugger, which is not a debugger command, will get evaluated in your running script as an expression. So let's try this by entering:
c=99
-
Then step over the line by entering "n" (Return) to evaluate the "print(c)" on line 9 in the script. You should see a "99" dumped out. To watch the value again, enter for example a
print(c)
. You should again see a "99" dumped out.
You can also set break and watchpoints in the debugger. Type 'help' in the terminal to get more info about this. Those who are familiar with gdb on the command-line may be able to quickly get up to speed when using the most common shortcuts (c,b,q, and so on...).
Please note that although "debug.stop()" is not necessary (you can simply quit the controller at any time to exit), its recommended and will be more comfortable when running a session over and over again.
Remote and Lua Editor debugging
Renoise's remdebug is fully compatible with the original remdebug controller from the kepler project. This means you can, in theory, also use debugger GUIs that use the original remdebug, like Lua Eclipse or SciTE for Lua.
However, this is often a PITA to setup and configure, and might not be worth the trouble. Try at your own risk...
The debugger can also be used to remote debug scripts, scripts running on other computers. To do so, use remdebug.engine.start/stop instead of "debug". debug.start/stop is just a shortcut to remdebug.session.start/stop.
"remdebug.engine.start" will only attach the debugger to your script and break execution. You then have to run the debugger controller manually on another computer. To do so, launch the remdebug.controller.lua file manually in a terminal:
- First we start the debugger controller. To do so, open a command-line on your system and invoke the remdebug/controller.lua script. You should see something like:
lua RENOISE_RESOURCE_FOLDER/Scripts/Libraries/remdebug/controller.lua
"Lua Remote Debugger"
"Run the program you wish to debug with 'remdebug.engine.start()' now"
- Now you can connect to this controller by running a script with
remdebug.engine.start()
, configured to find the controller on another machine (or the same one.)
require "remdebug.engine"
-- default config is "localhost" on port 8171
remdebug.engine.configure { host = "some_host", port = 1234 }
remdebug.engine.start()
Distribution
Eventually you might want to share your work with others, in this case you will have to use the zipped format.
- First, we recommend sharing your tool on the Tools section of the forum to get some feedback and early testing done. If you create a topic there, you can also use it to notify users about updates or discuss potential improvements.
- Later on, you might want to submit your tool to the official Tool library so that users can find it easier and have automatic updates.
Community channels
If you have further questions or just want to discuss tools with fellow devs you can reach out over the following channels
- The official Renoise forum has a dedicated topic
- The Discord server has a room for scripting
- Chat over IRC
- Enter the Matrix at Renoise space on Matrix
- Post on Reddit
- Join the Facebook Group
Now that you can write, package and test your tool, you are ready to explore the depths of the API and start implementing your ideas.
Guides to the Renoise API
In this section you can learn about different aspects of the API. These guides will assume you've already read the chapters in our introduction and you are able to package and install tools.
Classes
The Lua language has by default no class
construct. But the Renoises lua API has a simple OO support inbuilt -> class "MyClass"
. All Renoise API objects use such classes and you can use them too in your tools.
See luabind docs for more technical info and below for some simple examples
Examples
-- abstract class
class 'Animal'
function Animal:__init(name)
self.name = name
self.can_fly = nil
end
function Animal:__tostring()
assert(self.can_fly ~= nil, "I don't know if I can fly or not")
return ("I am a %s (%s) and I %s fly"):format(self.name, type(self),
(self.can_fly and "can fly" or "can not fly"))
end
-- derived classes
-- MAMMAL
class 'Mammal' (Animal)
function Mammal:__init(str)
Animal.__init(self, str)
self.can_fly = false
end
-- BIRD
class 'Bird' (Animal)
function Bird:__init(str)
Animal.__init(self, str)
self.can_fly = true
end
-- FISH
class 'Fish' (Animal)
function Fish:__init(str)
Animal.__init(self, str)
self.can_fly = false
end
-- run
local farm = table.create()
farm:insert(Mammal("cow"))
farm:insert(Bird("sparrow"))
farm:insert(Fish("bass"))
print(("type(Mammal('cow')) -> %s"):format(type(Mammal("cow"))))
print(("type(Mammal) -> %s"):format(type(Mammal)))
for _,animal in pairs(farm) do
print(animal)
end
Something to keep in mind:
-
constructor
function MyClass:__init(args)
must be defined for each class, or the class can't be used to instantiate objects. -
class defs are always global, so even locally defined classes will be registered globally.
Class operators
You can overload most operators in Lua for your classes. You do this by simply declaring a member function with the same name as an operator (the name of the metamethods in Lua).
The operators you can overload are:
- __add
- __sub
- __mul
- __div
- __pow
- __lt
- __le
- __eq
- __call
- __unm
- __tostring
- __len
Note: __tostring
isn't really an operator, but it's the metamethod that is
called by the standard library's tostring() function.
Observables
The Renoise API makes extensive use of the Observer pattern. In short, different values can be wrapped in Observable objects on which you can register notifier functions that will get called whenever the underlying value changes. This is a great way to react to changes without having to deal with constantly comparing the value to a cached state for example.
When reading the API, you will find a lot of fields with _observable
at the end. Let's see how we can utilize this feature by attaching a notifier so that our tool can do something whenever the user loads a new song.
local loaded_new_song = function()
renoise.app():show_message("Loaded a new song!")
end
renoise.tool().app_new_document_observable:add_notifier(loaded_new_song)
Try loading a tool with this code inside and open new songs. Whenever you want to attach notifiers to fields on the song, you should do it inside this function to make sure a song is already loaded and that you reattach things when the song changes. To listen to changes on specific values, you usually have to add _observable
the name of the field and add a notifier to that.
Let's extend the previous snippet so that it also fires whenever you change the name of an already loaded song.
local new_name_was_set = function()
renoise.app():show_message("New name was set!\nName: " .. renoise.song().name)
end
local loaded_new_song = function()
renoise.app():show_message("Loaded a new song!")
renoise.song().name_observable:add_notifier(new_name_was_set)
end
renoise.tool().app_new_document_observable:add_notifier(loaded_new_song)
Now try changing the name of the current song inside the first field of the Song / Song Comments dialog to see your message pop up.
With this technique you can listen to all sorts of things which is very useful when you want your tool to change its behaviour or initialize itself each time the user selects a new instrument, track, sample and so on.
There are different Observables for each primitive type like ObservableBoolean
, ObservableNumber
or ObservableString
and ones that are for storing list of such values (ObservableBooleanList
etc.). You can check out the entire Observables API to see them all.
Files&Bits.lua
In order to access the raw bits and bytes of some data, e.g. to read or write binary (file) streams, you can use the Lua bit
library. It's built into the Renoise API. There's no need to require
it.
See bit documentation for more info and examples.
-- reading integer numbers or raw bytes from a file
local function read_word(file)
local bytes = file:read(2)
if (not bytes or #bytes < 2) then
return nil
else
return bit.bor(bytes:byte( 1),
bit.lshift(bytes:byte(2), 8))
end
end
local function read_dword(file)
local bytes = file:read(4)
if (not bytes or #bytes < 4) then
return nil
else
return bit.bor(bytes:byte(1),
bit.lshift(bytes:byte(2), 8),
bit.lshift(bytes:byte(3), 16),
bit.lshift(bytes:byte(4), 24))
end
end
-- and so on (adapt as needed to mess with endianess!) ...
local file = io.open("some_binary_file.bin", "rb")
local bytes = file:read(512)
if (not bytes or #bytes < 512) then
print("unexpected end of file")
else
for i = 1, #bytes do
print(bytes:byte(i))
end
end
print(read_word(file) or "unexpected end of file")
print(read_dword(file) or "unexpected end of file")
Sockets
The Renoise API allows you to create network sockets. This can be used to communicate with other devices via UDP and TCP, e.g. to send or receive OSC messages.
note
There's no support for encrypted connections. So using protocols like HTTPs is not easily possible with the socket API in Renoise.
HTTP / GET client
Creates a TCP socket and connect it to www.wurst.de, http, giving up the connection attempt after 2 seconds.
local connection_timeout = 2000
local client, socket_error = renoise.Socket.create_client(
"www.wurst.de", 80, renoise.Socket.PROTOCOL_TCP, connection_timeout)
if socket_error then
renoise.app():show_warning(socket_error)
return
end
-- request something
local succeeded, socket_error =
client:send("GET / HTTP/1.0\n\n")
if (socket_error) then
renoise.app():show_warning(socket_error)
return
end
-- loop until we get no more data from the server.
-- note: this is a silly example. we should check the HTTP
-- header here and stop after receiveing "Content-Length"
local receive_succeeded = false
local receive_content = ""
while (true) do
local receive_timeout = 500
local message, socket_error =
client:receive("*line", receive_timeout)
if (message) then
receive_content = receive_content .. message .. "\n"
else
if (socket_error == "timeout" or
socket_error == "disconnected")
then
-- could retry here on timeout. we just stop in this example...
receive_succeeded = true
break
else
renoise.app():show_warning(
"'socket reveive' failed with the error: " .. socket_error)
break
end
end
end
-- close the connection if it was not closed by the server
if (client and client.is_open) then
client:close()
end
-- show what we've got
if (receive_succeeded and #receive_content > 0) then
renoise.app():show_prompt(
"GET / HTTP/1.0 response",
receive_content,
{"OK"}
)
else
renoise.app():show_prompt(
"GET / HTTP/1.0 response",
"Socket receive timeout.",
{"OK"}
)
end
Echo UDP Server (using a table as notifier)
local server, socket_error = renoise.Socket.create_server(
"localhost", 1025, renoise.Socket.PROTOCOL_UDP)
if socket_error then
app:show_warning(
"Failed to start the echo server: " .. socket_error)
else
server:run {
---@param socket_error string
socket_error = function(socket_error)
renoise.app():show_warning(socket_error)
end,
---@param socket renoise.Socket.SocketClient
socket_accepted = function(socket)
print(("client %s:%d connected"):format(
socket.peer_address, socket.peer_port))
end,
---@param socket renoise.Socket.SocketClient
---@param message string
socket_message = function(socket, message)
print(("client %s:%d sent '%s'"):format(
socket.peer_address, socket.peer_port, message))
-- simply sent the message back
socket:send(message)
end
}
end
-- will run and echo as long as the script runs...
Echo TCP Server (using a class as notifier)
...and allowing any addresses to connect by not specifying an address:
class "EchoServer"
function EchoServer:__init(port)
-- create a server socket
local server, socket_error = renoise.Socket.create_server(port)
if socket_error then
app:show_warning(
"Failed to start the echo server: " .. socket_error)
else
-- start running
self.server = server
self.server:run(self)
end
end
---@param socket_error string
function EchoServer:socket_error(socket_error)
renoise.app():show_warning(socket_error)
end
---@param socket renoise.Socket.SocketClient
function EchoServer:socket_accepted(socket)
print(("client %s:%d connected"):format(
socket.peer_address, socket.peer_port))
end
---@param message string
---@param socket renoise.Socket.SocketClient
function EchoServer:socket_message(socket, message)
print(("client %s:%d sent '%s'"):format(
socket.peer_address, socket.peer_port, message))
-- simply sent the message back
socket:send(message)
end
-- create and run the echo server on port 1025
local echo_server = EchoServer(1025)
-- will run and echo as long as the script runs or the EchoServer
-- object is garbage collected...
Midi
The Renoise API allows you to access raw MIDI input and output devices from within your tool. You can use this to add bi-directional MIDI controller support, for example.
Midi input listener (function callback)
-- NOTE: the midi device will be closed when the local variable gets garbage
-- collected. Make it global or assign it to something which is held globally
-- to avoid that.
local midi_device = nil
local inputs = renoise.Midi.available_input_devices()
if not table.is_empty(inputs) then
-- use the first avilable device in this example
local device_name = inputs[1]
local function midi_callback(message)
assert(#message == 3)
assert(message[1] >= 0 and message[1] <= 0xff)
assert(message[2] >= 0 and message[2] <= 0xff)
assert(message[3] >= 0 and message[3] <= 0xff)
print(("%s: got MIDI %X %X %X"):format(device_name,
message[1], message[2], message[3]))
end
-- note: sysex callback would be a optional 2nd arg...
midi_device = renoise.Midi.create_input_device(
device_name, midi_callback)
-- stop dumping with 'midi_device:close()' ...
end
Midi input and sysex listener (class callbacks)
class "MidiDumper"
function MidiDumper:__init(device_name)
self.device_name = device_name
end
function MidiDumper:start()
self.device = renoise.Midi.create_input_device(
self.device_name,
{ self, MidiDumper.midi_callback },
{ MidiDumper.sysex_callback, self }
)
end
function MidiDumper:stop()
if self.device then
self.device:close()
self.device = nil
end
end
function MidiDumper:midi_callback(message)
print(("%s: MidiDumper got MIDI %X %X %X"):format(
self.device_name, message[1], message[2], message[3]))
end
function MidiDumper:sysex_callback(message)
print(("%s: MidiDumper got SYSEX with %d bytes"):format(
self.device_name, #message))
end
-- NOTE: the midi device will be closed when the local variable gets garbage
-- collected. Make it global or assign it to something which is held globally
-- to avoid that.
local midi_dumper = nil
local inputs = renoise.Midi.available_input_devices()
if not table.is_empty(inputs) then
-- use the first avilable device in this example
local device_name = inputs[1]
midi_dumper = MidiDumper(device_name)
-- will dump till midi_dumper:stop() is called or the MidiDumber object
-- is garbage collected...
midi_dumper:start()
end
Midi output
local outputs = renoise.Midi.available_output_devices()
if not table.is_empty(outputs) then
local device_name = outputs[1]
local midi_device = renoise.Midi.create_output_device(device_name)
-- note on
midi_device:send { 0x90, 0x10, 0x7F }
-- sysex (MMC start)
midi_device:send { 0xF0, 0x7F, 0x00, 0x06, 0x02, 0xF7 }
-- no longer need the device in this example...
midi_device:close()
end
Osc
The Renoise API allows you to create web sockets and provides tools to receive and send OSC data. This allows you to connect your tools to other OSC servers and send OSC data to existing clients.
In general, this can be used as an alternative to MIDI, e.g. to connect to OSC-enabled devices such as monome.
note
Using TCP instead of UDP as the socket protocol would likely also require manual SLIP encoding/decoding of OSC message data. This is omitted here, so the examples below will only work with UDP servers/clients.
Osc Server (receive Osc from one or more Clients)
-- create some shortcuts
local OscMessage = renoise.Osc.Message
local OscBundle = renoise.Osc.Bundle
-- open a socket connection to the server
local server, socket_error = renoise.Socket.create_server(
"localhost", 8008, renoise.Socket.PROTOCOL_UDP)
if (socket_error) then
renoise.app():show_warning(("Failed to start the " ..
"OSC server. Error: '%s'"):format(socket_error))
return
end
server:run {
socket_message = function(socket, data)
-- decode the data to Osc
local message_or_bundle, osc_error = renoise.Osc.from_binary_data(data)
-- show what we've got
if (message_or_bundle) then
if (type(message_or_bundle) == "Message") then
print(("Got OSC message: '%s'"):format(tostring(message_or_bundle)))
elseif (type(message_or_bundle) == "Bundle") then
print(("Got OSC bundle: '%s'"):format(tostring(message_or_bundle)))
else
-- never will get in here
end
else
print(("Got invalid OSC data, or data which is not " ..
"OSC data at all. Error: '%s'"):format(osc_error))
end
socket:send(("%s:%d: Thank you so much for the OSC message. " ..
"Here's one in return:"):format(socket.peer_address, socket.peer_port))
-- open a socket connection to the client
local client, socket_error = renoise.Socket.create_client(
socket.peer_address, socket.peer_port, renoise.Socket.PROTOCOL_UDP)
if (not socket_error) then
client:send(OscMessage("/flowers"))
end
end
}
-- shut off the server at any time with:
-- server:close()
Osc Client & Message Construction (send Osc to a Server)
-- create some shortcuts
local OscMessage = renoise.Osc.Message
local OscBundle = renoise.Osc.Bundle
-- open a socket connection to the server
local client, socket_error = renoise.Socket.create_client(
"localhost", 8008, renoise.Socket.PROTOCOL_UDP)
if (socket_error) then
renoise.app():show_warning(("Failed to start the " ..
"OSC client. Error: '%s'"):format(socket_error))
return
end
-- construct and send messages
client:send(
OscMessage("/someone/transport/start")
)
client:send(
OscMessage("/someone/transport/bpm", {
{tag="f", value=127.5}
})
)
-- construct and send bundles
client:send(
OscBundle(os.clock(), OscMessage("/someone/transport/start"))
)
local message1 = OscMessage("/some/message")
local message2 = OscMessage("/another/one", {
{tag="b", value="with some blob data"},
{tag="s", value="and a string"}
})
client:send(
OscBundle(os.clock(), {message1, message2})
)
Pattern Iterator
As a quick and efficient way to access the pattern data in phrases and the Renoise song, you can use the existing pattern iterators. Here are a few examples of how to use them.
See also API docs for renoise.PatternIterator.
Change notes in selection
changes all "C-4"s to "E-4" in the selection in the current pattern
local pattern_iter = renoise.song().pattern_iterator
local pattern_index = renoise.song().selected_pattern_index
for pos,line in pattern_iter:lines_in_pattern(pattern_index) do
for _,note_column in pairs(line.note_columns) do
if (note_column.is_selected and
note_column.note_string == "C-4") then
note_column.note_string = "E-4"
end
end
end
Generate a simple arp sequence
... repeating in the current pattern & track from line 0 to the pattern end
local pattern_iter = renoise.song().pattern_iterator
local pattern_index = renoise.song().selected_pattern_index
local track_index = renoise.song().selected_track_index
local instrument_index = renoise.song().selected_instrument_index
local EMPTY_VOLUME = renoise.PatternLine.EMPTY_VOLUME
local EMPTY_INSTRUMENT = renoise.PatternLine.EMPTY_INSTRUMENT
local arp_sequence = {
{note="C-4", instrument = instrument_index, volume = 0x20},
{note="E-4", instrument = instrument_index, volume = 0x40},
{note="G-4", instrument = instrument_index, volume = 0x80},
{note="OFF", instrument = EMPTY_INSTRUMENT, volume = EMPTY_VOLUME},
{note="G-4", instrument = instrument_index, volume = EMPTY_VOLUME},
{note="---", instrument = EMPTY_INSTRUMENT, volume = EMPTY_VOLUME},
{note="E-4", instrument = instrument_index, volume = 0x40},
{note="C-4", instrument = instrument_index, volume = 0x20},
}
for pos,line in pattern_iter:lines_in_pattern_track(pattern_index, track_index) do
if not table.is_empty(line.note_columns) then
local note_column = line:note_column(1)
note_column:clear()
local arp_index = math.mod(pos.line - 1, #arp_sequence) + 1
note_column.note_string = arp_sequence[arp_index].note
note_column.instrument_value = arp_sequence[arp_index].instrument
note_column.volume_value = arp_sequence[arp_index].volume
end
end
Hide empty volume, panning, and delay colums
for track_index, track in pairs(renoise.song().tracks) do
-- Set some bools
local found_volume = false
local found_panning = false
local found_delay = false
local found_sample_effects = false
-- Check whether or not this is a regular track
if
track.type ~= renoise.Track.TRACK_TYPE_MASTER and
track.type ~= renoise.Track.TRACK_TYPE_SEND
then
-- Iterate through the regular track
local iter = renoise.song().pattern_iterator:lines_in_track(track_index)
for _,line in iter do
-- Check whether or not the line is empty
if not line.is_empty then
-- Check each column on the line
for _,note_column in ipairs(line.note_columns) do
-- Check for volume
if note_column.volume_value ~= renoise.PatternLine.EMPTY_VOLUME then
found_volume = true
end
-- Check for panning
if note_column.panning_value ~= renoise.PatternLine.EMPTY_PANNING then
found_panning = true
end
-- Check for delay
if note_column.delay_value ~= renoise.PatternLine.EMPTY_DELAY then
found_delay = true
end
-- Check for sample effects
if note_column.effect_number_value ~= renoise.PatternLine.EMPTY_EFFECT_NUMBER then
found_sample_effects = true
end
if note_column.effect_amount_value ~= renoise.PatternLine.EMPTY_EFFECT_AMOUNT then
found_sample_effects = true
end
end
-- If we found something in all three vol, pan, and del
-- Then there's no point in continuing down the rest of the track
-- We break this loop and move on to the next track
if found_volume and found_panning and found_delay and found_sample_effects then
break
end
end
end
-- Set some properties
track.volume_column_visible = found_volume
track.panning_column_visible = found_panning
track.delay_column_visible = found_delay
track.sample_effects_column_visible = found_sample_effects
end
end
Track Automation
Access the selected parameters automation
... selected in the "Automation" tab in Renoise
local selected_track_parameter = renoise.song().selected_track_parameter
local selected_pattern_track = renoise.song().selected_pattern_track
-- is a parameter selected?.
if (selected_track_parameter) then
local selected_parameters_automation = selected_pattern_track:find_automation(
selected_track_parameter)
-- is there automation for the seelcted parameter?
if (not selected_parameters_automation) then
-- if not, create a new automation for the currently selected pattern/track
selected_parameters_automation = selected_pattern_track:create_automation(
selected_track_parameter)
end
---- do something with existing automation
-- iterate over all existing automation points
for _,point in pairs(selected_parameters_automation.points) do
print(("track automation: time=%s, value=%s"):format(
point.time, point.value))
end
-- clear all points
selected_parameters_automation.points = {}
-- insert a single new point at line 2
selected_parameters_automation:add_point_at(2, 0.5)
-- change its value when it already exists
selected_parameters_automation:add_point_at(2, 0.8)
-- remove it again (must exist here)
selected_parameters_automation:remove_point_at(2)
-- batch creation/insertion of points
local new_points = table.create()
for i=1,selected_parameters_automation.length do
new_points:insert {
time=i,
value=i/selected_parameters_automation.length
}
end
-- assign them (note that new_points must be sorted by time)
selected_parameters_automation.points = new_points
-- change the automations interpolation mode
selected_parameters_automation.playmode =
renoise.PatternTrackAutomation.PLAYMODE_CUBIC
end
Add menu entries for automation
-- shows up in the automation list on the left of the "Automation" tab
renoise.tool():add_menu_entry {
name = "Track Automation:Do Something With Automation",
invoke = function() do_something_with_current_automation() end,
active = function() return can_do_something_with_current_automation() end
}
-- shows up in the context menu of the automation !rulers!
renoise.tool():add_menu_entry {
name = "Track Automation List:Do Something With Automation",
invoke = function() do_something_with_current_automation() end,
active = function() return can_do_something_with_current_automation() end
}
function can_do_something_with_current_automation()
-- is a parameter selected and automation present?
return (renoise.song().selected_track_parameter ~= nil and
selected_pattern_track:find_automation(selected_track_parameter))
end
function do_something_with_current_automation()
-- do something with selected_parameters_automation
end
SampleBuffer
Modify the selected sample
local sample_buffer = renoise.song().selected_sample.sample_buffer
-- check if sample data is preset at all first
if (sample_buffer.has_sample_data) then
-- before touching any sample, let renoise create undo data, when necessary
sample_buffer:prepare_sample_data_changes()
-- modify sample data in the selection (defaults to the whole sample)
for channel = 1, sample_buffer.number_of_channels do
for frame = sample_buffer.selection_start, sample_buffer.selection_end do
local value = sample_buffer:sample_data(channel, frame)
value = -value -- do something with the value
sample_buffer:set_sample_data(channel, frame, value)
end
end
-- let renoise update sample overviews and caches. apply bit depth
-- quantization. create undo/redo data if needed...
sample_buffer:finalize_sample_data_changes()
else
renoise.app():show_warning("No sample preset...")
end
Generate a new sample
local selected_sample = renoise.song().selected_sample
local sample_buffer = selected_sample.sample_buffer
-- create new or overwrite sample data for our sound:
local sample_rate = 44100
local num_channels = 1
local bit_depth = 32
local num_frames = sample_rate / 2
local allocation_succeeded = sample_buffer:create_sample_data(
sample_rate, bit_depth, num_channels, num_frames)
-- check for allocation failures
if (not allocation_succeeded) then
renoise.app():show_error("Out of memory. Failed to allocate sample data")
return
end
-- let renoise know we are about to change the sample buffer
sample_buffer:prepare_sample_data_changes()
-- fill in the sample data with an amazing zapp sound
for channel = 1,num_channels do
for frame = 1,num_frames do
local sample_value = math.sin(num_frames / frame)
sample_buffer:set_sample_data(channel, frame, sample_value)
end
end
-- let renoise update sample overviews and caches. apply bit depth
-- quantization. finalize data for undo/redo, when needed...
sample_buffer:finalize_sample_data_changes()
-- setup a pingpong loop for our new sample
selected_sample.loop_mode = renoise.Sample.LOOP_MODE_PING_PONG
selected_sample.loop_start = 1
selected_sample.loop_end = num_frames
Keybindings
Tools can add custom key bindings somewhere in Renoise's existing set of bindings, which will be activated and mapped by the user just as any other key binding in Renoise.
Keybindings can be global (applied everywhere in the GUI) or can be local to a specific part of the GUI, like the Pattern Editor.
Please note: there's no way to define default keyboard shortcuts for your entries. Users manually have to bind them in the keyboard prefs pane. As soon as they do, they'll get saved just like any other key binding in Renoise.
To do so, we are using the tool's add_keybinding function.
Example
renoise.tool():add_keybinding {
name = "Global:Tools:Example Script Shortcut",
invoke = function(repeated)
if (not repeated) then -- we ignore soft repeated keys here
renoise.app():show_prompt(
"Congrats!",
"You've pressed a magic keyboard combo "..
"which was defined by a scripting tool.",
{"OK?"}
)
end
end
}
Scopes
The scope, name and category of the key binding use the form:
$scope:$topic_name:$binding_name
:
-
scope
is where the shortcut will be applied, just like those in the categories list for the keyboard assignment preference pane. Your key binding will only fire, when the scope is currently focused, except it's the global scope one. Using an unavailable scope will not fire an error, instead it will render the binding useless. It will be listed and mappable, but never be invoked. -
topic_name
is useful when grouping entries in the key assignment pane. Use "tool" if you can't come up with something meaningful. -
binding_name
is the name of the binding.
Currently available scopes are:
See API Docs for KeybindingEntry.
Separating entries
To divide entries into groups (separate entries with a line), prepend one or more dashes to the name, like
"--- Main Menu:Tools:My Tool Group Starts Here"
Menu Entries
You can add new menu entries into any existing context menus or the global menu in Renoise.
To do so, we are using the tool's add_menu_entry function.
Example
renoise.tool():add_menu_entry {
name = "Main Menu:Tools:My Tool:Show Message...",
invoke = function()
renoise.app():show_prompt(
"Congrats!",
"You've pressed then 'Show Message...' menu entry from the tools menu, "..
"which was defined by a scripting tool.",
{"OK?"}
)
end
}
Avilable Menus
You can place your entries in any context menu or any window menu in Renoise. To do so, use one of the specified categories in its name.
See API Docs for ToolMenuEntry for more info.
Separating Entries
To divide entries into groups (separate entries with a line), prepend one or more dashes to the name, like
"--- Main Menu:Tools:My Tool Group Starts Here"
Entry Sub Groups
To move entries into a menu sub groups, use a common path for them, like
"Main Menu:Tools:My Tool Group:First Entry"
"Main Menu:Tools:My Tool Group:Second Entry"
"Main Menu:Tools:My Tool Group:Third Entry"
Views
Currently there are two ways to to create custom views in Tools:
-- Shows a modal dialog with a title, custom content and custom button labels:
renoise.app():show_custom_prompt(
title, content_view, {button_labels} [, key_handler_func, key_handler_options])
-> [pressed button]
See API Docs for show_custom_prompt for more info.
-- Shows a non modal dialog, a floating tool window, with custom content:
renoise.app():show_custom_dialog(
title, content_view [, key_handler_func, key_handler_options])
-> [dialog object]
See API Docs for show_custom_dialog for more info.
The key_handler_func
in the custom dialog is optional. When defined, it should point to a function
with the signature noted below.
See API Docs for KeyHandler for more info.
Creating Custom Views
Widgets are created with the renoise.ViewBuilder
class in the renoise API.
See API Docs for ViewBuilder for more info.
Hello World
-- We start by instantiating a view builder object. We'll use it to create views.
local vb = renoise.ViewBuilder()
-- Now we are going to use a "column" view. A column can do three things:
-- 1. showing a background (if you don't want your views on the plain dialogs
-- back)
-- 2. "stack" other views (its child views) either vertically, or horizontally
-- vertically = vb:column{}
-- horizontally = vb:row{}
-- 3. align child views via "margins" -> borders for nested views
local dialog_title = "Hello World"
local dialog_buttons = { "OK" }
-- fetch some consts to let the dialog look like Renoises default views...
local DEFAULT_MARGIN = renoise.ViewBuilder.DEFAULT_CONTROL_MARGIN
-- start with a 'column' view, to stack other views vertically:
local dialog_content = vb:column {
-- set a border of DEFAULT_MARGIN around our main content
margin = DEFAULT_MARGIN,
-- and create another column to align our text in a different background
vb:column {
-- background style that is usually used for "groups"
style = "group",
-- add again some "borders" to make it more pretty
margin = DEFAULT_MARGIN,
-- now add the first text into the inner column
vb:text {
text = "from the Renoise Scripting API\n"..
"in a vb:column with a background"
},
}
}
-- show the custom content in a prompt
renoise.app():show_custom_prompt(
dialog_title, dialog_content, dialog_buttons)
Dynamic Content
GUIs usually are dynamic. They interact with the user. Do do so, you'll need to memorize references to some of your widgets. Here's an example on how this works in the Renoise View API.
local vb = renoise.ViewBuilder()
-- we've used above an inlined style to create view. This is very elegant
-- when creating only small & simple GUIs, but can also be confusing when the
-- view hierarchy gets more complex.
-- you actually can also build views step by step, instead of passing a table
-- with properties, set the properties of the views manually:
local DEFAULT_DIALOG_MARGIN = renoise.ViewBuilder.DEFAULT_DIALOG_MARGIN
-- this:
local my_column_view = vb:column{}
my_column_view.margin = DEFAULT_DIALOG_MARGIN
my_column_view.style = "group"
local my_text_view = vb:text{}
my_text_view.text = "My text"
my_column_view:add_child(my_text_view)
-- is exactly the same like this:
local my_column_view = vb:column{
margin = DEFAULT_DIALOG_MARGIN,
style = "group",
vb:text{
text = "My text"
}
}
-- In practice you should use a combination of the above two notations, but
-- its recommended to setup & prepare components in separate steps while
-- still using the inlined / nested notation:
local my_first_column_view = vb:column {
-- some content
}
local my_second_column_view = vb:column {
-- some more content
}
-- Then do the final layout:
local my_final_layout = vb:row {
my_first_column_view,
my_second_column_view
}
-- The inlined notation has a problem though: You can not memorize your views
-- in local variables, in case you want to access them later (for example to
-- hide/how them, change the text or whatever else). This is what viewbuilder
-- "id"s are for.
-- Lets build up a simple view that dynamically reacts on a button hit:
local DEFAULT_DIALOG_MARGIN = renoise.ViewBuilder.DEFAULT_DIALOG_MARGIN
local DEFAULT_CONTROL_SPACING = renoise.ViewBuilder.DEFAULT_CONTROL_SPACING
local dialog_title = "vb IDs"
local dialog_buttons = {"OK"};
local dialog_content = vb:column {
margin = DEFAULT_DIALOG_MARGIN,
spacing = DEFAULT_CONTROL_SPACING,
vb:text {
id = "my_text", -- we're giving the view a unique id here
text = "Do what you see"
},
vb:button {
text = "Hit Me!",
tooltip = "Hit this button to change the above text.",
notifier = function()
-- here we resolve the id and access the above constructed view
local my_text_view = vb.views.my_text
my_text_view.text = "Button was hit."
end
}
}
-- We are doing two things here:
-- First we do create a vb:text as usual, but this time we also give it an
-- id "my_text_view". This id can then at any time be used to resolve this
-- view. So we can use the inlined notation without having to create lots of
-- local view refs
-- There's now also a first control present: a button. Controls may have
-- notifiers.
-- The buttons notifier is simply a function without arguments, which is
-- called as soon as you hit the button. Tf you use other views like a
-- value box, the notifiers will pass a value along your function...
-- Please note that ids are unique !per viewbuilder object!, so you can
-- create several viewbuilders (one for each component) to access multiple
-- sets of ids.
renoise.app():show_custom_prompt(
dialog_title, dialog_content, dialog_buttons)
More Examples
See com.renoise.ExampleToolGUI.xrnx for more examples. This tool be read as it's own little guide and provides a lot more in-depth examples.
The example tools can also be downloaded as part of a XRNX Starter pack Bundle from the releases page.
Preferences
Tools can have preferences just like Renoise. To use them we first need to create a renoise.Document object which holds the options that we want to store and restore.
Documents in Renoise have all of their fields defined as an Observable type. This comes extra handy when you want to create some settings dialog that needs to be able change the behaviour of your tool and remember its state, while you also want to access these settings across your tool. By using the built-in Document API you get a lot of this functionality for free.
Let's see an example of setting up an options
object that can be used for the above things.
Our goal here is to have three kinds of settings managed by the Document class and a dialog that can be used to change them. The tool will be able to randomize the song by changing BPM and creating a random number of tracks.
We will also define a menu entry for opening our tool settings dialog, you can read more about menu entries in the Menus guide, graphical dialogs are further discussed in Views.
-- We are creating a new renoise Document by supplying a table of values.
-- It has three fields, which will all get wrapped in an Observable
-- * a boolean for whether or not the tools should randomize the BPM
-- * another boolean for randomizing tracks
-- * an integer representing how many tracks we want to have at maximum
local options = renoise.Document.create("RandomizerToolPreferences") {
randomize_bpm = true,
randomize_tracks = false,
max_tracks = 16
}
-- once we have our options, we have to assign it to our tool
renoise.tool().preferences = options
-- we define a randomizer function
-- when called, it will set a random BPM
-- and add or remove tracks randomly
function randomize_song()
local song = renoise.song()
-- make sure you use .value when accessing the underlying value inside Observables
if options.randomize_bpm.value then
-- we are setting the BPM to a value between 60 and 180
song.transport.bpm = 60 + math.random() * 120
end
if options.randomize_tracks.value then
-- let's figure out how many tracks we want based on the max_tracks option
local target_count = 1 + math.floor(math.random() * options.max_tracks.value)
-- and cache the amount of regular tracks the song has
local current_count = song.sequencer_track_count
-- we will either insert new tracks if there aren't enough
if current_count < target_count then
for i = 1, target_count - current_count do
song:insert_track_at(current_count)
end
else
-- or remove them if there is too much
for i = 1, current_count - target_count do
song:delete_track_at(song.sequencer_track_count)
end
end
end
end
-- let's define a function for a custom dialog
-- it will contain checkboxes and a slider for our options
function show_options()
local vb = renoise.ViewBuilder()
local dialog_content = vb:vertical_aligner {
margin = renoise.ViewBuilder.DEFAULT_CONTROL_MARGIN,
vb:row {
vb:text {
text = "Randomize BPM"
},
-- here we are binding our observable value to this checkbox
vb:checkbox {
bind = options.randomize_bpm
}
},
vb:row {
vb:text {
text = "Randomize Tracks"
},
-- the same thing for the boolean for tracks
vb:checkbox {
bind = options.randomize_tracks
}
},
vb:row {
vb:text {
text = "Max Tracks"
},
-- for the maximum tracks we create a value box
-- and restrict it to a range of [1..16]
vb:valuebox {
min = 1,
max = 16,
bind = options.max_tracks
}
},
-- add a button that will execute the randomization based on our options
vb:row {
vb:button {
text = "Randomize",
pressed = randomize_song
}
}
}
renoise.app():show_custom_dialog(
"Randomizer Options", dialog_content
)
end
-- finally we add a menu entry to open our options dialog
renoise.tool():add_menu_entry {
name = "Main Menu:Tools:Randomizer Options",
invoke = show_options
}
As you can see all we had to do was to assign our observables to the bind
field on the checkboxes and valuebox, Renoise will take care of updating our settings when the view changes and vice versa.
Of course you could also use observables this way without them being included in your settings, but this is the most common usage pattern for them.
preferences.xml
When you assign the preferences, Renoise will take care of saving and loading your settings. Your tool will have a preferences.xml
file created in its folder with the values from your options table. As long as you are using simple types, you don't have to worry about (de)serializing values.
Try restarting Renoise to see that the values you've set in your dialog will persist over different sessions.
Complex Documents
For more complex applications (or if you just prefer doing things the Object-Oriented way) you can also inherit from renoise.Document.DocumentNode
and register properties in the constructor.
You could change the above Document creation to something like this:
---@class RandomizerToolPreferences : renoise.Document.DocumentNode
---@field randomize_bpm renoise.Document.ObservableBoolean
---@field randomize_tracks renoise.Document.ObservableBoolean
---@field max_tracks renoise.Document.ObservableNumber
class "RandomizerToolPreferences" (renoise.Document.DocumentNode)
function RandomizerToolPreferences:__init()
renoise.Document.DocumentNode.__init(self)
-- register an observable properties which will make up our Document
self:add_property("randomize_bpm", true)
self:add_property("randomize_tracks", false)
self:add_property("max_tracks", 16)
end
---@type RandomizerToolPreferences
local options = RandomizerToolPreferences()
renoise.tool().preferences = options
This allows you to create more complex documents. Have a look at the complete Document API for more info and details about what else you can load/store this way.
note
This time we also included type annotations (like ---@class RandomizerToolPreferences
). These can help you with development but they aren't strictly necessary. You can read more about how to use them at the LuaLS website.
Renoise Lua API Overview
The API files in this documentation folder will list all available Lua functions and classes that can be accessed from scripts in Renoise. If you are familiar with Renoise, the names of the classes, functions and properties should be self explanitory.
A note about the general API design:
-
Whatever you do with the API, you should never be able to fatally crash Renoise. If you manage to do this, then please file a bug report in our forums so we can fix it. All errors, as stupid they might be, should always result in a clean error message from Lua.
-
The Renoise Lua API also allows global File IO and external program execution (via
os.execute()
) which can obviously be hazardous. Please be careful with these, as you would with programming in general...
Some notes about the documentation, and a couple of tips:
-
All classes, functions in the API, are nested in the namespace (Lua table)
renoise
. E.g: to get the application object, you will have to typerenoise.app()
-
The API is object-oriented, and thus split into classes. The references will first note the class name (e.g.
renoise.Application
), then list its Constants, Properties, Functions and Operators. All properties and functions are always listed with their full path to make it clear where they belong and how to access them. -
Nearly all functions are actually methods, so you have to invoke them via the colon operator
:
E.g.renoise.app():show_status("Status Message")
If you're new to Lua, this takes a while to get used to. Don't worry, it'll make sense sooner or later. ;) -
Properties are syntactic sugar for get/set functions.
song().comments
will invoke a function which returns comments. But not all properties have setters, and thus can only be used as read-only getters. Those are marked as**READ-ONLY**
. -
All exposed objects are read-only (you can not add new fields, properties). In contrast, the classes are not. This means you can extend the API classes with your own helper functions, if needed, but can not add new properties to objects. Objects, like for example the result of
song()
, are read-only to make it easier to catch typos.song().transport.bmp = 80
will fire an error, because there is no such property 'bmp.' You probably meantsong().transport.bpm = 80
here. If you need to store data somewhere, do it in your own tables, objects instead of using the Renoise API objects. -
some_property, _observable means, that there is also an observer object available for the property. An observable object allows you to attach notifiers (global functions or methods) that will be called as soon as a value has changed. Please see Renoise.Document.API for more info about observables and related classes.
A small example using bpm:
renoise.song().transport.bpm_observable:add_notifier(function()
print("bpm changed")
end)
-- will print "bpm changed", but only if the bpm was not 120 before
renoise.song().transport.bpm = 120
The above notifier is called when anything changes the bpm, including your script, other scripts, or anything else in Renoise (you've automated the BPM in the song, entered a new BPM value in Renoise's GUI, whatever...)
Lists like renoise.song().tracks[]
can also have notifiers. But these will only fire when the list layout has changed: an element was added, removed or elements in the list changed their order. They will not fire when the list values changed. Attach notifiers to the list elements to get such notifications.
- Can't remember what the name of function XYZ was? In the scripting terminal you can list all methods/properties of API objects (or your own class objects) via the global function
oprint(some_object)
- e.g.oprint(renoise.song())
. To dump the renoise module/class layout, userprint(renoise)
.
renoise
Holds all renoise related API functions and classes.
Constants
API_VERSION : number
Currently 6.1. Any changes in the API which are not backwards compatible, will increase the internal API's major version number (e.g. from 1.4 -> 2.0). All other backwards compatible changes, like new functionality, new functions and classes which do not break existing scripts, will increase only the minor version number (e.g. 1.0 -> 1.1).
RENOISE_VERSION : string
Renoise Version "Major.Minor.Revision[AlphaBetaRcVersion][Demo]"
Functions
ViewBuilder()
Construct a new viewbuilder instance you can use to create views.
app()
Global access to the Renoise Application.
song()
Global access to the Renoise Song.
NB: The song instance changes when a new song is loaded or created in Renoise, so tools can not memorize the song instance globally once, but must instead react on the application's
new_document_observable
observable.
tool()
Global access to the Renoise Scripting Tool (your XRNX tool).
This is only valid when getting called from a tool and not when e.g. using the scripting terminal and editor in Renoise.
renoise.Application
The Renoise application.
- Properties
- Functions
- show_message(self, message :
string
) - show_error(self, message :
string
) - show_warning(self, message :
string
) - show_status(self, message :
string
) - show_prompt(self, title :
string
, message :string
, button_labels :string
[]?
) - show_custom_prompt(self, title :
string
, content_view :renoise.Views.View
, button_labels :string
[], key_handler :KeyHandler``?
, key_handler_options :KeyHandlerOptions``?
) - show_custom_dialog(self, title :
DialogTitle
, content_view :renoise.Views.View
, key_handler :KeyHandler``?
, key_handler_options :KeyHandlerOptions``?
) - prompt_for_path(self, title :
DialogTitle
) - prompt_for_filename_to_read(self, file_extensions :
string
[], title :DialogTitle
) - prompt_for_multiple_filenames_to_read(self, file_extensions :
string
[], title :DialogTitle
) - prompt_for_filename_to_write(self, file_extension :
string
, title :DialogTitle
) - open_url(self, url :
string
) - open_path(self, file_path :
string
) - install_tool(self, file_path :
string
) - uninstall_tool(self, file_path :
string
) - new_song(self)
- new_song_no_template(self)
- load_song(self, filename :
string
) - load_track_device_chain(self, filename :
string
) - load_track_device_preset(self, filename :
string
) - load_instrument(self, filename :
string
) - load_instrument_multi_sample(self, filename :
string
) - load_instrument_device_chain(self, filename :
string
) - load_instrument_device_preset(self, filename :
string
) - load_instrument_modulation_set(self, filename :
string
) - load_instrument_phrase(self, filename :
string
) - load_instrument_sample(self, filename :
string
) - load_theme(self, filename :
string
) - save_song(self)
- save_song_as(self, filename :
string
) - save_track_device_chain(self, filename :
string
) - save_instrument(self, filename :
string
) - save_instrument_multi_sample(self, filename :
string
) - save_instrument_device_chain(self, filename :
string
) - save_instrument_modulation_set(self, filename :
string
) - save_instrument_phrase(self, filename :
string
) - save_instrument_sample(self, filename :
string
) - save_theme(self, filename :
string
)
- show_message(self, message :
- Local Structs
- KeyEvent
- KeyHandlerOptions
Properties
log_filename : string
READ-ONLY Access to the application's full log filename and path. Will already be opened for writing, but you nevertheless should be able to read from it.
current_song : renoise.Song
READ-ONLY Get the apps main document, the song. The global "renoise.song()" function is, in fact, a shortcut to this property.
recently_loaded_song_files : string
[]
READ-ONLY List of recently loaded song files.
recently_saved_song_files : string
[]
READ-ONLY List of recently saved song files.
installed_tools : table<string
, string
>
READ-ONLY Returns information about all currently installed tools.
key_modifier_states : table<string
, string
>
READ-ONLY Access keyboard modifier states.
window : renoise.ApplicationWindow
READ-ONLY Access to the application's window.
active_clipboard_index : 1
| 2
| 3
| 4
Range: (1 - 4) Get or set globally used clipboard "slots" in the application.
Functions
show_message(self, message : string
)
Shows an info message dialog to the user.
show_error(self, message : string
)
Shows an error dialog to the user.
show_warning(self, message : string
)
Shows a warning dialog to the user.
show_status(self, message : string
)
Shows a message in Renoise's status bar to the user.
show_prompt(self, title : string
, message : string
, button_labels : string
[]?
)
->
label : string
Opens a modal dialog with a title, text and custom button labels. Returns the pressed button label or an empty string when canceled.
show_custom_prompt(self, title : string
, content_view : renoise.Views.View
, button_labels : string
[], key_handler : KeyHandler
?
, key_handler_options : KeyHandlerOptions
?
)
->
label : string
Opens a modal dialog with a title, custom content and custom button labels.
See: renoise.ViewBuilder for more info about custom views.
show_custom_dialog(self, title : DialogTitle
, content_view : renoise.Views.View
, key_handler : KeyHandler
?
, key_handler_options : KeyHandlerOptions
?
)
Shows a non modal dialog (a floating tool window) with custom content.
When no key_handler is provided, the Escape key is used to close the dialog. See: renoise.ViewBuilder for more info about custom views.
prompt_for_path(self, title : DialogTitle
)
->
path : string
Opens a modal dialog to query an existing directory from the user.
prompt_for_filename_to_read(self, file_extensions : string
[], title : DialogTitle
)
->
path : string
Opens a modal dialog to query a filename and path to read from a file.
prompt_for_multiple_filenames_to_read(self, file_extensions : string
[], title : DialogTitle
)
->
paths : string
[]
Same as 'prompt_for_filename_to_read' but allows the user to select more than one file.
prompt_for_filename_to_write(self, file_extension : string
, title : DialogTitle
)
->
path : string
Open a modal dialog to get a filename and path for writing. When an existing file is selected, the dialog will ask whether or not to overwrite it, so you don't have to take care of this on your own.
open_url(self, url : string
)
Opens the default internet browser with the given URL. The URL can also be a file that browsers can open (like xml, html files...).
open_path(self, file_path : string
)
Opens the default file browser (explorer, finder...) with the given path.
install_tool(self, file_path : string
)
Install order update a tool. Any errors are shown to the user during installation. Installing an already existing tool will upgrade the tool without confirmation. Upgraded tools will automatically be re-enabled, if necessary.
uninstall_tool(self, file_path : string
)
Uninstall an existing tool. Any errors are shown to the user during uninstallation.
new_song(self)
Create a new song document (will ask the user to save changes if needed). The song is not created immediately, but soon after the call was made and the user did not aborted the operation. In order to continue execution with the new song, attach a notifier to 'renoise.app().new_document_observable' See: renoise.ScriptingTool for more info.
new_song_no_template(self)
Create a new song document, avoiding template XRNS songs (when present) to be loaded. The song is not created immediately, but soon after the call was made and the user did not aborted the operation. In order to continue execution with the new song, attach a notifier to 'renoise.app().new_document_observable' See: renoise.ScriptingTool for more info.
load_song(self, filename : string
)
Load a new song document from the given filename (will ask to save changes if needed, any errors are shown to the user). Just like new_song(), the song is not loaded immediately, but soon after the call was made. See 'renoise.app():new_song()' for details.
load_track_device_chain(self, filename : string
)
->
success : boolean
Load a track device chains into the currently selected track. Any errors during the export are shown to the user.
load_track_device_preset(self, filename : string
)
->
success : boolean
Load a track device devices into the currently selected track. When no device is selected a new device will be created. Any errors during the export are shown to the user.
load_instrument(self, filename : string
)
->
success : boolean
Load an instrument into the currently selected instrument. Any errors during the export are shown to the user.
load_instrument_multi_sample(self, filename : string
)
->
success : boolean
Load an instrument multi sample into the currently selected instrument. Any errors during the export are shown to the user.
load_instrument_device_chain(self, filename : string
)
->
success : boolean
Load an instrument device chain into the currently selected instrument's device chain. When no device chain is selected, a new one will be created. Any errors during the export are shown to the user.
load_instrument_device_preset(self, filename : string
)
->
success : boolean
Load an instrument device into the currently selected instrument's device chain's device. When no device is selected, a new one will be created. Any errors during the export are shown to the user.
load_instrument_modulation_set(self, filename : string
)
->
success : boolean
Load an instrument modulation chain into the currently selected instrument's modulation chain. When no device is selected, a new one will be created. Any errors during the export are shown to the user.
load_instrument_phrase(self, filename : string
)
->
success : boolean
Load an instrument phrase into the currently selected instrument's phrases. When no phrase is selected, a new one will be created. Any errors during the export are shown to the user.
load_instrument_sample(self, filename : string
)
->
success : boolean
Load an instrument sample into the currently selected instrument's sample lists. When no sample is selected, a new one will be created. Any errors during the export are shown to the user.
load_theme(self, filename : string
)
->
success : boolean
Load a new theme file and apply it. Any errors during the export are shown to the user.
save_song(self)
Quicksave or save the current song under a new name. Any errors during the export are shown to the user.
save_song_as(self, filename : string
)
Save the current song under a new name. Any errors during the export are shown to the user.
save_track_device_chain(self, filename : string
)
->
success : boolean
Save a currently selected track device chain to a file with the given name. When no device chain is selected an error is raised. returns success.
save_instrument(self, filename : string
)
->
success : boolean
Save a currently selected instrument to a file with the given name. When no instruemnt is selected an error is raised. returns success.
save_instrument_multi_sample(self, filename : string
)
->
success : boolean
Save a currently selected instrument multi sample file to a file with the given name. When no instrument is selected an error is raised. returns success.
save_instrument_device_chain(self, filename : string
)
->
success : boolean
Save a currently selected instrument's device chain to a file with the given name. When no chain is selected an error is raised. returns success.
save_instrument_modulation_set(self, filename : string
)
->
success : boolean
Save a currently selected instrument's modulation set to a file with the given name. When no modulation is selected an error is raised. returns success.
save_instrument_phrase(self, filename : string
)
->
success : boolean
Save a currently selected instrument's phrase to a file with the given name. When no phrase is selected an error is raised. returns success.
save_instrument_sample(self, filename : string
)
->
success : boolean
Save a currently selected instrument's sample to a file with the given name. When no sample is selected an error is raised. returns success.
save_theme(self, filename : string
)
->
success : boolean
Save a current theme to a file with the given name. returns success.
Local Structs
KeyEvent
Properties
name : string
name of the key, like 'esc' or 'a'
modifiers : ModifierStates
the held down modifiers as a string
character : string
?
possible character representation of the key
note : integer
?
virtual keyboard piano key value (starting from 0)
state : "pressed"
| "released"
only present if
send_key_release
was set to true
repeated : boolean
?
only present if
send_key_repeat
was set to true
KeyHandlerOptions
Properties
send_key_repeat : boolean
?
Default: true
send_key_release : boolean
?
Default: false
Local Aliases
DialogTitle
The title that shows up at the title-bar of the dialog.
KeyHandler
(dialog : renoise.Dialog
, key_event : KeyEvent
) ->
KeyEvent
?
Optional keyhandler to process key events on a custom dialog.
When returning the passed key from the key-handler function, the key will be passed back to Renoise's key event chain, in order to allow processing global Renoise key-bindings from your dialog. This will not work for modal dialogs. This also only applies to global shortcuts in Renoise, because your dialog will steal the focus from all other Renoise views such as the Pattern Editor, etc.
ModifierStates
The modifier keys will be provided as a string.
Possible keys are dependent on the platform
- Windows : "shift", "alt", "control", "winkey"
- Linux : "shift", "alt", "control", "meta"
- Mac : "shift", "option", "control", "command" If multiple modifiers are held down, the string will be formatted as
"+ " Their order will correspond to the following precedence shift + alt/option + control + winkey/meta/command
If no modifier is pressed, this will be an empty string
renoise.ApplicationWindow
Application window and general UI properties of the Renoise app.
- Constants
- Properties
- fullscreen :
boolean
- is_maximized :
boolean
- is_minimized :
boolean
- lock_keyboard_focus :
boolean
- sample_record_dialog_is_visible :
boolean
- disk_browser_is_visible :
boolean
- disk_browser_is_visible_observable :
renoise.Document.Observable
- instrument_box_is_visible :
boolean
- instrument_box_is_visible_observable :
renoise.Document.Observable
- instrument_editor_is_detached :
boolean
- instrument_editor_is_detached_observable :
renoise.Document.Observable
- mixer_view_is_detached :
boolean
- mixer_view_is_detached_observable :
renoise.Document.Observable
- upper_frame_is_visible :
boolean
- upper_frame_is_visible_observable :
renoise.Document.Observable
- active_upper_frame :
renoise.ApplicationWindow.UpperFrame
- active_upper_frame_observable :
renoise.Document.Observable
- active_middle_frame :
renoise.ApplicationWindow.MiddleFrame
- active_middle_frame_observable :
renoise.Document.Observable
- lower_frame_is_visible :
boolean
- lower_frame_is_visible_observable :
renoise.Document.Observable
- active_lower_frame :
renoise.ApplicationWindow.LowerFrame
- active_lower_frame_observable :
renoise.Document.Observable
- pattern_matrix_is_visible :
boolean
- pattern_matrix_is_visible_observable :
renoise.Document.Observable
- pattern_advanced_edit_is_visible :
boolean
- pattern_advanced_edit_is_visible_observable :
renoise.Document.Observable
- mixer_view_post_fx :
boolean
- mixer_view_post_fx_observable :
renoise.Document.Observable
- mixer_fader_type :
renoise.ApplicationWindow.MixerFader
- mixer_fader_type_observable :
renoise.Document.Observable
- fullscreen :
- Functions
Constants
UpperFrame
{ UPPER_FRAME_TRACK_SCOPES: integer = 1, UPPER_FRAME_MASTER_SPECTRUM: integer = 2, }
MiddleFrame
{ MIDDLE_FRAME_PATTERN_EDITOR: integer = 1, MIDDLE_FRAME_MIXER: integer = 2, MIDDLE_FRAME_INSTRUMENT_PHRASE_EDITOR: integer = 3, MIDDLE_FRAME_INSTRUMENT_SAMPLE_KEYZONES: integer = 4, MIDDLE_FRAME_INSTRUMENT_SAMPLE_EDITOR: integer = 5, MIDDLE_FRAME_INSTRUMENT_SAMPLE_MODULATION: integer = 6, MIDDLE_FRAME_INSTRUMENT_SAMPLE_EFFECTS: integer = 7, MIDDLE_FRAME_INSTRUMENT_PLUGIN_EDITOR: integer = 8, MIDDLE_FRAME_INSTRUMENT_MIDI_EDITOR: integer = 9, }
LowerFrame
{ LOWER_FRAME_TRACK_DSPS: integer = 1, LOWER_FRAME_TRACK_AUTOMATION: integer = 2, }
MixerFader
{ MIXER_FADER_TYPE_24DB: integer = 1, MIXER_FADER_TYPE_48DB: integer = 2, MIXER_FADER_TYPE_96DB: integer = 3, MIXER_FADER_TYPE_LINEAR: integer = 4, }
Properties
fullscreen : boolean
Get/set if the application is running fullscreen.
is_maximized : boolean
READ-ONLY. Window status flag.
is_minimized : boolean
READ-ONLY. Window status flag.
lock_keyboard_focus : boolean
When true, the middle frame views (like the pattern editor) will stay focused unless alt or middle mouse is clicked.
sample_record_dialog_is_visible : boolean
Dialog for recording new samples, floating above the main window.
disk_browser_is_visible : boolean
Diskbrowser Panel.
disk_browser_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
instrument_box_is_visible : boolean
InstrumentBox
instrument_box_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
instrument_editor_is_detached : boolean
Instrument Editor detaching.
instrument_editor_is_detached_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mixer_view_is_detached : boolean
Mixer View detaching.
mixer_view_is_detached_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
upper_frame_is_visible : boolean
Frame with the scopes/master spectrum...
upper_frame_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_upper_frame : renoise.ApplicationWindow.UpperFrame
active_upper_frame_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_middle_frame : renoise.ApplicationWindow.MiddleFrame
Frame with the pattern editor, mixer...
active_middle_frame_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
lower_frame_is_visible : boolean
Frame with the DSP chain view, automation, etc.
lower_frame_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_lower_frame : renoise.ApplicationWindow.LowerFrame
active_lower_frame_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
pattern_matrix_is_visible : boolean
Pattern matrix, visible in pattern editor and mixer only...
pattern_matrix_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
pattern_advanced_edit_is_visible : boolean
Pattern advanced edit, visible in pattern editor only...
pattern_advanced_edit_is_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mixer_view_post_fx : boolean
Mixer views Pre/Post volume setting.
mixer_view_post_fx_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mixer_fader_type : renoise.ApplicationWindow.MixerFader
Mixer fader type setting.
mixer_fader_type_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
maximize(self)
Expand the window over the entire screen, without hiding menu bars, docks and so on.
minimize(self)
Minimize the window to the dock or taskbar, depending on the OS.
restore(self)
"un-maximize" or "un-minimize" the window, or just bring it to front.
select_preset(self, preset_index : integer
)
Select/activate one of the global view presets, to memorize/restore the user interface layout.
renoise.AudioDevice
Audio DSP device in tracks or sample device chains.
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- active_preset :
integer
- active_preset_observable :
renoise.Document.Observable
- active_preset_data :
string
- presets :
string
[] - is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - external_editor_available :
boolean
- external_editor_visible :
boolean
- device_path :
string
- name :
- Functions
Properties
name : string
READ-ONLY
short_name : string
READ-ONLY
display_name : string
Configurable device display name. When empty
name
is displayed.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
!active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in DSP chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_preset : integer
0 when none is active or available
active_preset_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_preset_data : string
raw serialized data in XML format of the active preset
presets : string
[]
READ-ONLY preset names
is_active_parameter : renoise.DeviceParameter
READ-ONLY
parameters : renoise.DeviceParameter
[]
READ-ONLY
external_editor_available : boolean
READ-ONLY Returns whether or not the device provides its own custom GUI (only available for some plugin devices)
external_editor_visible : boolean
true to show the editor, false to close it
device_path : string
READ-ONLY Returns a string that uniquely identifies the device, from
available_devices
. The string can be passed into:renoise.song().tracks[]:insert_device_at()
Functions
preset(self, index : integer
)
->
preset_name : string
Access to a single preset name by index. Use properties 'presets' to iterate over all presets and to query the presets count. comment
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.DeviceParameter
A single parameter within an audio DSP effect (renoise.AudioDevice)
- Constants
- Properties
- name :
string
- polarity :
renoise.DeviceParameter.Polarity
- value_min :
number
- value_max :
number
- value_quantum :
number
- value_default :
number
- time_quantum :
number
- is_automatable :
boolean
- is_automated :
boolean
- is_automated_observable :
renoise.Document.Observable
- is_midi_mapped :
boolean
- is_midi_mapped_observable :
renoise.Document.Observable
- show_in_mixer :
boolean
- show_in_mixer_observable :
renoise.Document.Observable
- value :
number
- value_observable :
renoise.Document.Observable
- value_string :
string
- value_string_observable :
renoise.Document.Observable
- name :
- Functions
Constants
Polarity
{ POLARITY_UNIPOLAR: integer = 1, POLARITY_BIPOLAR: integer = 2, }
Properties
name : string
READ-ONLY
polarity : renoise.DeviceParameter.Polarity
READ-ONLY
value_min : number
READ-ONLY
value_max : number
READ-ONLY
value_quantum : number
READ-ONLY
value_default : number
READ-ONLY
time_quantum : number
READ-ONLY
is_automatable : boolean
READ-ONLY
is_automated : boolean
READ-ONLY Is automated. Not valid for parameters of instrument devices.
is_automated_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_midi_mapped : boolean
READ-ONLY Parameter has a custom MIDI mapping in the current song.
is_midi_mapped_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
show_in_mixer : boolean
Show in mixer. Not valid for parameters of instrument devices.
show_in_mixer_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
value : number
value in Range: (value_min - value_max)
value_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
value_string : string
value_string_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
record_value(self, value : number
)
Set a new value and write automation when the MIDI mapping "record to automation" option is set. Only works for parameters of track devices, not for instrument devices.
renoise.Dialog
A custom dialog created via the scripting API. Dialogs can be created via
renoise.app():show_custom_dialog
.See
create custom views
on top of the renoise.ViewBuilder docs on how to create views for the dialog.
Properties
visible : boolean
READ-ONLY Check if a dialog is alive and visible.
Functions
show(self)
Bring an already visible dialog to front and make it the key window.
close(self)
Close a visible dialog.
renoise.Document
renoise.Document classes are wrappers for Renoise's internal document classes.
Please note: the Lua wrappers are not really "the Lua way" of solving and expressing things. e.g: there's no support for mixed types in lists, tuples at the moment.
Documents can be serialized from/to XML, just like Renoise's internal document and are observable.
An empty document (node) object can be created via
renoise.Document.create("MyDoc"){}
Such document objects can then be extended with the document's
add_property
function. Existing properties can also be removed again with theremove_property
function.examples:
-- Creates an empty document, using "MyDoc" as the model name (a type name) local my_document = renoise.Document.create("MyDoc"){ } -- adds a number to the document with the initial value 1 my_document:add_property("value1", 1) -- adds a string my_document:add_property("value2", "bla") -- create another document and adds it local node = renoise.Document.create("MySubDoc"){ } node:add_property("another_value", 1) -- add another already existing node my_document:add_property("nested_node", node) -- removes a previously added node my_document:remove_property(node) -- access properties local value1 = my_document.value1 value1 = my_document:property("value1")
As an alternative to
renoise.Document.create
, you can also inherit from renoise.Document.DocumentNode in order to create your own document classes. This is especially recommended when dealing with more complex docs, because you can also use additional methods to deal with your properties, the data.examples:
class "MyDocument" (renoise.Document.DocumentNode) function MyDocument:__init() -- important! call super first renoise.Document.DocumentNode.__init(self) -- add properties to construct the document model self:add_property("age", 1) self:add_property("name", renoise.Document.ObservableString("value")) -- other doc renoise.Document.DocumentNode object self:add_property("sub_node", MySubNode()) -- list of renoise.Document.DocumentNode objects self:add_property("doc_list", renoise.Document.DocumentList()) -- or the create() way: self:add_properties { something = "else" } end
Instantiating such custom document objects can then be done by simply calling the constructor:
my_document = MyDocument() -- do something with my_document, load/save, add/remove more properties
Functions
create(model_name : string
)
->
(properties : ObservableProperties
) ->
renoise.Document.DocumentNode
Create an empty DocumentNode or a DocumentNode that is modeled after the passed key value table. "model_name" will be used to identify the documents type when loading/saving. It also allows you to instantiate new document objects (see renoise.Document.instantiate).
examples:
my_document = renoise.Document.create("MyDoc") { age = 1, name = "bla", -- implicitly specify a property type is_valid = renoise.Document.ObservableBoolean(false), -- or explicitly age_list = {1, 2, 3}, another_list = renoise.Document.ObservableNumberList(), sub_node = { sub_value1 = 2, sub_value2 = "bla2" } }
This will create a document node which is !modeled! after the the passed table. The table is not used internally by the document after construction, and will only be referenced to construct new instances. Also note that you need to assign values for all passed table properties in order to automatically determine it's type, or specify the types explicitly -> renoise.Document.ObservableXXX().
The passed name ("MyDoc" in the example above) is used to identify the document when loading/saving it (loading a XML file which was saved with a different model will fail) and to generally specify the "type".
Additionally, once "create" is called, you can use the specified model name to create new instances.
examples:
-- create a new instance of "MyDoc" my_other_document = renoise.Document.instantiate("MyDoc")
instantiate(model_name : string
)
->
renoise.Document.DocumentNode
Create a new instance of the given document model. Given
model_name
must have been registered withrenoise.Document.create
before.
Local Aliases
DocumentMember
renoise.Document.DocumentList
| renoise.Document.DocumentNode
| renoise.Document.Observable
| renoise.Document.ObservableList
Track changes to document properties or general states by attaching listener functions to it.
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
NotifierFunction
fun()
NotifierMemberContext
ObservableProperties
table<string
, ObservableTypes
| renoise.Document.DocumentList
| renoise.Document.DocumentNode
>
ObservableTypes
boolean
| string
| number
| boolean
[] | number
[] | string
[]
renoise.Document.DocumentList
A document list is a document sub component which may contain other document nodes in an observable list.
- Functions
- size(self)
- property(self, index :
integer
) - find(self, start_pos :
integer
, value :renoise.Document.DocumentNode
) - insert(self, pos :
integer
, value :renoise.Document.DocumentNode
) - remove(self, pos :
integer
) - swap(self, pos1 :
integer
, pos2 :integer
) - has_notifier(self, notifier :
ListNotifierFunction
) - add_notifier(self, notifier :
ListNotifierFunction
) - remove_notifier(self, notifier :
ListNotifierFunction
|ListNotifierMemberContext
)
- Local Aliases
Functions
size(self)
->
integer
Returns the number of entries of the list.
property(self, index : integer
)
->
renoise.Document.DocumentNode
?
List item access by index. returns nil for non existing items.
find(self, start_pos : integer
, value : renoise.Document.DocumentNode
)
Find a value in the list by comparing the list values with the passed value. The first successful match is returned. When no match is found, nil is returned.
insert(self, pos : integer
, value : renoise.Document.DocumentNode
)
->
renoise.Document.DocumentNode
Insert a new item to the end of the list when no position is specified, or at the specified position. Returns the newly created and inserted Observable.
remove(self, pos : integer
)
Removes an item (or the last one if no index is specified) from the list.
swap(self, pos1 : integer
, pos2 : integer
)
Swaps the positions of two items without adding/removing the items.
With a series of swaps you can move the item from/to any position.
has_notifier(self, notifier : ListNotifierFunction
)
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : ListNotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the document lists layout changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
remove_notifier(self, notifier : ListNotifierFunction
| ListNotifierMemberContext
)
Unregister a previously registered list notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
Local Aliases
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
renoise.Document.DocumentNode
A document node is a sub component in a document which contains other documents or observables.
- Functions
- __init(self)
- has_property(self, property_name :
string
) - property(self, property_name :
string
) - add_property(self, name :
string
, value :renoise.Document.DocumentNode
) - add_properties(self, properties :
ObservableProperties
) - remove_property(self, value :
DocumentMember
) - save_as(self, file_name :
string
) - load_from(self, file_name :
string
) - to_string(self)
- from_string(self, string :
string
)
- Local Aliases
Functions
__init(self)
Base constructor, only necessary to be called in your custom class constructor, when inheriting from renoise.Document.DocumentNode.
has_property(self, property_name : string
)
->
boolean
Check if the given property exists.
property(self, property_name : string
)
Access a property by name. Returns the property, or nil when there is no such property.
add_property(self, name : string
, value : renoise.Document.DocumentNode
)
->
renoise.Document.DocumentNode
Add a new property. Name must be unique: overwriting already existing properties with the same name is not allowed and will fire an error. If you want to replace a property, remove it first, then add it again.
add_properties(self, properties : ObservableProperties
)
Add a batch of properties in one go, similar to renoise.Document.create.
remove_property(self, value : DocumentMember
)
Remove a previously added property. Property must exist.
In order to remove a value by it's key, use
my_document:remove_property(my_document["some_member"])
save_as(self, file_name : string
)
->
success : boolean
, error : string
?
Save the whole document tree to an XML file. Overwrites all contents of the file when it already exists.
load_from(self, file_name : string
)
->
success : boolean
, error : string
?
Load the document tree from an XML file. This will NOT create new properties, except for list items, but will only assign existing property values in the document node with existing property values from the XML. This means: nodes that only exist in the XML will silently be ignored. Nodes that only exist in the document, will not be altered in any way. The loaded document's type must match the document type that saved the XML data. A document's type is specified in the renoise.Document.create() function as "model_name". For classes which inherit from renoise.Document.DocumentNode it's the class name.
to_string(self)
->
string
Serialize the whole document tree to a XML string.
from_string(self, string : string
)
->
success : boolean
, error : string
?
Parse document tree from the given string data. See renoise.Document.DocumentNode:load_from for details about how properties are parsed and errors are handled.
Local Aliases
DocumentMember
renoise.Document.DocumentList
| renoise.Document.DocumentNode
| renoise.Document.Observable
| renoise.Document.ObservableList
Track changes to document properties or general states by attaching listener functions to it.
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
NotifierFunction
fun()
NotifierMemberContext
ObservableProperties
table<string
, ObservableTypes
| renoise.Document.DocumentList
| renoise.Document.DocumentNode
>
ObservableTypes
boolean
| string
| number
| boolean
[] | number
[] | string
[]
renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
has_notifier(self, notifier : NotifierFunction
)
->
boolean
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : NotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable's value changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
examples:
renoise.song().transport.bpm_observable:add_notifier(function() print("BPM changed") end) local my_context = { bpm_changes = 0, something_else = "bla" } renoise.song().transport.bpm_observable:add_notifier({ my_context, function(context) context.bpm_changes = context.bpm_changes + 1; print(("#BPM changes: %s"):format(context.bpm_changes)); end })
remove_notifier(self, notifier : NotifierFunction
| NotifierMemberContext
)
Unregister a previously registered notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
Local Aliases
NotifierFunction
fun()
NotifierMemberContext
renoise.Document.ObservableBang
Observable without a value which sends out notifications when "banging" it.
Functions
has_notifier(self, notifier : NotifierFunction
)
->
boolean
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : NotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable's value changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
examples:
renoise.song().transport.bpm_observable:add_notifier(function() print("BPM changed") end) local my_context = { bpm_changes = 0, something_else = "bla" } renoise.song().transport.bpm_observable:add_notifier({ my_context, function(context) context.bpm_changes = context.bpm_changes + 1; print(("#BPM changes: %s"):format(context.bpm_changes)); end })
remove_notifier(self, notifier : NotifierFunction
| NotifierMemberContext
)
Unregister a previously registered notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
bang(self)
fire a notification, calling all registered notifiers.
Local Aliases
NotifierFunction
fun()
NotifierMemberContext
renoise.Document.ObservableBoolean
Properties
value : boolean
Read/write access to the value of an observable.
Functions
has_notifier(self, notifier : NotifierFunction
)
->
boolean
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : NotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable's value changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
examples:
renoise.song().transport.bpm_observable:add_notifier(function() print("BPM changed") end) local my_context = { bpm_changes = 0, something_else = "bla" } renoise.song().transport.bpm_observable:add_notifier({ my_context, function(context) context.bpm_changes = context.bpm_changes + 1; print(("#BPM changes: %s"):format(context.bpm_changes)); end })
remove_notifier(self, notifier : NotifierFunction
| NotifierMemberContext
)
Unregister a previously registered notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
Local Aliases
NotifierFunction
fun()
NotifierMemberContext
renoise.Document.ObservableBooleanList
A observable list of boolean values.
- Functions
- to_string(self)
- from_string(self, string :
any
) - size(self)
- has_notifier(self, notifier :
ListNotifierFunction
) - add_notifier(self, notifier :
ListNotifierFunction
) - remove_notifier(self, notifier :
ListNotifierFunction
|ListNotifierMemberContext
) - property(self, index :
integer
) - find(self, start_pos :
integer
, value :boolean
) - insert(self, pos :
integer
, value :boolean
) - remove(self, pos :
integer
) - swap(self, pos1 :
integer
, pos2 :integer
)
- Local Aliases
Functions
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
size(self)
->
integer
Returns the number of entries of the list.
has_notifier(self, notifier : ListNotifierFunction
)
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : ListNotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable lists layout changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
remove_notifier(self, notifier : ListNotifierFunction
| ListNotifierMemberContext
)
Unregister a previously registered list notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
property(self, index : integer
)
->
renoise.Document.ObservableBoolean
?
List item access by index. returns nil for non existing items.
find(self, start_pos : integer
, value : boolean
)
Find a value in the list by comparing the list values with the passed value. The first successful match is returned. When no match is found, nil is returned.
insert(self, pos : integer
, value : boolean
)
->
renoise.Document.ObservableBoolean
Insert a new item to the end of the list when no position is specified, or at the specified position. Returns the newly created and inserted Observable.
remove(self, pos : integer
)
Removes an item (or the last one if no index is specified) from the list.
swap(self, pos1 : integer
, pos2 : integer
)
Swaps the positions of two items without adding/removing the items.
With a series of swaps you can move the item from/to any position.
Local Aliases
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
size(self)
->
integer
Returns the number of entries of the list.
has_notifier(self, notifier : ListNotifierFunction
)
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : ListNotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable lists layout changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
remove_notifier(self, notifier : ListNotifierFunction
| ListNotifierMemberContext
)
Unregister a previously registered list notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
Local Aliases
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
renoise.Document.ObservableNumber
Properties
value : number
Read/write access to the value of an Observable.
Functions
has_notifier(self, notifier : NotifierFunction
)
->
boolean
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : NotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable's value changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
examples:
renoise.song().transport.bpm_observable:add_notifier(function() print("BPM changed") end) local my_context = { bpm_changes = 0, something_else = "bla" } renoise.song().transport.bpm_observable:add_notifier({ my_context, function(context) context.bpm_changes = context.bpm_changes + 1; print(("#BPM changes: %s"):format(context.bpm_changes)); end })
remove_notifier(self, notifier : NotifierFunction
| NotifierMemberContext
)
Unregister a previously registered notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
Local Aliases
NotifierFunction
fun()
NotifierMemberContext
renoise.Document.ObservableNumberList
A observable list of number values.
- Functions
- to_string(self)
- from_string(self, string :
any
) - size(self)
- has_notifier(self, notifier :
ListNotifierFunction
) - add_notifier(self, notifier :
ListNotifierFunction
) - remove_notifier(self, notifier :
ListNotifierFunction
|ListNotifierMemberContext
) - property(self, index :
integer
) - find(self, start_pos :
integer
, value :number
) - insert(self, pos :
integer
, value :number
) - remove(self, pos :
integer
) - swap(self, pos1 :
integer
, pos2 :integer
)
- Local Aliases
Functions
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
size(self)
->
integer
Returns the number of entries of the list.
has_notifier(self, notifier : ListNotifierFunction
)
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : ListNotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable lists layout changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
remove_notifier(self, notifier : ListNotifierFunction
| ListNotifierMemberContext
)
Unregister a previously registered list notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
property(self, index : integer
)
->
renoise.Document.ObservableNumber
?
List item access by index. returns nil for non existing items.
find(self, start_pos : integer
, value : number
)
Find a value in the list by comparing the list values with the passed value. The first successful match is returned. When no match is found, nil is returned.
insert(self, pos : integer
, value : number
)
->
renoise.Document.ObservableNumber
Insert a new item to the end of the list when no position is specified, or at the specified position. Returns the newly created and inserted Observable.
remove(self, pos : integer
)
Removes an item (or the last one if no index is specified) from the list.
swap(self, pos1 : integer
, pos2 : integer
)
Swaps the positions of two items without adding/removing the items. With a series of swaps you can move the item from/to any position.
Local Aliases
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
renoise.Document.ObservableString
Properties
value : string
Read/write access to the value of an Observable.
Functions
has_notifier(self, notifier : NotifierFunction
)
->
boolean
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : NotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable's value changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
examples:
renoise.song().transport.bpm_observable:add_notifier(function() print("BPM changed") end) local my_context = { bpm_changes = 0, something_else = "bla" } renoise.song().transport.bpm_observable:add_notifier({ my_context, function(context) context.bpm_changes = context.bpm_changes + 1; print(("#BPM changes: %s"):format(context.bpm_changes)); end })
remove_notifier(self, notifier : NotifierFunction
| NotifierMemberContext
)
Unregister a previously registered notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
Local Aliases
NotifierFunction
fun()
NotifierMemberContext
renoise.Document.ObservableStringList
A observable list of number values.
- Functions
- to_string(self)
- from_string(self, string :
any
) - size(self)
- has_notifier(self, notifier :
ListNotifierFunction
) - add_notifier(self, notifier :
ListNotifierFunction
) - remove_notifier(self, notifier :
ListNotifierFunction
|ListNotifierMemberContext
) - property(self, index :
integer
) - find(self, start_pos :
integer
, value :number
) - insert(self, pos :
integer
, value :number
) - remove(self, pos :
integer
) - swap(self, pos1 :
integer
, pos2 :integer
)
- Local Aliases
Functions
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
size(self)
->
integer
Returns the number of entries of the list.
has_notifier(self, notifier : ListNotifierFunction
)
Checks if the given function, method was already registered as notifier.
add_notifier(self, notifier : ListNotifierFunction
)
Register a function or method as a notifier, which will be called as soon as the observable lists layout changed. The passed notifier can either be a function or a table with a function and some context (an "object") -> method.
remove_notifier(self, notifier : ListNotifierFunction
| ListNotifierMemberContext
)
Unregister a previously registered list notifier. When only passing an object, all notifier functions that match the given object will be removed. This will not fire errors when no methods for the given object are attached. Trying to unregister a function or method which wasn't registered, will resolve into an error.
property(self, index : integer
)
->
renoise.Document.ObservableString
?
List item access by index. returns nil for non existing items.
find(self, start_pos : integer
, value : number
)
Find a value in the list by comparing the list values with the passed value. The first successful match is returned. When no match is found, nil is returned.
insert(self, pos : integer
, value : number
)
->
renoise.Document.ObservableString
Insert a new item to the end of the list when no position is specified, or at the specified position. Returns the newly created and inserted Observable.
remove(self, pos : integer
)
Removes an item (or the last one if no index is specified) from the list.
swap(self, pos1 : integer
, pos2 : integer
)
Swaps the positions of two items without adding/removing the items. With a series of swaps you can move the item from/to any position.
Local Aliases
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
renoise.Document.Serializable
Functions
to_string(self)
->
string
Serialize an object to a string.
from_string(self, string : any
)
->
string
Assign the object's value from a string - when possible. Errors are silently ignored.
renoise.EffectColumn
A single effect column in a pattern line.
Access effect column properties either by values (numbers) or by strings. The string representation uses exactly the same notation as you see them in Renoise's pattern or phrase editor.
Properties
is_empty : boolean
READ-ONLY True, when all effect column properties are empty.
is_selected : boolean
READ-ONLY True, when this column is selected in the pattern or phrase editor.
number_value : integer
0-65535 in the form 0x0000xxyy where xx=effect char 1 and yy=effect char 2
number_string : string
Range: ('00' - 'ZZ')
amount_value : integer
Range: (0 - 255)
amount_string : string
Range: ('00' - 'FF')
Functions
clear(self)
Clear the effect column.
copy_from(self, other : renoise.EffectColumn
)
Copy the column's content from another column.
renoise.GroupTrack
Group track component of a Renoise song.
- Properties
- type :
renoise.Track.TrackType
- name :
string
- name_observable :
renoise.Document.Observable
- color :
RGBColor
- color_observable :
renoise.Document.Observable
- color_blend :
integer
- color_blend_observable :
renoise.Document.Observable
- mute_state :
renoise.Track.MuteState
- mute_state_observable :
renoise.Document.Observable
- solo_state :
boolean
- solo_state_observable :
renoise.Document.Observable
- prefx_volume :
renoise.DeviceParameter
- prefx_panning :
renoise.DeviceParameter
- prefx_width :
renoise.DeviceParameter
- postfx_volume :
renoise.DeviceParameter
- postfx_panning :
renoise.DeviceParameter
- collapsed :
boolean
- collapsed_observable :
renoise.Document.Observable
- group_parent :
renoise.GroupTrack
- available_output_routings :
string
[] - output_routing :
string
- output_routing_observable :
renoise.Document.Observable
- output_delay :
number
- output_delay_observable :
renoise.Document.Observable
- max_effect_columns :
integer
- min_effect_columns :
integer
- max_note_columns :
integer
- min_note_columns :
integer
- visible_effect_columns :
integer
- visible_effect_columns_observable :
renoise.Document.Observable
- visible_note_columns :
integer
- visible_note_columns_observable :
renoise.Document.Observable
- volume_column_visible :
boolean
- volume_column_visible_observable :
renoise.Document.Observable
- panning_column_visible :
boolean
- panning_column_visible_observable :
renoise.Document.Observable
- delay_column_visible :
boolean
- delay_column_visible_observable :
renoise.Document.Observable
- sample_effects_column_visible :
boolean
- sample_effects_column_visible_observable :
renoise.Document.Observable
- available_devices :
string
[] - available_device_infos :
AudioDeviceInfo
[] - devices :
renoise.AudioDevice
[] - devices_observable :
renoise.Document.ObservableList
- members :
renoise.Track
[] - group_collapsed :
boolean
- type :
- Functions
- insert_device_at(self, device_path :
string
, device_index :integer
) - delete_device_at(self, device_index :
any
) - swap_devices_at(self, device_index1 :
integer
, device_index2 :integer
) - device(self, device_index :
integer
) - mute(self)
- unmute(self)
- solo(self)
- column_is_muted(self, column_index :
integer
) - column_is_muted_observable(self, column_index :
integer
) - set_column_is_muted(self, column_index :
integer
, muted :boolean
) - column_name(self, column_index :
integer
) - column_name_observable(self, column_index :
integer
) - set_column_name(self, column_index :
integer
, name :string
) - swap_note_columns_at(self, column_index1 :
integer
, column_index2 :integer
) - swap_effect_columns_at(self, column_index1 :
integer
, column_index2 :integer
)
- insert_device_at(self, device_path :
- Local Structs
- AudioDeviceInfo
Properties
type : renoise.Track.TrackType
READ-ONLY
name : string
Name, as visible in track headers
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
color : RGBColor
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
color_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
color_blend : integer
Range: (0 - 100) Color blend in percent
color_blend_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mute_state : renoise.Track.MuteState
Mute and solo states. Not available for the master track.
mute_state_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
solo_state : boolean
solo_state_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
prefx_volume : renoise.DeviceParameter
READ-ONLY
prefx_panning : renoise.DeviceParameter
READ-ONLY
prefx_width : renoise.DeviceParameter
READ-ONLY
postfx_volume : renoise.DeviceParameter
READ-ONLY
postfx_panning : renoise.DeviceParameter
READ-ONLY
collapsed : boolean
Collapsed/expanded visual appearance.
collapsed_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
group_parent : renoise.GroupTrack
READ-ONLY
available_output_routings : string
[]
READ-ONLY
output_routing : string
One of
available_output_routings
output_routing_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
output_delay : number
Range: (-100.0-100.0) in ms
output_delay_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
max_effect_columns : integer
READ-ONLY 8 OR 0 depending on the track type
min_effect_columns : integer
READ-ONLY 1 OR 0 depending on the track type
max_note_columns : integer
READ-ONLY 12 OR 0 depending on the track type
min_note_columns : integer
READ-ONLY 1 OR 0 depending on the track type
visible_effect_columns : integer
1-8 OR 0-8, depending on the track type
visible_effect_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
visible_note_columns : integer
0 OR 1-12, depending on the track type
visible_note_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume_column_visible : boolean
volume_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
panning_column_visible : boolean
panning_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
delay_column_visible : boolean
delay_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sample_effects_column_visible : boolean
sample_effects_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
available_devices : string
[]
READ-ONLY FX devices this track can handle.
available_device_infos : AudioDeviceInfo
[]
READ-ONLY Array of tables containing information about the devices.
devices : renoise.AudioDevice
[]
READ-ONLY List of audio DSP FX.
devices_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
members : renoise.Track
[]
READ-ONLY All member tracks of this group, including subgroups and their tracks.
group_collapsed : boolean
Collapsed/expanded visual appearance of whole group.
Functions
insert_device_at(self, device_path : string
, device_index : integer
)
Insert a new device at the given position.
device_path
must be one ofrenoise.Track.available_devices
.
delete_device_at(self, device_index : any
)
Delete an existing device in a track. The mixer device at index 1 can not be deleted from any track.
swap_devices_at(self, device_index1 : integer
, device_index2 : integer
)
Swap the positions of two devices in the device chain. The mixer device at index 1 can not be swapped or moved.
device(self, device_index : integer
)
Access to a single device by index. Use property
devices
to iterate over all devices and to query the device count.
mute(self)
Uses default mute state from the prefs. Not for the master track.
unmute(self)
solo(self)
column_is_muted(self, column_index : integer
)
->
boolean
Note column mutes. Only valid within (1-track.max_note_columns)
column_is_muted_observable(self, column_index : integer
)
set_column_is_muted(self, column_index : integer
, muted : boolean
)
column_name(self, column_index : integer
)
->
string
Note column names. Only valid within (1-track.max_note_columns)
column_name_observable(self, column_index : integer
)
set_column_name(self, column_index : integer
, name : string
)
swap_note_columns_at(self, column_index1 : integer
, column_index2 : integer
)
Swap the positions of two note or effect columns within a track.
swap_effect_columns_at(self, column_index1 : integer
, column_index2 : integer
)
Local Structs
AudioDeviceInfo
Audio device info
Properties
path : string
The device's path used by
renoise.Track:insert_device_at
name : string
The device's name
short_name : string
The device's name as displayed in shortened lists
favorite_name : string
The device's name as displayed in favorites
is_favorite : boolean
true if the device is a favorite
is_bridged : boolean
true if the device is a bridged plugin
Local Aliases
RGBColor
{ 1 : integer
, 2 : integer
, 3 : integer
}
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
renoise.Instrument
- Constants
- Properties
- active_tab :
renoise.Instrument.Tab
- active_tab_observable :
renoise.Document.Observable
- name :
string
- name_observable :
renoise.Document.Observable
- comments :
string
[] - comments_observable :
renoise.Document.Observable
- comments_assignment_observable :
renoise.Document.Observable
- show_comments_after_loading :
boolean
- show_comments_after_loading_observable :
renoise.Document.Observable
- macros_visible :
boolean
- macros_visible_observable :
renoise.Document.Observable
- macros :
renoise.InstrumentMacro
[] - pitchbend_macro :
renoise.InstrumentMacro
- modulation_wheel_macro :
renoise.InstrumentMacro
- channel_pressure_macro :
renoise.InstrumentMacro
- volume :
number
- volume_observable :
renoise.Document.Observable
- transpose :
integer
- transpose_observable :
renoise.Document.Observable
- trigger_options :
renoise.InstrumentTriggerOptions
- sample_mapping_overlap_mode :
renoise.Instrument.OverlapMode
- sample_mapping_overlap_mode_observable :
renoise.Document.Observable
- phrase_editor_visible :
boolean
- phrase_editor_visible_observable :
renoise.Document.Observable
- phrase_playback_mode :
renoise.Instrument.PhrasePlaybackMode
- phrase_playback_mode_observable :
renoise.Document.Observable
- phrase_program :
integer
- phrase_program_observable :
renoise.Document.Observable
- phrases :
renoise.InstrumentPhrase
[] - phrases_observable :
renoise.Document.ObservableList
- phrase_mappings :
renoise.InstrumentPhraseMapping
[] - phrase_mappings_observable :
renoise.Document.ObservableList
- samples :
renoise.Sample
[] - samples_observable :
renoise.Document.ObservableList
- sample_mappings :
renoise.SampleMapping
[] - sample_mappings_observable :
renoise.Document.ObservableList
- sample_modulation_sets :
renoise.SampleModulationSet
[] - sample_modulation_sets_observable :
renoise.Document.ObservableList
- sample_device_chains :
renoise.SampleDeviceChain
[] - sample_device_chains_observable :
renoise.Document.ObservableList
- midi_input_properties :
renoise.InstrumentMidiInputProperties
- midi_output_properties :
renoise.InstrumentMidiOutputProperties
- plugin_properties :
renoise.InstrumentPluginProperties
- active_tab :
- Functions
- clear(self)
- copy_from(self, instrument :
renoise.Instrument
) - macro(self, index :
integer
) - insert_phrase_at(self, index :
integer
) - delete_phrase_at(self, index :
integer
) - phrase(self, index :
integer
) - can_insert_phrase_mapping_at(self, index :
integer
) - insert_phrase_mapping_at(self, index :
integer
, phrase :renoise.InstrumentPhrase
) - delete_phrase_mapping_at(self, index :
integer
) - phrase_mapping(self, index :
integer
) - insert_sample_at(self, index :
integer
) - delete_sample_at(self, index :
integer
) - swap_samples_at(self, index1 :
integer
, index2 :integer
) - sample(self, index :
integer
) - sample_mapping(self, layer :
renoise.Instrument.Layer
, index :integer
) - insert_sample_modulation_set_at(self, index :
integer
) - delete_sample_modulation_set_at(self, index :
integer
) - swap_sample_modulation_sets_at(self, index1 :
any
, index2 :any
) - sample_modulation_set(self, index :
integer
) - insert_sample_device_chain_at(self, index :
integer
) - delete_sample_device_chain_at(self, index :
integer
) - swap_sample_device_chains_at(self, index1 :
integer
, index2 :integer
) - sample_device_chain(self, index :
integer
)
Constants
Tab
{ TAB_SAMPLES: integer = 1, TAB_PLUGIN: integer = 2, TAB_EXT_MIDI: integer = 3, }
PhrasePlaybackMode
{ PHRASES_OFF: integer = 1, PHRASES_PLAY_SELECTIVE: integer = 2, PHRASES_PLAY_KEYMAP: integer = 3, }
Layer
{ LAYER_NOTE_DISABLED: integer = 0, LAYER_NOTE_ON: integer = 1, LAYER_NOTE_OFF: integer = 2, }
OverlapMode
{ OVERLAP_MODE_ALL: integer = 0, OVERLAP_MODE_CYCLED: integer = 1, OVERLAP_MODE_RANDOM: integer = 2, }
NUMBER_OF_MACROS : integer
MAX_NUMBER_OF_PHRASES : integer
Properties
active_tab : renoise.Instrument.Tab
Currently active tab in the instrument GUI (samples, plugin or MIDI).
active_tab_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
name : string
Instrument's name.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
comments : string
[]
Instrument's comment list. See renoise.song().comments for more info on how to get notified on changes and how to change it.
comments_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
comments_assignment_observable : renoise.Document.Observable
Notifier which is called as soon as any paragraph in the comments change.
show_comments_after_loading : boolean
Set this to true to show the comments dialog after loading a song
show_comments_after_loading_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
macros_visible : boolean
Macro parameter pane visibility in the GUI.
macros_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
macros : renoise.InstrumentMacro
[]
READ-ONLY Macro parameters. Array with size Instrument.NUMBER_OF_MACROS.
pitchbend_macro : renoise.InstrumentMacro
Access the MIDI pitch-bend macro
modulation_wheel_macro : renoise.InstrumentMacro
Access the MIDI modulation-wheel macro
channel_pressure_macro : renoise.InstrumentMacro
Access the MIDI channel pressure macro
volume : number
Range: (0 - math.db2lin(6))
volume_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
transpose : integer
Range: (-120 - 120). Global relative pitch in semi tones. Applied to all samples, MIDI and plugins in the instrument.
transpose_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
trigger_options : renoise.InstrumentTriggerOptions
Global trigger options (quantization and scaling options).
sample_mapping_overlap_mode : renoise.Instrument.OverlapMode
Sample mapping's overlap trigger mode.
sample_mapping_overlap_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
phrase_editor_visible : boolean
Phrase editor pane visibility in the GUI.
phrase_editor_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
phrase_playback_mode : renoise.Instrument.PhrasePlaybackMode
Phrase playback.
phrase_playback_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
phrase_program : integer
Phrase playback program: 0 = Off, 1-126 = specific phrase, 127 = keymap.
phrase_program_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
phrases : renoise.InstrumentPhrase
[]
READ-ONLY Phrases.
phrases_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
phrase_mappings : renoise.InstrumentPhraseMapping
[]
READ-ONLY Phrase mappings.
phrase_mappings_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
samples : renoise.Sample
[]
READ-ONLY Samples slots.
samples_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
sample_mappings : renoise.SampleMapping
[]
READ-ONLY Sample mappings (key/velocity to sample slot mappings). sample_mappings[LAYER_NOTE_ON/OFF][]. Sample mappings also can be accessed via ---@field samples[].sample_mapping
sample_mappings_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
sample_modulation_sets : renoise.SampleModulationSet
[]
READ-ONLY Sample modulation sets.
sample_modulation_sets_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
sample_device_chains : renoise.SampleDeviceChain
[]
READ-ONLY Sample device chains.
sample_device_chains_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
midi_input_properties : renoise.InstrumentMidiInputProperties
READ-ONLY MIDI input properties.
midi_output_properties : renoise.InstrumentMidiOutputProperties
READ-ONLY MIDI output properties.
plugin_properties : renoise.InstrumentPluginProperties
READ-ONLY Plugin properties.
Functions
clear(self)
Reset, clear all settings and all samples.
copy_from(self, instrument : renoise.Instrument
)
Copy all settings from the other instrument, including all samples.
macro(self, index : integer
)
->
instrument_macro : renoise.InstrumentMacro
Range: (1 - renoise.Instrument.NUMBER_OF_MACROS) Access a single macro by index. See also property
macros
.
insert_phrase_at(self, index : integer
)
->
new_phrase : renoise.InstrumentPhrase
Insert a new phrase behind the given phrase index (1 for the first one).
delete_phrase_at(self, index : integer
)
Delete a new phrase at the given phrase index.
phrase(self, index : integer
)
Access a single phrase by index. Use properties 'phrases' to iterate over all phrases and to query the phrase count.
can_insert_phrase_mapping_at(self, index : integer
)
->
boolean
Returns true if a new phrase mapping can be inserted at the given phrase mapping index (see See renoise.song().instruments[].phrase_mappings). Passed phrase must exist and must not have a mapping yet. Phrase note mappings may not overlap and are sorted by note, so there can be max 119 phrases per instrument when each phrase is mapped to a single key only. To make up room for new phrases, access phrases by index, adjust their note_range, then call 'insert_phrase_mapping_at' again.
insert_phrase_mapping_at(self, index : integer
, phrase : renoise.InstrumentPhrase
)
->
new_mapping : renoise.InstrumentPhraseMapping
Insert a new phrase mapping behind the given phrase mapping index. The new phrase mapping will by default use the entire free (note) range between the previous and next phrase (if any). To adjust the note range of the new phrase change its 'new_phrase_mapping.note_range' property.
delete_phrase_mapping_at(self, index : integer
)
Delete a new phrase mapping at the given phrase mapping index.
phrase_mapping(self, index : integer
)
->
renoise.InstrumentPhraseMapping
Access to a phrase note mapping by index. Use property 'phrase_mappings' to iterate over all phrase mappings and to query the phrase (mapping) count.
insert_sample_at(self, index : integer
)
->
new_sample : renoise.Sample
Insert a new empty sample. returns the new renoise.Sample object. Every newly inserted sample has a default mapping, which covers the entire key and velocity range, or it gets added as drum kit mapping when the instrument used a drum-kit mapping before the sample got added.
delete_sample_at(self, index : integer
)
Delete an existing sample.
swap_samples_at(self, index1 : integer
, index2 : integer
)
Swap positions of two samples.
sample(self, index : integer
)
Access to a single sample by index. Use properties 'samples' to iterate over all samples and to query the sample count.
sample_mapping(self, layer : renoise.Instrument.Layer
, index : integer
)
Access to a sample mapping by index. Use property 'sample_mappings' to iterate over all sample mappings and to query the sample (mapping) count.
insert_sample_modulation_set_at(self, index : integer
)
->
new_sample_modulation_set : renoise.SampleModulationSet
Insert a new modulation set at the given index
delete_sample_modulation_set_at(self, index : integer
)
Delete an existing modulation set at the given index.
swap_sample_modulation_sets_at(self, index1 : any
, index2 : any
)
Swap positions of two modulation sets.
sample_modulation_set(self, index : integer
)
Access to a single sample modulation set by index. Use property 'sample_modulation_sets' to iterate over all sets and to query the set count.
insert_sample_device_chain_at(self, index : integer
)
->
new_sample_device_chain : renoise.SampleDeviceChain
Insert a new sample device chain at the given index.
delete_sample_device_chain_at(self, index : integer
)
Delete an existing sample device chain at the given index.
swap_sample_device_chains_at(self, index1 : integer
, index2 : integer
)
Swap positions of two sample device chains.
sample_device_chain(self, index : integer
)
Access to a single device chain by index. Use property 'sample_device_chains' to iterate over all chains and to query the chain count.
renoise.InstrumentDevice
Properties
name : string
READ-ONLY Device name.
short_name : string
READ-ONLY
presets : string
[]
READ-ONLY
active_preset : integer
Preset handling. 0 when when none is active (or available)
active_preset_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_preset_data : string
raw XML data of the active preset
parameters : renoise.DeviceParameter
[]
READ-ONLY
external_editor_available : boolean
READ-ONLY Returns whether or not the plugin provides its own custom GUI.
external_editor_visible : boolean
When the plugin has no custom GUI, Renoise will create a dummy editor for it which lists the plugin parameters. set to true to show the editor, false to close it
device_path : string
READ-ONLY Returns a string that uniquely identifies the plugin The string can be passed into: renoise.InstrumentPluginProperties:load_plugin()
Functions
preset(self, index : integer
)
->
string
Access to a single preset name by index. Use properties 'presets' to iterate over all presets and to query the presets count.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.InstrumentMacro
Properties
name : string
Macro name as visible in the GUI when mappings are presents.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
value : number
Range: (0 - 1)
value_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
value_string : string
Range: (0 - 100)
value_string_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mappings : renoise.InstrumentMacroMapping
[]
READ-ONLY Macro mappings, target parameters
mappings_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
mapping(self, index : integer
)
->
renoise.InstrumentMacroMapping
Access to a single attached parameter mapping by index. Use property 'mappings' to query mapping count.
renoise.InstrumentMacroMapping
- Constants
- Properties
- parameter :
renoise.DeviceParameter
- parameter_min :
number
- parameter_min_observable :
renoise.Document.Observable
- parameter_max :
number
- parameter_max_observable :
renoise.Document.Observable
- parameter_scaling :
renoise.InstrumentMacroMapping.Scaling
- parameter_scaling_observable :
renoise.Document.Observable
- parameter :
Constants
Scaling
{ SCALING_LOG_FAST: integer = 1, SCALING_LOG_SLOW: integer = 2, SCALING_LINEAR: integer = 3, SCALING_EXP_SLOW: integer = 4, SCALING_EXP_FAST: integer = 5, }
Properties
parameter : renoise.DeviceParameter
READ-ONLY Linked parameter. Can be a sample FX- or modulation parameter. Never nil.
parameter_min : number
Range: (0 - 1)
parameter_min_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
parameter_max : number
Range: (0 - 1)
parameter_max_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
parameter_scaling : renoise.InstrumentMacroMapping.Scaling
Scaling which gets applied within the min/max range to set the dest value.
parameter_scaling_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.InstrumentMidiInputProperties
Properties
device_name : string
When setting new devices, device names must be one of renoise.Midi.available_input_devices. Devices are automatically opened when needed. To close a device, set its name to "", e.g. an empty string.
device_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
channel : integer
Range: (1 - 16) 0 = Omni
channel_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
note_range : integer
[]
Table of two numbers in range (0-119) where C-4 is 48
note_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
assigned_track : integer
Range: (1 - song.sequencer_track_count) 0 = Current track
assigned_track_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.InstrumentMidiOutputProperties
- Constants
- Properties
- instrument_type :
renoise.InstrumentMidiOutputProperties.Type
- instrument_type_observable :
renoise.Document.Observable
- device_name :
string
- device_name_observable :
renoise.Document.Observable
- channel :
integer
- channel_observable :
renoise.Document.Observable
- transpose :
integer
- transpose_observable :
renoise.Document.Observable
- program :
integer
- program_observable :
renoise.Document.Observable
- bank :
integer
- bank_observable :
renoise.Document.Observable
- delay :
integer
- delay_observable :
renoise.Document.Observable
- duration :
integer
- duration_observable :
renoise.Document.Observable
- instrument_type :
Constants
Type
{ TYPE_EXTERNAL: integer = 1, TYPE_LINE_IN_RET: integer = 2, TYPE_INTERNAL: integer = 3, }
Properties
instrument_type : renoise.InstrumentMidiOutputProperties.Type
Note: ReWire device always start with "ReWire: " in the device_name and will always ignore the instrument_type and channel properties. MIDI channels are not configurable for ReWire MIDI, and instrument_type will always be "TYPE_INTERNAL" for ReWire devices.
instrument_type_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
device_name : string
When setting new devices, device names must be one of: renoise.Midi.available_output_devices. Devices are automatically opened when needed. To close a device, set its name to "", e.g. an empty string.
device_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
channel : integer
Range: (1 - 16)
channel_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
transpose : integer
Range: (-120 - 120)
transpose_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
program : integer
Range: (1 - 128) 0 = OFF
program_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bank : integer
Range: (1 - 65536) 0 = OFF
bank_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
delay : integer
Range: (0 - 100)
delay_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
duration : integer
Range: (1 - 8000) 8000 = INF
duration_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.InstrumentPhrase
General remarks: Phrases do use renoise.PatternLine objects just like the pattern tracks do. When the instrument column is enabled and used, not instruments, but samples are addressed/triggered in phrases.
- Constants
- Properties
- name :
string
- name_observable :
renoise.Document.Observable
- mapping :
renoise.InstrumentPhraseMapping
- is_empty :
boolean
- is_empty_observable :
renoise.Document.Observable
- number_of_lines :
integer
- number_of_lines_observable :
renoise.Document.Observable
- lines :
renoise.PatternLine
[] - visible_note_columns :
integer
- visible_note_columns_observable :
renoise.Document.Observable
- visible_effect_columns :
integer
- visible_effect_columns_observable :
renoise.Document.Observable
- key_tracking :
renoise.InstrumentPhrase.KeyTrackingMode
- key_tracking_observable :
renoise.Document.Observable
- base_note :
integer
- base_note_observable :
renoise.Document.Observable
- looping :
boolean
- looping_observable :
renoise.Document.Observable
- loop_start :
integer
- loop_start_observable :
renoise.Document.Observable
- loop_end :
integer
- loop_end_observable :
renoise.Document.Observable
- autoseek :
boolean
- autoseek_observable :
renoise.Document.Observable
- lpb :
integer
- lpb_observable :
renoise.Document.Observable
- shuffle :
number
- shuffle_observable :
renoise.Document.Observable
- instrument_column_visible :
boolean
- instrument_column_visible_observable :
renoise.Document.Observable
- volume_column_visible :
boolean
- volume_column_visible_observable :
renoise.Document.Observable
- panning_column_visible :
boolean
- panning_column_visible_observable :
renoise.Document.Observable
- delay_column_visible :
boolean
- delay_column_visible_observable :
renoise.Document.Observable
- sample_effects_column_visible :
boolean
- sample_effects_column_visible_observable :
renoise.Document.Observable
- name :
- Functions
- clear(self)
- copy_from(self, phrase :
renoise.InstrumentPhrase
) - line(self, index :
integer
) - lines_in_range(self, index_from :
integer
, index_to :integer
) - has_line_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - add_line_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - remove_line_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - has_line_edited_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - add_line_edited_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - remove_line_edited_notifier(self, func :
PhraseLineChangeCallbackWithContext
, obj :table
|userdata
) - column_is_muted(self, column :
integer
) - column_is_muted_observable(self, column :
integer
) - set_column_is_muted(self, column :
integer
, muted :boolean
) - column_name(self, column :
integer
) - column_name_observable(self, column :
integer
) - set_column_name(self, column :
integer
, name :string
) - swap_note_columns_at(self, index1 :
integer
, index2 :integer
) - swap_effect_columns_at(self, index1 :
integer
, index2 :integer
)
- Local Structs
- PhraseLinePosition
Constants
KeyTrackingMode
{ KEY_TRACKING_NONE: integer = 1, KEY_TRACKING_TRANSPOSE: integer = 2, KEY_TRACKING_OFFSET: integer = 3, }
MAX_NUMBER_OF_LINES : integer
Maximum number of lines that can be present in a phrase.
MIN_NUMBER_OF_NOTE_COLUMNS : integer
Min/Maximum number of note columns that can be present in a phrase.
MAX_NUMBER_OF_NOTE_COLUMNS : integer
MIN_NUMBER_OF_EFFECT_COLUMNS : integer
Min/Maximum number of effect columns that can be present in a phrase.
MAX_NUMBER_OF_EFFECT_COLUMNS : integer
Properties
name : string
Name of the phrase as visible in the phrase editor and piano mappings.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mapping : renoise.InstrumentPhraseMapping
(Key)Mapping properties of the phrase or nil when no mapping is present.
is_empty : boolean
READ-ONLY Quickly check if a phrase has some non empty pattern lines.
is_empty_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
number_of_lines : integer
Default: 16, Range: (1 - renoise.InstrumentPhrase.MAX_NUMBER_OF_LINES) Number of lines the phrase currently has.
number_of_lines_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
lines : renoise.PatternLine
[]
READ-ONLY Get all lines in a range [1, number_of_lines_in_pattern]
visible_note_columns : integer
Range: (MIN_NUMBER_OF_NOTE_COLUMNS - MAX_NUMBER_OF_NOTE_COLUMNS) How many note columns are visible in the phrase.
visible_note_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
visible_effect_columns : integer
Range: (MIN_NUMBER_OF_EFFECT_COLUMNS - MAX_NUMBER_OF_EFFECT_COLUMNS) How many effect columns are visible in the phrase.
visible_effect_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
key_tracking : renoise.InstrumentPhrase.KeyTrackingMode
Phrase's key-tracking mode.
key_tracking_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
base_note : integer
Range: (0 - 119) where C-4 is 48 Phrase's base-note. Only relevant when key_tracking is set to transpose.
base_note_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
looping : boolean
Loop mode. The phrase plays as one-shot when disabled.
looping_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_start : integer
Range: (1 - number_of_lines) Loop start. Playback will start from the beginning before entering loop
loop_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_end : integer
Range: (loop_start - number_of_lines) Loop end. Needs to be > loop_start and <= number_of_lines
loop_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
autoseek : boolean
Phrase autoseek settings
autoseek_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
lpb : integer
Range: (1 - 256) Phrase local lines per beat setting. New phrases get initialized with the song's current LPB setting. TPL can not be configured in phrases.
lpb_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
shuffle : number
Range: (0 - 1) Shuffle groove amount for a phrase. 0.0 = no shuffle (off), 1.0 = full shuffle
shuffle_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
instrument_column_visible : boolean
Column visibility.
instrument_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume_column_visible : boolean
volume_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
panning_column_visible : boolean
panning_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
delay_column_visible : boolean
delay_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sample_effects_column_visible : boolean
sample_effects_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
clear(self)
Deletes all lines.
copy_from(self, phrase : renoise.InstrumentPhrase
)
Copy contents from another phrase.
line(self, index : integer
)
Range: (1 - renoise.InstrumentPhrase.MAX_NUMBER_OF_LINES) Access to a single line by index. Line must be in Range: (1 - MAX_NUMBER_OF_LINES). This is a !lot! more efficient than calling the property: lines[index] to randomly access lines.
lines_in_range(self, index_from : integer
, index_to : integer
)
Get a specific line range
has_line_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
->
boolean
Check/add/remove notifier functions or methods, which are called by Renoise as soon as any of the phrases's lines have changed. See: renoise.Pattern.has_line_notifier for more details.
add_line_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
remove_line_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
has_line_edited_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
->
boolean
Same as line_notifier above, but the notifier only fires when the user added, changed or deleted a line with the computer keyboard. See:
renoise.Pattern.has_line_editoed_notifierfor more details.
add_line_edited_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
remove_line_edited_notifier(self, func : PhraseLineChangeCallbackWithContext
, obj : table
| userdata
)
column_is_muted(self, column : integer
)
->
boolean
Note column mute states.
column_is_muted_observable(self, column : integer
)
set_column_is_muted(self, column : integer
, muted : boolean
)
column_name(self, column : integer
)
->
string
Note column names.
column_name_observable(self, column : integer
)
set_column_name(self, column : integer
, name : string
)
swap_note_columns_at(self, index1 : integer
, index2 : integer
)
Swap the positions of two note columns within a phrase.
swap_effect_columns_at(self, index1 : integer
, index2 : integer
)
Swap the positions of two effect columns within a phrase.
Local Structs
PhraseLinePosition
Line iterator position.
Properties
line : integer
Local Aliases
PhraseLineChangeCallbackWithContext
(obj : table
| userdata
, pos : PhraseLinePosition
)
renoise.InstrumentPhraseMapping
- Constants
- Properties
- phrase :
renoise.InstrumentPhrase
- key_tracking :
renoise.InstrumentPhraseMapping.KeyTrackingMode
- key_tracking_observable :
renoise.Document.Observable
- base_note :
integer
- base_note_observable :
renoise.Document.Observable
- note_range :
integer
[] - note_range_observable :
renoise.Document.Observable
- looping :
boolean
- looping_observable :
renoise.Document.Observable
- loop_start :
integer
- loop_start_observable :
renoise.Document.Observable
- loop_end :
integer
- loop_end_observable :
renoise.Document.Observable
- phrase :
Constants
KeyTrackingMode
{ KEY_TRACKING_NONE: integer = 1, KEY_TRACKING_TRANSPOSE: integer = 2, KEY_TRACKING_OFFSET: integer = 3, }
Properties
phrase : renoise.InstrumentPhrase
Linked phrase.
key_tracking : renoise.InstrumentPhraseMapping.KeyTrackingMode
Phrase's key-tracking mode.
key_tracking_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
base_note : integer
Range: (0 - 119) where C-4 is 48
base_note_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
note_range : integer
[]
Range: (0 - 119) where C-4 is 48
note_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
looping : boolean
Loop mode. The phrase plays as one-shot when disabled.
looping_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_start : integer
loop_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_end : integer
loop_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.InstrumentPluginDevice
Properties
name : string
READ-ONLY Device name.
short_name : string
READ-ONLY
presets : string
[]
READ-ONLY
active_preset : integer
Preset handling. 0 when when none is active (or available)
active_preset_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
active_preset_data : string
raw XML data of the active preset
parameters : renoise.DeviceParameter
[]
READ-ONLY
external_editor_available : boolean
READ-ONLY Returns whether or not the plugin provides its own custom GUI.
external_editor_visible : boolean
When the plugin has no custom GUI, Renoise will create a dummy editor for it which lists the plugin parameters. set to true to show the editor, false to close it
device_path : string
READ-ONLY Returns a string that uniquely identifies the plugin The string can be passed into: renoise.InstrumentPluginProperties:load_plugin()
Functions
preset(self, index : integer
)
->
string
Access to a single preset name by index. Use properties 'presets' to iterate over all presets and to query the presets count.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.InstrumentPluginProperties
- Properties
- available_plugins :
string
[] - available_plugin_infos :
PluginInfo
[] - plugin_loaded :
boolean
- plugin_device :
renoise.AudioDevice
|renoise.InstrumentPluginDevice
- plugin_device_observable :
renoise.Document.Observable
- alias_instrument_index :
integer
- alias_instrument_index_observable :
renoise.Document.Observable
- alias_fx_track_index :
integer
- alias_fx_track_index_observable :
renoise.Document.Observable
- alias_fx_device_index :
integer
- alias_fx_device_index_observable :
renoise.Document.Observable
- midi_output_routing_index :
integer
- midi_output_routing_index_observable :
renoise.Document.Observable
- channel :
integer
- channel_observable :
renoise.Document.Observable
- transpose :
integer
- transpose_observable :
renoise.Document.Observable
- volume :
number
- volume_observable :
renoise.Document.Observable
- auto_suspend :
boolean
- auto_suspend_observable :
renoise.Document.Observable
- available_plugins :
- Local Structs
- PluginInfo
Properties
available_plugins : string
[]
READ-ONLY List of all currently available plugins. This is a list of unique plugin names which also contains the plugin's type (VST/AU/DSSI/...), not including the vendor names as visible in Renoise's GUI. So its an identifier, and not the name as visible in the GUI. When no plugin is loaded, the identifier is an empty string.
available_plugin_infos : PluginInfo
[]
READ-ONLY Returns a list of tables containing more information about the plugins.
plugin_loaded : boolean
READ-ONLY Returns true when a plugin is present; loaded successfully.
plugin_device : renoise.AudioDevice
| renoise.InstrumentPluginDevice
Valid object for successfully loaded plugins, otherwise nil. Alias plugin instruments of FX will return the resolved device, will link to the device the alias points to. The observable is fired when the device changes: when a plugin gets loaded or unloaded or a plugin alias is assigned or unassigned.
plugin_device_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
alias_instrument_index : integer
0 when no alias instrument is set
alias_instrument_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
alias_fx_track_index : integer
READ-ONLY 0 when no alias FX is set
alias_fx_track_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
alias_fx_device_index : integer
READ-ONLY 0 when no alias FX is set
alias_fx_device_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
midi_output_routing_index : integer
0 when no routing is set
midi_output_routing_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
channel : integer
Range: (1 - 16)
channel_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
transpose : integer
Range: (-120 - 120)
transpose_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume : number
Range: (0.0 - 4.0) linear gain
volume_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
auto_suspend : boolean
Valid for loaded and unloaded plugins.
auto_suspend_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Local Structs
PluginInfo
Properties
path : string
The plugin's path used by load_plugin()
name : string
The plugin's name
short_name : string
The plugin's name as displayed in shortened lists
favorite_name : string
The plugin's name as displayed in favorites
is_favorite : string
true if the plugin is a favorite
is_bridged : string
true if the plugin is a bridged plugin
renoise.InstrumentTriggerOptions
- Constants
- Properties
- available_scale_modes :
string
[] - scale_mode :
string
- scale_mode_observable :
renoise.Document.Observable
- scale_key :
integer
- scale_key_observable :
renoise.Document.Observable
- quantize :
renoise.InstrumentTriggerOptions.QuantizeMode
- quantize_observable :
renoise.Document.Observable
- monophonic :
boolean
- monophonic_observable :
renoise.Document.Observable
- monophonic_glide :
integer
- monophonic_glide_observable :
renoise.Document.Observable
- available_scale_modes :
Constants
QuantizeMode
{ QUANTIZE_NONE: integer = 1, QUANTIZE_LINE: integer = 2, QUANTIZE_BEAT: integer = 3, QUANTIZE_BAR: integer = 4, }
Properties
available_scale_modes : string
[]
READ-ONLY List of all available scale modes.
scale_mode : string
one of 'available_scales']
scale_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
scale_key : integer
Scale-key to use when transposing (1=C, 2=C#, 3=D, ...)
scale_key_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
quantize : renoise.InstrumentTriggerOptions.QuantizeMode
Trigger quantization mode.
quantize_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
monophonic : boolean
Mono/Poly mode.
monophonic_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
monophonic_glide : integer
Glide amount when monophonic. 0 == off, 255 = instant
monophonic_glide_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.Midi
Raw MIDI IO support for scripts in Renoise; the ability to send and receive MIDI data.
- Functions
available_input_devices()
available_output_devices()
devices_changed_observable()
- create_input_device(device_name :
string
, callback :MidiMessageFunction
|MidiMessageMethod1
|MidiMessageMethod2``?
, sysex_callback :MidiMessageFunction
|MidiMessageMethod1
|MidiMessageMethod2``?
) - create_output_device(device_name :
string
)
- Local Aliases
Functions
available_input_devices()
->
string
[]
Return a list of strings with currently available MIDI input devices. This list can change when devices are hot-plugged. See
renoise.Midi.devices_changed_observable
available_output_devices()
->
string
[]
Return a list of strings with currently available MIDI output devices. This list can change when devices are hot-plugged. See
renoise.Midi.devices_changed_observable
devices_changed_observable()
Fire notifications as soon as new devices become active or a previously added device gets removed/unplugged. This will only happen on Linux and OSX with real devices. On Windows this may happen when using ReWire slaves. ReWire adds virtual MIDI devices to Renoise. Already opened references to devices which are no longer available will do nothing: ou can use them as before and they will not fire any errors. The messages will simply go into the void...
create_input_device(device_name : string
, callback : MidiMessageFunction
| MidiMessageMethod1
| MidiMessageMethod2
?
, sysex_callback : MidiMessageFunction
| MidiMessageMethod1
| MidiMessageMethod2
?
)
->
renoise.Midi.MidiInputDevice
Listen to incoming MIDI data: opens access to a MIDI input device by specifying a device name. Name must be one of
renoise.Midi.available_input_devices()
.One or both callbacks should be valid, and should either point to a function with one parameter
function (message: number[]) end
, or a table with an object and class{context, function(context, message: number[]) end}
-> a method.All MIDI messages except active sensing will be forwarded to the callbacks. When Renoise is already listening to this device, your callback and Renoise (or even other scripts) will handle the message.
Messages are received until the device reference is manually closed (see renoise.Midi.MidiDevice:close()) or until the MidiInputDevice object gets garbage collected.
create_output_device(device_name : string
)
->
renoise.Midi.MidiOutputDevice
Open access to a MIDI device by specifying the device name. Name must be one of
renoise.Midi.available_input_devices()
. All other device names will fire an error.The real device driver gets automatically closed when the MidiOutputDevice object gets garbage collected or when the device is explicitly closed via midi_device:close() and nothing else references it.
Local Aliases
MidiMessage
integer
[]
MidiMessageFunction
(message : MidiMessage
)
MidiMessageMemberContext
MidiMessageMemberFunction
(self : MidiMessageMemberContext
, message : MidiMessage
)
MidiMessageMethod1
{ 1 : MidiMessageMemberContext
, 2 : MidiMessageMemberFunction
}
MidiMessageMethod2
{ 1 : MidiMessageMemberFunction
, 2 : MidiMessageMemberContext
}
renoise.Midi.MidiDevice
Baseclass of renoise.Midi.MidiIn/OutDevice with common properties for MIDI input and output devices.
Properties
is_open : boolean
Returns true while the device is open (ready to send or receive messages). Your device refs will never be auto-closed, "is_open" will only be false if you explicitly call "midi_device:close()" to release a device.
name : string
The name of a device. This is the name you create a device with via
renoise.Midi.create_input_device
orrenoise.Midi.create_output_device
.
renoise.Midi.MidiInputDevice
Midi device interface for receiving MIDI messages. Instances are created via
renoise.Midi.create_input_device
Properties
is_open : boolean
Returns true while the device is open (ready to send or receive messages). Your device refs will never be auto-closed, "is_open" will only be false if you explicitly call "midi_device:close()" to release a device.
name : string
The name of a device. This is the name you create a device with via
renoise.Midi.create_input_device
orrenoise.Midi.create_output_device
.
renoise.Midi.MidiOutputDevice
Midi device interface for sending MIDI messages. Instances are created via
renoise.Midi.create_output_device
Properties
is_open : boolean
Returns true while the device is open (ready to send or receive messages). Your device refs will never be auto-closed, "is_open" will only be false if you explicitly call "midi_device:close()" to release a device.
name : string
The name of a device. This is the name you create a device with via
renoise.Midi.create_input_device
orrenoise.Midi.create_output_device
.
Functions
send(self, message : integer
[])
Send raw 1-3 byte MIDI messages or sysex messages. Message is expected to be an array of numbers. It must not be empty and can only contain numbers >= 0 and <= 0xFF (bytes). Sysex messages must be sent in one block, and must start with 0xF0, and end with 0xF7.
renoise.NoteColumn
A single note column in a pattern line.
General remarks: instrument columns are available for lines in phrases but are ignored. See renoise.InstrumentPhrase for detail.
Access note column properties either by values (numbers) or by strings. The string representation uses exactly the same notation as you see them in Renoise's pattern or phrase editor.
- Properties
- is_empty :
boolean
- is_selected :
boolean
- note_value :
integer
- note_string :
string
- instrument_value :
integer
- instrument_string :
string
- volume_value :
integer
- volume_string :
string
- panning_value :
integer
- panning_string :
string
- delay_value :
integer
- delay_string :
string
- effect_number_value :
integer
- effect_number_string :
string
- effect_amount_value :
integer
- effect_amount_string :
string
- is_empty :
- Functions
Properties
is_empty : boolean
READ-ONLY True, when all note column properties are empty.
is_selected : boolean
READ-ONLY True, when this column is selected in the pattern or phrase editors current pattern.
note_value : integer
Range: (0-119) or 120=Off or 121=Empty
note_string : string
Range: ('C-0'-'G-9') or 'OFF' or '---'
instrument_value : integer
Range: (0-254), 255==Empty
instrument_string : string
Range: ('00'-'FE') or '..'
volume_value : integer
Range: (0-127) or 255==Empty when column value is <= 0x80 or is 0xFF, i.e. to specify a volume value.
Range: (0-65535) in the form 0x0000xxyy where xx=effect char 1 and yy=effect char 2, when column value is > 0x80, i.e. to specify an effect.
volume_string : string
Range('00'-'ZF') or '..'
panning_value : integer
Range: (0-127) or 255==Empty when column value is <= 0x80 or is 0xFF, i.e. to specify a pan value.
Range: (0-65535) in the form 0x0000xxyy where xx=effect char 1 and yy=effect char 2, when column value is > 0x80, i.e. to specify an effect.
panning_string : string
Range: ('00'-'ZF') or '..'
delay_value : integer
Range: (0-255)
delay_string : string
Range: ('00'-'FF') or '..'
effect_number_value : integer
Range: (0-65535) in the form 0x0000xxyy where xx=effect char 1 and yy=effect char 2
effect_number_string : string
Range: ('00'-'ZZ')
effect_amount_value : integer
Range: (0-255)
effect_amount_string : string
Range: ('00' - 'FF')
Functions
clear(self)
Clear the note column.
copy_from(self, other : renoise.NoteColumn
)
Copy the column's content from another column.
renoise.Osc
OSC (Open Sound Control) support for Lua scripts in Renoise.
Functions
from_binary_data(binary_data : string
)
->
renoise.Osc.Bundle
| renoise.Osc.Message
?
, string
?
De-packetizing raw (socket) data to OSC messages or bundles: Converts the binary data to an OSC message or bundle. If the data does not look like an OSC message, or the message contains errors, nil is returned as first argument and the second return value will contain the error. If de-packetizing was successful, either a renoise.Osc.Bundle or Message object is returned. Bundles may contain multiple messages or nested bundles.
Message(pattern : string
, arguments : OscValue
[]?
)
Create a new OSC message with the given pattern and optional arguments.
Bundle(time : integer
, arguments : renoise.Osc.Message
| renoise.Osc.Message
[])
Create a new bundle by specifying a time-tag and one or more messages. If you do not know what to do with the time-tag, use
os.clock()
, which simply means "now". Messages must be renoise.Osc.Message objects. Nested bundles (bundles in bundles) are right now not supported.
Local Structs
OscValue
tag
is a standard OSC type tag.value
is the arguments value expressed by a Lua type. The value must be convertible to the specified tag, which means, you cannot for example specify an "i" (integer) as type and then pass a string as the value. Use a number value instead. Not all tags require a value, like the T,F boolean tags. Then avalue
field should not be specified. For more info, see: http://opensoundcontrol.org/spec-1_0
Properties
tag : OscTag
value : boolean
| string
| number
Local Aliases
OscTag
"F"
| "I"
| "N"
| "S"
| "T"
| "b"
| "c"
| "d"
| "f"
| "h"
| "i"
| "m"
| "r"
| "s"
| "t"
OscTag: | "i" -- int32 | "f" -- float32 | "s" -- OSC-string | "b" -- OSC-blob (raw string) | "h" -- 64 bit big-endian two's complement integer | "t" -- OSC-timetag | "d" -- 64 bit ("double") IEEE 754 floating point number | "S" -- Alternate type represented as an OSC-string | "c" -- An ascii character, sent as 32 bits | "r" -- 32 bit RGBA color | "m" -- 4 byte MIDI message. Bytes from MSB to LSB are: port id, status byte, data1, data2 | "T" -- True. No value needs to be specified. | "F" -- False. No value needs to be specified. | "N" -- Nil. No value needs to be specified. | "I" -- Infinitum. No value needs to be specified.
renoise.Osc.Bundle
Properties
timetag : number
READ-ONLY Time value of the bundle.
elements : renoise.Osc.Bundle
| renoise.Osc.Message
[]
READ-ONLY Access to the bundle elements (table of messages or bundles)
binary_data : string
READ-ONLY Raw binary representation of the bundle, as needed when e.g. sending the message over the network through sockets.
renoise.Osc.Message
Properties
pattern : string
READ-ONLY The message pattern (e.g. "/renoise/transport/start")
arguments : OscValue
[]
READ-ONLY Table of
{tag="X", value=SomeValue}
that represents the message arguments. Seerenoise.Osc.Message.create
for more info.
binary_data : string
READ-ONLY Raw binary representation of the message, as needed when e.g. sending the message over the network through sockets.
Local Structs
OscValue
tag
is a standard OSC type tag.value
is the arguments value expressed by a Lua type. The value must be convertible to the specified tag, which means, you cannot for example specify an "i" (integer) as type and then pass a string as the value. Use a number value instead. Not all tags require a value, like the T,F boolean tags. Then avalue
field should not be specified. For more info, see: http://opensoundcontrol.org/spec-1_0
Properties
tag : OscTag
value : boolean
| string
| number
Local Aliases
OscTag
"F"
| "I"
| "N"
| "S"
| "T"
| "b"
| "c"
| "d"
| "f"
| "h"
| "i"
| "m"
| "r"
| "s"
| "t"
OscTag: | "i" -- int32 | "f" -- float32 | "s" -- OSC-string | "b" -- OSC-blob (raw string) | "h" -- 64 bit big-endian two's complement integer | "t" -- OSC-timetag | "d" -- 64 bit ("double") IEEE 754 floating point number | "S" -- Alternate type represented as an OSC-string | "c" -- An ascii character, sent as 32 bits | "r" -- 32 bit RGBA color | "m" -- 4 byte MIDI message. Bytes from MSB to LSB are: port id, status byte, data1, data2 | "T" -- True. No value needs to be specified. | "F" -- False. No value needs to be specified. | "N" -- Nil. No value needs to be specified. | "I" -- Infinitum. No value needs to be specified.
renoise.Pattern
- Constants
- Properties
- Functions
- clear(self)
- copy_from(self, other :
renoise.Pattern
) - track(self, index :
integer
) - has_line_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
) - add_line_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
) - remove_line_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
) - has_line_edited_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
) - add_line_edited_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
) - remove_line_edited_notifier(self, func :
PatternLineChangeCallbackWithContext
, obj :table
|userdata
)
- Local Structs
- PatternLinePosition
Constants
MAX_NUMBER_OF_LINES : integer
Maximum number of lines that can be present in a pattern.
Properties
is_empty : boolean
Quickly check if any track in a pattern has some non empty pattern lines. This does not look at track automation.
name : string
Name of the pattern, as visible in the pattern sequencer.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
number_of_lines : integer
Number of lines the pattern currently has. 64 by default. Max is renoise.Pattern.MAX_NUMBER_OF_LINES, min is 1.
number_of_lines_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tracks : renoise.PatternTrack
[]
READ-ONLY Access to the pattern tracks. Each pattern has #renoise.song().tracks amount of tracks.
Functions
clear(self)
Deletes all lines & automation.
copy_from(self, other : renoise.Pattern
)
Copy contents from other patterns, including automation, when possible.
track(self, index : integer
)
Access to a single pattern track by index. Use properties 'tracks' to iterate over all tracks and to query the track count.
has_line_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
->
boolean
Check/add/remove notifier functions or methods, which are called by Renoise as soon as any of the pattern's lines have changed. The notifiers are called as soon as a new line is added, an existing line is cleared, or existing lines are somehow changed (notes, effects, anything)
A single argument is passed to the notifier function: "pos", a table with the fields "pattern", "track" and "line", which defines where the change has happened.
examples:
function my_pattern_line_notifier(pos) -- check pos.pattern, pos.track, pos.line (all are indices) end
Please be gentle with these notifiers, don't do too much stuff in there. Ideally just set a flag like "pattern_dirty" which then gets picked up by an app_idle notifier: The danger here is that line change notifiers can be called hundreds of times when, for example, simply clearing a pattern.
If you are only interested in changes that are made to the currently edited pattern, dynamically attach and detach to the selected pattern's line notifiers by listening to "renoise.song().selected_pattern_observable".
add_line_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
remove_line_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
has_line_edited_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
->
boolean
Same as
line_notifier
, but the notifier only fires when the user added, changed or deleted a line with the computer or MIDI keyboard.
add_line_edited_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
remove_line_edited_notifier(self, func : PatternLineChangeCallbackWithContext
, obj : table
| userdata
)
Local Structs
PatternLinePosition
Line iterator position.
Properties
pattern : integer
track : integer
line : integer
Local Aliases
PatternLineChangeCallbackWithContext
(obj : table
| userdata
, pos : PatternLinePosition
)
renoise.PatternIterator
Lua pairs/ipairs alike iterator functions to walk through all lines or columns in the entire song, track or a single pattern.
General remarks: Iterators can only be use in "for" loops like you would use "pairs" in Lua.
examples:
for pos,line in renoise.song().pattern_iterator:lines_in_song() do [...] end
The returned
pos
is a table with "pattern", "track", "line" fields, and an additional "column" field for the note/effect columns.The
visible_only
flag controls if all content should be traversed, or only the currently used patterns, columns, and so on. Patterns are traversed in the order they are referenced in the pattern sequence, but each pattern is accessed only once.
- Functions
- lines_in_song(self, visible_only :
boolean``?
) - note_columns_in_song(self, visible_only :
boolean``?
) - effect_columns_in_song(self, visible_only :
boolean``?
) - lines_in_pattern(self, pattern_index :
integer
) - note_columns_in_pattern(self, pattern_index :
integer
, visible_only :boolean``?
) - effect_columns_in_pattern(self, pattern_index :
integer
, visible_only :boolean``?
) - lines_in_track(self, track_index :
integer
, visible_only :boolean``?
) - note_columns_in_track(self, track_index :
integer
, visible_only :boolean``?
) - effect_columns_in_track(self, track_index :
integer
, visible_only :boolean``?
) - lines_in_pattern_track(self, pattern_index :
integer
, track_index :integer
) - note_columns_in_pattern_track(self, pattern_index :
integer
, track_index :integer
, visible_only :boolean``?
) - effect_columns_in_pattern_track(self, pattern_index :
integer
, track_index :integer
, visible_only :boolean``?
)
- lines_in_song(self, visible_only :
- Local Structs
- PatternColumnPosition
- PatternLinePosition
Functions
lines_in_song(self, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternLinePosition
, renoise.PatternLine
, line : renoise.PatternLine
, pos : PatternLinePosition
Iterate over all pattern lines in the song.
note_columns_in_song(self, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.NoteColumn
, column : renoise.NoteColumn
, pos : PatternColumnPosition
Iterate over all note columns in the song.
effect_columns_in_song(self, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.EffectColumn
, column : renoise.EffectColumn
, pos : PatternColumnPosition
Iterate over all effect columns in the song.
lines_in_pattern(self, pattern_index : integer
)
->
(context : unknown
) ->
PatternLinePosition
, renoise.PatternLine
, line : renoise.PatternLine
, pos : PatternLinePosition
Iterate over all lines in the given pattern only.
note_columns_in_pattern(self, pattern_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.NoteColumn
, column : renoise.NoteColumn
, pos : PatternColumnPosition
Iterate over all note columns in the specified pattern.
effect_columns_in_pattern(self, pattern_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.EffectColumn
, column : renoise.EffectColumn
, pos : PatternColumnPosition
Iterate over all note columns in the specified pattern.
lines_in_track(self, track_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternLinePosition
, renoise.PatternLine
, line : renoise.PatternLine
, pos : PatternLinePosition
Iterate over all lines in the given track only.
note_columns_in_track(self, track_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.NoteColumn
, column : renoise.NoteColumn
, pos : PatternColumnPosition
Iterate over all note/effect columns in the specified track.
effect_columns_in_track(self, track_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.EffectColumn
, column : renoise.EffectColumn
, pos : PatternColumnPosition
lines_in_pattern_track(self, pattern_index : integer
, track_index : integer
)
->
(context : unknown
) ->
PatternLinePosition
, renoise.PatternLine
, line : renoise.PatternLine
, pos : PatternLinePosition
Iterate over all lines in the given pattern, track only.
note_columns_in_pattern_track(self, pattern_index : integer
, track_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.NoteColumn
, column : renoise.NoteColumn
, pos : PatternColumnPosition
Iterate over all note/effect columns in the specified pattern track.
effect_columns_in_pattern_track(self, pattern_index : integer
, track_index : integer
, visible_only : boolean
?
)
->
(context : unknown
) ->
PatternColumnPosition
, renoise.EffectColumn
, column : renoise.EffectColumn
, pos : PatternColumnPosition
Local Structs
PatternColumnPosition
Note/Effect column iterator position.
Properties
pattern : integer
track : integer
line : integer
column : integer
PatternLinePosition
Line iterator position.
Properties
pattern : integer
track : integer
line : integer
renoise.PatternLine
Constants
EMPTY_NOTE : integer
NOTE_OFF : integer
EMPTY_INSTRUMENT : integer
EMPTY_VOLUME : integer
EMPTY_PANNING : integer
EMPTY_DELAY : integer
EMPTY_EFFECT_NUMBER : integer
EMPTY_EFFECT_AMOUNT : integer
Properties
is_empty : boolean
READ-ONLY
note_columns : renoise.NoteColumn
[]
READ-ONLY
effect_columns : renoise.EffectColumn
[]
READ-ONLY
Functions
clear(self)
Clear all note and effect columns.
copy_from(self, other : renoise.PatternLine
)
Copy contents from other_line, trashing column content.
note_column(self, index : integer
)
Access to a single note column by index. Use properties 'note_columns' to iterate over all note columns and to query the note_column count. This is a !lot! more efficient than calling the property: note_columns[index] to randomly access columns. When iterating over all columns, use pairs(note_columns).
effect_column(self, index : integer
)
Access to a single effect column by index. Use properties 'effect_columns' to iterate over all effect columns and to query the effect_column count. This is a !lot! more efficient than calling the property: effect_columns[index] to randomly access columns. When iterating over all columns, use pairs(effect_columns).
renoise.PatternSequencer
Pattern sequencer component of the Renoise song.
- Properties
- keep_sequence_sorted :
boolean
- keep_sequence_sorted_observable :
renoise.Document.Observable
- selection_range :
integer
[] - selection_range_observable :
renoise.Document.Observable
- pattern_sequence :
integer
[] - pattern_sequence_observable :
renoise.Document.ObservableList
- pattern_assignments_observable :
renoise.Document.Observable
- pattern_slot_mutes_observable :
renoise.Document.Observable
- keep_sequence_sorted :
- Functions
- insert_sequence_at(self, sequence_index :
integer
, pattern_index :integer
) - insert_new_pattern_at(self, sequence_index :
integer
) - delete_sequence_at(self, sequence_index :
any
) - pattern(self, sequence_index :
integer
) - clone_range(self, from_sequence_index :
integer
, to_sequence_index :integer
) - make_range_unique(self, from_sequence_index :
integer
, to_sequence_index :integer
) - sort(self)
- sequence_is_start_of_section(self, sequence_index :
integer
) - set_sequence_is_start_of_section(self, sequence_index :
integer
, is_section :boolean
) - sequence_is_start_of_section_observable(self, sequence_index :
integer
) - sequence_section_name(self, sequence_index :
integer
) - set_sequence_section_name(self, sequence_index :
integer
, name :string
) - sequence_section_name_observable(self, sequence_index :
integer
) - sequence_is_part_of_section(self, sequence_index :
integer
) - sequence_is_end_of_section(self, sequence_index :
integer
) - sequence_sections_changed_observable(self)
- track_sequence_slot_is_muted(self, track_index :
integer
, sequence_index :integer
) - set_track_sequence_slot_is_muted(self, track_index :
integer
, sequence_index :integer
, muted :any
) - track_sequence_slot_is_selected(self, track_index :
integer
, sequence_index :integer
) - set_track_sequence_slot_is_selected(self, track_index :
integer
, sequence_index :integer
, selected :any
)
- insert_sequence_at(self, sequence_index :
Properties
keep_sequence_sorted : boolean
When true, the sequence will be auto sorted.
keep_sequence_sorted_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_range : integer
[]
Access to the selected slots in the sequencer. When no selection is present
{0, 0}
is returned, else Range: (1 - #sequencer.pattern_sequence)
selection_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
pattern_sequence : integer
[]
Pattern order list: Notifiers will only be fired when sequence positions are added, removed or their order changed. To get notified of pattern assignment changes use the property
pattern_assignments_observable
.
pattern_sequence_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
pattern_assignments_observable : renoise.Document.Observable
Attach notifiers that will be called as soon as any pattern assignment at any sequence position changes.
pattern_slot_mutes_observable : renoise.Document.Observable
Attach notifiers that will be fired as soon as any slot muting property in any track/sequence slot changes.
Functions
insert_sequence_at(self, sequence_index : integer
, pattern_index : integer
)
Insert the specified pattern at the given position in the sequence.
insert_new_pattern_at(self, sequence_index : integer
)
->
new_pattern_index : integer
Insert an empty, unreferenced pattern at the given position.
delete_sequence_at(self, sequence_index : any
)
Delete an existing position in the sequence. Renoise needs at least one sequence in the song for playback. Completely removing all sequence positions is not allowed.
pattern(self, sequence_index : integer
)
->
pattern_index : integer
Access to a single sequence by index (the pattern number). Use property
pattern_sequence
to iterate over the whole sequence and to query the sequence count.
clone_range(self, from_sequence_index : integer
, to_sequence_index : integer
)
Clone a sequence range, appending it right after to_sequence_index. Slot muting is copied as well.
make_range_unique(self, from_sequence_index : integer
, to_sequence_index : integer
)
Make patterns in the given sequence pos range unique, if needed.
sort(self)
Sort patterns in the sequence in ascending order, keeping the old pattern data in place. This will only change the visual order of patterns, but not change the song's structure.
sequence_is_start_of_section(self, sequence_index : integer
)
Access to pattern sequence sections. When the
is_start_of_section flag
is set for a sequence pos, a section ranges from this pos to the next pos which starts a section, or till the end of the song when there are no others.
set_sequence_is_start_of_section(self, sequence_index : integer
, is_section : boolean
)
sequence_is_start_of_section_observable(self, sequence_index : integer
)
sequence_section_name(self, sequence_index : integer
)
->
string
Access to a pattern sequence section's name. Section names are only visible for a sequence pos which starts the section (see
sequence_is_start_of_section
).
set_sequence_section_name(self, sequence_index : integer
, name : string
)
sequence_section_name_observable(self, sequence_index : integer
)
sequence_is_part_of_section(self, sequence_index : integer
)
->
boolean
Returns true if the given sequence pos is part of a section, else false.
sequence_is_end_of_section(self, sequence_index : integer
)
->
boolean
Returns true if the given sequence pos is the end of a section, else false
sequence_sections_changed_observable(self)
Observable, which is fired, whenever the section layout in the sequence changed in any way, i.e. new sections got added, existing ones got deleted
track_sequence_slot_is_muted(self, track_index : integer
, sequence_index : integer
)
->
boolean
Access to sequencer slot mute states. Mute slots are memorized in the sequencer and not in the patterns.
set_track_sequence_slot_is_muted(self, track_index : integer
, sequence_index : integer
, muted : any
)
track_sequence_slot_is_selected(self, track_index : integer
, sequence_index : integer
)
->
boolean
Access to sequencer slot selection states.
set_track_sequence_slot_is_selected(self, track_index : integer
, sequence_index : integer
, selected : any
)
renoise.PatternTrack
- Properties
- is_alias :
boolean
- alias_pattern_index :
integer
- alias_pattern_index_observable :
renoise.Document.Observable
- color :
RGBColor``?
- color_observable :
renoise.Document.Observable
- is_empty :
boolean
- is_empty_observable :
renoise.Document.Observable
- lines :
renoise.PatternLine
[] - automation :
renoise.PatternTrackAutomation
[] - automation_observable :
renoise.Document.ObservableList
- is_alias :
- Functions
- clear(self)
- copy_from(self, other :
renoise.PatternTrack
) - line(self, index :
integer
) - lines_in_range(self, index_from :
integer
, index_to :integer
) - find_automation(self, parameter :
renoise.DeviceParameter
) - create_automation(self, parameter :
renoise.DeviceParameter
) - delete_automation(self, parameter :
renoise.DeviceParameter
)
- Local Aliases
Properties
is_alias : boolean
READ-ONLY
alias_pattern_index : integer
index or 0 when no alias is present
alias_pattern_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
color : RGBColor
?
slot color of the pattern in the matrix, nil when no slot color is set
color_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_empty : boolean
Returns true when all the track lines are empty. Does not look at automation.
is_empty_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
lines : renoise.PatternLine
[]
READ-ONLY Get all lines in range [1, number_of_lines_in_pattern]. Use
renoise.Pattern:add/remove_line_notifier
for change notifications.
automation : renoise.PatternTrackAutomation
[]
Automation.
automation_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
clear(self)
Deletes all lines & automation.
copy_from(self, other : renoise.PatternTrack
)
Copy contents from other pattern tracks, including automation when possible.
line(self, index : integer
)
Access to a single line by index. Line must be in Range: (1 - MAX_NUMBER_OF_LINES). This is a !lot! more efficient than calling the property: lines[index] to randomly access lines.
lines_in_range(self, index_from : integer
, index_to : integer
)
Get a specific line range. Index must be Range: (1 - Pattern.MAX_NUMBER_OF_LINES)
find_automation(self, parameter : renoise.DeviceParameter
)
->
renoise.PatternTrackAutomation
?
Returns the automation for the given device parameter or nil when there is none.
create_automation(self, parameter : renoise.DeviceParameter
)
->
renoise.PatternTrackAutomation
Creates a new automation for the given device parameter. Fires an error when an automation for the given parameter already exists. Returns the newly created automation. Passed parameter must be automatable, which can be tested with 'parameter.is_automatable'.
delete_automation(self, parameter : renoise.DeviceParameter
)
Remove an existing automation the given device parameter. Automation must exist.
Local Aliases
RGBColor
{ 1 : integer
, 2 : integer
, 3 : integer
}
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
renoise.PatternTrackAutomation
Graphical automation of a device parameter within a pattern track.
General remarks: Automation "time" is specified in lines + optional 1/256 line fraction for the sub line grid. The sub line grid has 256 units per line. All times are internally quantized to this sub line grid. For example a time of 1.5 means: line 1 with a note column delay of 128
- Constants
- Properties
- dest_device :
renoise.AudioDevice
- dest_parameter :
renoise.DeviceParameter
- playmode :
renoise.PatternTrackAutomation.Playmode
- playmode_observable :
renoise.Document.Observable
- length :
integer
- selection_start :
integer
- selection_start_observable :
renoise.Document.Observable
- selection_end :
integer
- selection_end_observable :
renoise.Document.Observable
- selection_range :
integer
[] - selection_range_observable :
renoise.Document.Observable
- points :
EnvelopePoint
[] - points_observable :
renoise.Document.ObservableList
- dest_device :
- Functions
- Local Structs
- EnvelopePoint
Constants
Playmode
{ PLAYMODE_POINTS: integer = 1, PLAYMODE_LINES: integer = 2, PLAYMODE_CURVES: integer = 3, }
Properties
dest_device : renoise.AudioDevice
Destination device. Can in some rare circumstances be nil, i.e. when a device or track is about to be deleted.
dest_parameter : renoise.DeviceParameter
Destination device's parameter. Can in some rare circumstances be nil, i.e. when a device or track is about to be deleted.
playmode : renoise.PatternTrackAutomation.Playmode
play-mode (interpolation mode).
playmode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
length : integer
Range: (1 - NUM_LINES_IN_PATTERN)
selection_start : integer
Range: (1 - automation.length + 1)
selection_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_end : integer
Range: (1 - automation.length + 1)
selection_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_range : integer
[]
Get or set selection range. when setting an empty table, the existing selection, if any, will be cleared. array of two numbers [] OR Range: (1 - automation.length + 1)
selection_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
points : EnvelopePoint
[]
Get all points of the automation. When setting a new list of points, items may be unsorted by time, but there may not be multiple points for the same time. Returns a copy of the list, so changing
points[1].value
will not do anything. Instead, change them viapoints = { modified_points }
.
points_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
clear(self)
Removes all points from the automation. Will not delete the automation from tracks[]:automation, instead the resulting automation will not do anything at all.
clear_range(self, from_time : integer
, to_time : integer
)
Remove all existing points in the given [from, to) time range from the automation.
copy_from(self, other : renoise.PatternTrackAutomation
)
Copy all points and playback settings from another track automation.
has_point_at(self, time : integer
)
->
boolean
Test if a point exists at the given time
add_point_at(self, time : integer
, value : number
, scaling : number
?
)
Insert a new point, or change an existing one, if a point in time already exists.
remove_point_at(self, time : integer
)
Removes a point at the given time. Point must exist.
Local Structs
EnvelopePoint
Single point within a pattern track automation envelope.
Properties
time : integer
Automation point's time in pattern lines in Range: (1 - NUM_LINES_IN_PATTERN).
value : number
Automation point value in Range: (0 - 1.0)
scaling : number
Automation point scaling. Used in 'lines' playback mode only - 0.0 is linear.
renoise.Sample
- Constants
- Properties
- is_slice_alias :
boolean
- slice_markers :
integer
[] - slice_markers_observable :
renoise.Document.ObservableList
- name :
string
- name_observable :
renoise.Document.Observable
- panning :
number
- panning_observable :
renoise.Document.Observable
- volume :
number
- volume_observable :
renoise.Document.Observable
- transpose :
integer
- transpose_observable :
renoise.Document.Observable
- fine_tune :
integer
- fine_tune_observable :
renoise.Document.Observable
- beat_sync_enabled :
boolean
- beat_sync_enabled_observable :
renoise.Document.Observable
- beat_sync_lines :
integer
- beat_sync_lines_observable :
renoise.Document.Observable
- beat_sync_mode :
renoise.Sample.BeatSyncMode
- beat_sync_mode_observable :
renoise.Document.Observable
- interpolation_mode :
renoise.Sample.InterpolationMode
- interpolation_mode_observable :
renoise.Document.Observable
- oversample_enabled :
boolean
- oversample_enabled_observable :
renoise.Document.Observable
- new_note_action :
renoise.Sample.NewNoteActionMode
- new_note_action_observable :
renoise.Document.Observable
- oneshot :
boolean
- oneshot_observable :
renoise.Document.Observable
- mute_group :
integer
- mute_group_observable :
renoise.Document.Observable
- autoseek :
boolean
- autoseek_observable :
renoise.Document.Observable
- autofade :
boolean
- autofade_observable :
renoise.Document.Observable
- loop_mode :
renoise.Sample.LoopMode
- loop_mode_observable :
renoise.Document.Observable
- loop_release :
boolean
- loop_release_observable :
renoise.Document.Observable
- loop_start :
integer
- loop_start_observable :
renoise.Document.Observable
- loop_end :
integer
- loop_end_observable :
renoise.Document.Observable
- modulation_set_index :
integer
- modulation_set_index_observable :
renoise.Document.Observable
- device_chain_index :
integer
- device_chain_index_observable :
renoise.Document.Observable
- sample_buffer :
renoise.SampleBuffer
- sample_buffer_observable :
renoise.Document.Observable
- sample_mapping :
renoise.SampleMapping
- is_slice_alias :
- Functions
Constants
InterpolationMode
{ INTERPOLATE_NONE: integer = 1, INTERPOLATE_LINEAR: integer = 2, INTERPOLATE_CUBIC: integer = 3, INTERPOLATE_SINC: integer = 4, }
LoopMode
{ LOOP_MODE_OFF: integer = 1, LOOP_MODE_FORWARD: integer = 2, LOOP_MODE_REVERSE: integer = 3, LOOP_MODE_PING_PONG: integer = 4, }
BeatSyncMode
{ BEAT_SYNC_REPITCH: integer = 1, BEAT_SYNC_PERCUSSION: integer = 2, BEAT_SYNC_TEXTURE: integer = 3, }
NewNoteActionMode
{ NEW_NOTE_ACTION_NOTE_CUT: integer = 1, NEW_NOTE_ACTION_NOTE_OFF: integer = 2, NEW_NOTE_ACTION_SUSTAIN: integer = 3, }
Properties
is_slice_alias : boolean
READ-ONLY True, when the sample slot is an alias to a sliced master sample. Such sample slots are read-only and automatically managed with the master samples slice list.
slice_markers : integer
[]
Read/write access to the slice marker list of a sample. When new markers are set or existing ones unset, existing 0S effects or notes to existing slices will NOT be remapped (unlike its done with the insert/remove/move_slice_marker functions). See function insert_slice_marker for info about marker limitations and preconditions.
slice_markers_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
name : string
Name.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
panning : number
Range: (0.0 - 1.0)
panning_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume : number
Range: (0.0 - 4.0)
volume_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
transpose : integer
Range: (-120 - 120)
transpose_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
fine_tune : integer
Range: (-127 - 127)
fine_tune_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
beat_sync_enabled : boolean
Beat sync.
beat_sync_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
beat_sync_lines : integer
Range: (1 - 512)
beat_sync_lines_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
beat_sync_mode : renoise.Sample.BeatSyncMode
beat_sync_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
interpolation_mode : renoise.Sample.InterpolationMode
Interpolation, new note action, oneshot, mute_group, autoseek, autofade.
interpolation_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
oversample_enabled : boolean
oversample_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
new_note_action : renoise.Sample.NewNoteActionMode
new_note_action_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
oneshot : boolean
oneshot_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mute_group : integer
Range: (0 - 15) where 0 means no group
mute_group_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
autoseek : boolean
autoseek_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
autofade : boolean
autofade_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_mode : renoise.Sample.LoopMode
Loops.
loop_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_release : boolean
loop_release_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_start : integer
Range: (1 - num_sample_frames)
loop_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_end : integer
Range: (1 - num_sample_frames)
loop_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
modulation_set_index : integer
The linked modulation set. 0 when disable, else a valid index for the renoise.Instrument.sample_modulation_sets table
modulation_set_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
device_chain_index : integer
The linked instrument device chain. 0 when disable, else a valid index for the renoise.Instrument.sample_device_chain table
device_chain_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sample_buffer : renoise.SampleBuffer
READ-ONLY
sample_buffer_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sample_mapping : renoise.SampleMapping
READ-ONLY Keyboard Note/velocity mapping
Functions
clear(self)
Reset, clear all sample settings and sample data.
copy_from(self, sample : renoise.Sample
)
Copy all settings, including sample data from another sample.
insert_slice_marker(self, marker_sample_pos : integer
)
Insert a new slice marker at the given sample position. Only samples in the first sample slot may use slices. Creating slices will automatically create sample aliases in the following slots: read-only sample slots that play the sample slice and are mapped to notes. Sliced sample lists can not be modified manually then. To update such aliases, modify the slice marker list instead. Existing 0S effects or notes will be updated to ensure that the old slices are played back just as before.
delete_slice_marker(self, marker_sample_pos : integer
)
Delete an existing slice marker. marker_sample_pos must point to an existing marker. See also property 'samples[].slice_markers'. Existing 0S effects or notes will be updated to ensure that the old slices are played back just as before.
move_slice_marker(self, old_marker_pos : integer
, new_marker_pos : integer
)
Change the sample position of an existing slice marker. see also property 'samples[].slice_markers'. When moving a marker behind or before an existing other marker, existing 0S effects or notes will automatically be updated to ensure that the old slices are played back just as before.
renoise.SampleAhdrsModulationDevice
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - attack :
renoise.DeviceParameter
- hold :
renoise.DeviceParameter
- duration :
renoise.DeviceParameter
- sustain :
renoise.DeviceParameter
- release :
renoise.DeviceParameter
- name :
- Functions
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
attack : renoise.DeviceParameter
with range (0-1)
hold : renoise.DeviceParameter
with range (0-1)
duration : renoise.DeviceParameter
with range (0-1)
sustain : renoise.DeviceParameter
with range (0-1)
release : renoise.DeviceParameter
with range (0-1)
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleBuffer
NOTE: All properties are invalid when no sample data is present, 'has_sample_data' returns false:
- Constants
- Properties
- has_sample_data :
boolean
- read_only :
boolean
- sample_rate :
integer
- bit_depth :
integer
- number_of_channels :
integer
- number_of_frames :
integer
- display_start :
integer
- display_start_observable :
renoise.Document.Observable
- display_length :
integer
- display_length_observable :
renoise.Document.Observable
- display_range :
integer
[] - display_range_observable :
renoise.Document.Observable
- vertical_zoom_factor :
number
- vertical_zoom_factor_observable :
renoise.Document.Observable
- selection_start :
integer
- selection_start_observable :
renoise.Document.Observable
- selection_end :
integer
- selection_end_observable :
renoise.Document.Observable
- selection_range :
integer
[] - selection_range_observable :
renoise.Document.Observable
- selected_channel :
renoise.SampleBuffer.Channel
- selected_channel_observable :
renoise.Document.Observable
- has_sample_data :
- Functions
- create_sample_data(self, sample_rate :
integer
, bit_depth :integer
, num_channels :integer
, num_frames :integer
) - delete_sample_data(self)
- sample_data(self, channel_index :
integer
, frame_index :integer
) - set_sample_data(self, channel_index :
integer
, frame_index :integer
, sample_value :integer
) - prepare_sample_data_changes(self)
- finalize_sample_data_changes(self)
- load_from(self, filename :
string
) - save_as(self, filename :
string
, format :SampleBuffer.ExportType
)
- create_sample_data(self, sample_rate :
- Local Aliases
Constants
Channel
{ CHANNEL_LEFT: integer = 1, CHANNEL_RIGHT: integer = 2, CHANNEL_LEFT_AND_RIGHT: integer = 3, }
Properties
has_sample_data : boolean
READ-ONLY Check this before accessing properties
read_only : boolean
READ-ONLY True, when the sample buffer can only be read, but not be modified. true for sample aliases of sliced samples. To modify such sample buffers, modify the sliced master sample buffer instead.
sample_rate : integer
READ-ONLY The current sample rate in Hz, like 44100.
bit_depth : integer
READ-ONLY The current bit depth, like 32, 16, 8.
number_of_channels : integer
READ-ONLY The integer of sample channels (1 or 2)
number_of_frames : integer
READ-ONLY The sample frame count (integer of samples per channel)
display_start : integer
Range: (1 - number_of_frames)
display_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
display_length : integer
Range: (1 - number_of_frames)
display_length_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
display_range : integer
[]
Range: (1 - number_of_frames)
display_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
vertical_zoom_factor : number
Range(0.0-1.0)
vertical_zoom_factor_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_start : integer
Range: (1 - number_of_frames)
selection_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_end : integer
Range: (1 - number_of_frames)
selection_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selection_range : integer
[]
Range: (1 - number_of_frames)
selection_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_channel : renoise.SampleBuffer.Channel
The selected channel.
selected_channel_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
create_sample_data(self, sample_rate : integer
, bit_depth : integer
, num_channels : integer
, num_frames : integer
)
->
success : boolean
Create new sample data with the given rate, bit-depth, channel and frame count. Will trash existing sample data. Initial buffer is all zero. Will only return false when memory allocation fails (you're running out of memory). All other errors are fired as usual.
delete_sample_data(self)
Delete existing sample data.
sample_data(self, channel_index : integer
, frame_index : integer
)
->
values : number
[]
Read access to samples in a sample data buffer.
set_sample_data(self, channel_index : integer
, frame_index : integer
, sample_value : integer
)
Write access to samples in a sample data buffer. New samples values must be within [-1, 1] and will be clipped automatically. Sample buffers may be read-only (see property 'read_only'). Attempts to write on such buffers will result into errors. IMPORTANT: before modifying buffers, call 'prepare_sample_data_changes'. When you are done, call 'finalize_sample_data_changes' to generate undo/redo data for your changes and update sample overview caches!
prepare_sample_data_changes(self)
To be called once BEFORE sample data gets manipulated via 'set_sample_data'. This will prepare undo/redo data for the whole sample. See also 'finalize_sample_data_changes'.
finalize_sample_data_changes(self)
To be called once AFTER the sample data is manipulated via 'set_sample_data'. This will create undo/redo data for the whole sample, and also update the sample view caches for the sample. The reason this isn't automatically invoked is to avoid performance overhead when changing sample data 'sample by sample'. Don't forget to call this after any data changes, or changes may not be visible in the GUI and can not be un/redone!
load_from(self, filename : string
)
->
success : boolean
Load sample data from a file. Files can be any audio format Renoise supports. Possible errors are shown to the user, otherwise success is returned.
save_as(self, filename : string
, format : SampleBuffer.ExportType
)
->
success : boolean
Export sample data to a file. Possible errors are shown to the user, otherwise success is returned.
format: | "wav" | "flac"
Local Aliases
SampleBuffer.ExportType
"flac"
| "wav"
SampleBuffer.ExportType: | "wav" | "flac"
renoise.SampleDeviceChain
- Properties
- name :
string
- name_observable :
renoise.Document.Observable
- available_devices :
string
[] - available_device_infos :
AudioDeviceInfo
[] - devices :
renoise.AudioDevice
[] - devices_observable :
renoise.Document.ObservableList
- available_output_routings :
string
[] - output_routing :
string
- output_routing_observable :
renoise.Document.Observable
- name :
- Functions
- Local Structs
- AudioDeviceInfo
Properties
name : string
Name of the audio effect chain.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
available_devices : string
[]
READ-ONLY Allowed, available devices for 'insert_device_at'.
available_device_infos : AudioDeviceInfo
[]
READ-ONLY Returns a list of tables containing more information about the devices.
devices : renoise.AudioDevice
[]
READ-ONLY Device access.
devices_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
available_output_routings : string
[]
READ-ONLY Output routing.
output_routing : string
One of 'available_output_routings'
output_routing_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
insert_device_at(self, device_path : string
, index : integer
)
->
new_device : renoise.AudioDevice
Insert a new device at the given position. "device_path" must be an available device See: renoise.SampleDeviceChain.available_devices
delete_device_at(self, index : integer
)
Delete an existing device from a chain. The mixer device at index 1 can not be deleted.
swap_devices_at(self, index1 : integer
, index2 : integer
)
Swap the positions of two devices in the device chain. The mixer device at index 1 can not be swapped or moved.
device(self, index : integer
)
Access to a single device in the chain.
Local Structs
AudioDeviceInfo
Audio device info
Properties
path : string
The device's path used by
renoise.Track:insert_device_at
name : string
The device's name
short_name : string
The device's name as displayed in shortened lists
favorite_name : string
The device's name as displayed in favorites
is_favorite : boolean
true if the device is a favorite
is_bridged : boolean
true if the device is a bridged plugin
renoise.SampleEnvelopeModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - external_editor_visible :
boolean
- play_mode :
renoise.SampleEnvelopeModulationDevice.PlayMode
- play_mode_observable :
renoise.Document.Observable
- length :
integer
- length_observable :
renoise.Document.Observable
- loop_mode :
renoise.SampleEnvelopeModulationDevice.LoopMode
- loop_mode_observable :
renoise.Document.Observable
- loop_start :
integer
- loop_start_observable :
renoise.Document.Observable
- loop_end :
integer
- loop_end_observable :
renoise.Document.Observable
- sustain_enabled :
boolean
- sustain_enabled_observable :
renoise.Document.Observable
- sustain_position :
integer
- sustain_position_observable :
renoise.Document.Observable
- fade_amount :
integer
- fade_amount_observable :
renoise.Document.Observable
- points :
SampleEnvelopeModulationDevice.Point
[] - points_observable :
renoise.Document.ObservableList
- name :
- Functions
- parameter(self, index :
integer
) - init(self)
- copy_from(self, other_device :
renoise.SampleEnvelopeModulationDevice
) - clear_points(self)
- clear_points_in_range(self, from_time :
integer
, to_time :integer
) - copy_points_from(self, other_device :
renoise.SampleEnvelopeModulationDevice
) - has_point_at(self, time :
integer
) - add_point_at(self, time :
integer
, value :number
, scaling :number``?
) - remove_point_at(self, time :
integer
)
- parameter(self, index :
- Local Structs
- SampleEnvelopeModulationDevice.Point
Constants
PlayMode
{ PLAYMODE_POINTS: integer = 1, PLAYMODE_LINES: integer = 2, PLAYMODE_CURVES: integer = 3, }
LoopMode
{ LOOP_MODE_OFF: integer = 1, LOOP_MODE_FORWARD: integer = 2, LOOP_MODE_REVERSE: integer = 3, LOOP_MODE_PING_PONG: integer = 4, }
MIN_NUMBER_OF_POINTS : integer
MAX_NUMBER_OF_POINTS : integer
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
external_editor_visible : boolean
External editor visibility. set to true to show the editor, false to close it
play_mode : renoise.SampleEnvelopeModulationDevice.PlayMode
Play mode (interpolation mode).
play_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
length : integer
Range: (6 - 1000)
length_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_mode : renoise.SampleEnvelopeModulationDevice.LoopMode
Loop.
loop_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_start : integer
Range: (1 - envelope.length)
loop_start_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_end : integer
Range: (1 - envelope.length)
loop_end_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sustain_enabled : boolean
Sustain.
sustain_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sustain_position : integer
Range: (1 - envelope.length)
sustain_position_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
fade_amount : integer
Range: (0 - 4095)
fade_amount_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
points : SampleEnvelopeModulationDevice.Point
[]
Get all points of the envelope. When setting a new list of points, items may be unsorted by time, but there may not be multiple points for the same time. Returns a copy of the list, so changing
points[1].value
will not do anything. Instead, change them viapoints = { something }
instead.
points_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
init(self)
Reset the envelope back to its default initial state.
copy_from(self, other_device : renoise.SampleEnvelopeModulationDevice
)
Copy all properties from another SampleEnvelopeModulation object.
clear_points(self)
Remove all points from the envelope.
clear_points_in_range(self, from_time : integer
, to_time : integer
)
Remove points in the given [from, to) time range from the envelope.
copy_points_from(self, other_device : renoise.SampleEnvelopeModulationDevice
)
Copy all points from another SampleEnvelopeModulation object.
has_point_at(self, time : integer
)
->
boolean
Test if a point exists at the given time.
add_point_at(self, time : integer
, value : number
, scaling : number
?
)
Add a new point value (or replace any existing value) at time.
remove_point_at(self, time : integer
)
Removes a point at the given time. Point must exist.
Local Structs
SampleEnvelopeModulationDevice.Point
Properties
time : integer
Range: (1 - envelope.length)
value : number
Range: (0.0 - 1.0)
scaling : number
Range: (-1.0 - 1.0)
renoise.SampleFaderModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - scaling :
renoise.SampleFaderModulationDevice.ScalingType
- scaling_observable :
renoise.Document.Observable
- from :
renoise.DeviceParameter
- to :
renoise.DeviceParameter
- duration :
renoise.DeviceParameter
- delay :
renoise.DeviceParameter
- name :
- Functions
Constants
ScalingType
{ SCALING_LOG_FAST: integer = 1, SCALING_LOG_SLOW: integer = 2, SCALING_LINEAR: integer = 3, SCALING_EXP_SLOW: integer = 4, SCALING_EXP_FAST: integer = 5, }
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
scaling : renoise.SampleFaderModulationDevice.ScalingType
Scaling mode.
scaling_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
from : renoise.DeviceParameter
Start value.
to : renoise.DeviceParameter
Target value.
duration : renoise.DeviceParameter
Duration.
delay : renoise.DeviceParameter
Delay.
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleKeyTrackingModulationDevice
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - min :
renoise.DeviceParameter
- max :
renoise.DeviceParameter
- name :
- Functions
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
min : renoise.DeviceParameter
with range (0-119)
max : renoise.DeviceParameter
with range (0-119)
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleLfoModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - mode :
renoise.SampleLfoModulationDevice.Mode
- phase :
renoise.DeviceParameter
- frequency :
renoise.DeviceParameter
- amount :
renoise.DeviceParameter
- delay :
renoise.DeviceParameter
- name :
- Functions
Constants
Mode
{ MODE_SIN: integer = 1, MODE_SAW: integer = 2, MODE_PULSE: integer = 3, MODE_RANDOM: integer = 4, }
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
mode : renoise.SampleLfoModulationDevice.Mode
LFO mode.
phase : renoise.DeviceParameter
with range (0-360)
frequency : renoise.DeviceParameter
with range (0-1)
amount : renoise.DeviceParameter
with range (0-1)
delay : renoise.DeviceParameter
Delay.
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleMapping
General remarks: Sample mappings of sliced samples are read-only: can not be modified. See
sample_mappings[].read_only
- Properties
- read_only :
boolean
- sample :
renoise.Sample
- layer :
renoise.Instrument.Layer
- layer_observable :
renoise.Document.Observable
- map_velocity_to_volume :
boolean
- map_velocity_to_volume_observable :
renoise.Document.Observable
- map_key_to_pitch :
boolean
- map_key_to_pitch_observable :
renoise.Document.Observable
- base_note :
integer
- base_note_observable :
renoise.Document.Observable
- note_range :
integer
[] - note_range_observable :
renoise.Document.Observable
- velocity_range :
integer
[] - velocity_range_observable :
renoise.Document.Observable
- read_only :
Properties
read_only : boolean
READ-ONLY True for sliced instruments. No sample mapping properties are allowed to be modified, but can be read.
sample : renoise.Sample
Linked sample.
layer : renoise.Instrument.Layer
Mapping's layer (triggered via Note-Ons or Note-Offs?).
layer_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
map_velocity_to_volume : boolean
Mappings velocity->volume and key->pitch options.
map_velocity_to_volume_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
map_key_to_pitch : boolean
map_key_to_pitch_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
base_note : integer
Range: (0-119, c-4=48)]
base_note_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
note_range : integer
[]
Range: (0 - 119) where C-4 is 48
note_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
velocity_range : integer
[]
Range: (0 - 127)
velocity_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
renoise.SampleModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[]
- name :
- Functions
Constants
TargetType
{ TARGET_VOLUME: integer = 1, TARGET_PANNING: integer = 2, TARGET_PITCH: integer = 3, TARGET_CUTOFF: integer = 4, TARGET_RESONANCE: integer = 5, TARGET_DRIVE: integer = 6, }
OperatorType
{ OPERATOR_ADD: integer = 1, OPERATOR_SUB: integer = 2, OPERATOR_MUL: integer = 3, OPERATOR_DIV: integer = 4, }
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleModulationSet
- Properties
- name :
string
- name_observable :
renoise.Document.Observable
- volume_input :
renoise.DeviceParameter
- panning_input :
renoise.DeviceParameter
- pitch_input :
renoise.DeviceParameter
- cutoff_input :
renoise.DeviceParameter
- resonance_input :
renoise.DeviceParameter
- drive_input :
renoise.DeviceParameter
- pitch_range :
integer
- pitch_range_observable :
renoise.Document.Observable
- available_devices :
string
[] - devices :
renoise.SampleModulationDevice
[] - devices_observable :
renoise.Document.ObservableList
- filter_version :
1
|2
|3
- filter_version_observable :
renoise.Document.Observable
- available_filter_types :
FilterTypes
[] - filter_type :
FilterTypes
- filter_type_observable :
renoise.Document.Observable
- name :
- Functions
- Local Aliases
Properties
name : string
Name of the modulation set.
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume_input : renoise.DeviceParameter
Input value for the volume domain
panning_input : renoise.DeviceParameter
Input value for the panning domain
pitch_input : renoise.DeviceParameter
Input value for the pitch domain
cutoff_input : renoise.DeviceParameter
Input value for the cutoff domain
resonance_input : renoise.DeviceParameter
Input value for the resonance domain
drive_input : renoise.DeviceParameter
Input value for the drive domain
pitch_range : integer
Range: (1 - 96)
pitch_range_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
available_devices : string
[]
READ-ONLY All available devices, to be used in 'insert_device_at'.
devices : renoise.SampleModulationDevice
[]
READ-ONLY Device list access.
devices_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
filter_version : 1
| 2
| 3
READ-ONLY Filter version, 3 is the latest.
filter_version_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
available_filter_types : FilterTypes
[]
READ-ONLY List of available filter types depending on the filter_version.
filter_type : FilterTypes
The type of the filter selected for the modulation set. Songs made with previous versions of Renoise may use old filter types.
filter_type_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
init(self)
Reset all chain back to default initial state. Removing all devices too.
copy_from(self, other_set : renoise.SampleModulationSet
)
Copy all devices from another SampleModulationSet object.
insert_device_at(self, device_path : string
, target_type : renoise.SampleModulationDevice.TargetType
, index : integer
)
->
new_sample_modulation_device : renoise.SampleModulationDevice
Insert a new device at the given position. "device_path" must be one of renoise.song().instruments[].sample_modulation_sets[].available_devices.
delete_device_at(self, index : integer
)
Delete a device at the given index.
device(self, index : integer
)
->
renoise.SampleModulationDevice
Access a single device by index.
upgrade_filter_version(self)
Upgrade filter to the latest version. Tries to find a somewhat matching filter in the new version, but things quite likely won't sound the same.
Local Aliases
FilterTypes
FilterTypes1
| FilterTypes2
| FilterTypes3
-- Available filter types when filter_version = 3 -- Available filter types when filter_version = 3 -- Available filter types when filter_version = 2 -- Available filter types when filter_version = 1 FilterTypes: | "None" | "LP Clean" | "LP K35" | "LP Moog" | "LP Diode" | "HP Clean" | "HP K35" | "HP Moog" | "BP Clean" | "BP K35" | "BP Moog" | "BandPass" | "BandStop" | "Vowel" | "Comb" | "Decimator" | "Dist Shape" | "Dist Fold" | "AM Sine" | "AM Triangle" | "AM Saw" | "AM Pulse" | "None" | "LP 2x2 Pole" | "LP 2 Pole" | "LP Biquad" | "LP Moog" | "LP Single" | "HP 2x2 Pole" | "HP 2 Pole" | "HP Moog" | "Band Reject" | "Band Pass" | "EQ -15 dB" | "EQ -6 dB" | "EQ +6 dB" | "EQ +15 dB" | "EQ Peaking" | "Dist. Low" | "Dist. Mid" | "Dist. High" | "Dist." | "RingMod" | "None" | "LP -12 dB" | "LP -24 dB" | "LP -48 dB" | "Moog LP" | "Single Pole" | "HP -12 dB" | "HP -24 dB" | "Moog HP" | "Band Reject" | "Band Pass" | "EQ -15 dB" | "EQ -6 dB" | "EQ +6 dB" | "EQ +15 dB" | "Peaking EQ" | "Dist. Low" | "Dist. Mid" | "Dist. High" | "Dist." | "AMod"
FilterTypes1
"AMod"
| "Band Pass"
| "Band Reject"
| "Dist. High"
| "Dist. Low"
| "Dist. Mid"
| "Dist."
| "EQ +15 dB"
| "EQ +6 dB"
| "EQ -15 dB"
| "EQ -6 dB"
| "HP -12 dB"
| "HP -24 dB"
| "LP -12 dB"
| "LP -24 dB"
| "LP -48 dB"
| "Moog HP"
| "Moog LP"
| "None"
| "Peaking EQ"
| "Single Pole"
-- Available filter types when filter_version = 1 FilterTypes1: | "None" | "LP -12 dB" | "LP -24 dB" | "LP -48 dB" | "Moog LP" | "Single Pole" | "HP -12 dB" | "HP -24 dB" | "Moog HP" | "Band Reject" | "Band Pass" | "EQ -15 dB" | "EQ -6 dB" | "EQ +6 dB" | "EQ +15 dB" | "Peaking EQ" | "Dist. Low" | "Dist. Mid" | "Dist. High" | "Dist." | "AMod"
FilterTypes2
"Band Pass"
| "Band Reject"
| "Dist. High"
| "Dist. Low"
| "Dist. Mid"
| "Dist."
| "EQ +15 dB"
| "EQ +6 dB"
| "EQ -15 dB"
| "EQ -6 dB"
| "EQ Peaking"
| "HP 2 Pole"
| "HP 2x2 Pole"
| "HP Moog"
| "LP 2 Pole"
| "LP 2x2 Pole"
| "LP Biquad"
| "LP Moog"
| "LP Single"
| "None"
| "RingMod"
-- Available filter types when filter_version = 2 FilterTypes2: | "None" | "LP 2x2 Pole" | "LP 2 Pole" | "LP Biquad" | "LP Moog" | "LP Single" | "HP 2x2 Pole" | "HP 2 Pole" | "HP Moog" | "Band Reject" | "Band Pass" | "EQ -15 dB" | "EQ -6 dB" | "EQ +6 dB" | "EQ +15 dB" | "EQ Peaking" | "Dist. Low" | "Dist. Mid" | "Dist. High" | "Dist." | "RingMod"
FilterTypes3
"AM Pulse"
| "AM Saw"
| "AM Sine"
| "AM Triangle"
| "BP Clean"
| "BP K35"
| "BP Moog"
| "BandPass"
| "BandStop"
| "Comb"
| "Decimator"
| "Dist Fold"
| "Dist Shape"
| "HP Clean"
| "HP K35"
| "HP Moog"
| "LP Clean"
| "LP Diode"
| "LP K35"
| "LP Moog"
| "None"
| "Vowel"
-- Available filter types when filter_version = 3 FilterTypes3: | "None" | "LP Clean" | "LP K35" | "LP Moog" | "LP Diode" | "HP Clean" | "HP K35" | "HP Moog" | "BP Clean" | "BP K35" | "BP Moog" | "BandPass" | "BandStop" | "Vowel" | "Comb" | "Decimator" | "Dist Shape" | "Dist Fold" | "AM Sine" | "AM Triangle" | "AM Saw" | "AM Pulse"
renoise.SampleOperandModulationDevice
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - value :
renoise.DeviceParameter
- name :
- Functions
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
value : renoise.DeviceParameter
Operand value.
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.SampleStepperModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - external_editor_visible :
boolean
- play_mode :
renoise.SampleStepperModulationDevice.PlayMode
- play_mode_observable :
renoise.Document.Observable
- play_step :
integer
- play_step_observable :
renoise.Document.Observable
- length :
integer
- length_observable :
renoise.Document.Observable
- points :
SampleStepperModulationDevice.Point
[] - points_observable :
renoise.Document.ObservableList
- name :
- Functions
- parameter(self, index :
integer
) - init(self)
- copy_from(self, other_device :
renoise.SampleStepperModulationDevice
) - clear_points(self)
- clear_points_in_range(self, from_time :
integer
, to_time :integer
) - copy_points_from(self, other_device :
renoise.SampleStepperModulationDevice
) - has_point_at(self, time :
integer
) - add_point_at(self, time :
integer
, value :number
, scaling :number``?
) - remove_point_at(self, time :
integer
)
- parameter(self, index :
- Local Structs
- SampleStepperModulationDevice.Point
Constants
PlayMode
{ PLAYMODE_POINTS: integer = 1, PLAYMODE_LINES: integer = 2, PLAYMODE_CURVES: integer = 3, }
MIN_NUMBER_OF_POINTS : integer
MAX_NUMBER_OF_POINTS : integer
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
external_editor_visible : boolean
External editor visibility. set to true to show the editor, false to close it
play_mode : renoise.SampleStepperModulationDevice.PlayMode
Play mode (interpolation mode).
play_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
play_step : integer
Range: (-1 - 16)
play_step_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
length : integer
Range: (1 - 256)
length_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
points : SampleStepperModulationDevice.Point
[]
Get all points of the envelope. When setting a new list of points, items may be unsorted by time, but there may not be multiple points for the same time. Returns a copy of the list, so changing
points[1].value
will not do anything. Instead, change them viapoints = { something }
.
points_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
init(self)
Reset the envelope back to its default initial state.
copy_from(self, other_device : renoise.SampleStepperModulationDevice
)
Copy all properties from another SampleStepperModulation object.
clear_points(self)
Remove all points from the envelope.
clear_points_in_range(self, from_time : integer
, to_time : integer
)
Remove points in the given [from, to) time range from the envelope.
copy_points_from(self, other_device : renoise.SampleStepperModulationDevice
)
Copy all points from another SampleStepperModulation object.
has_point_at(self, time : integer
)
->
boolean
Test if a point exists at the given time.
add_point_at(self, time : integer
, value : number
, scaling : number
?
)
Add a new point value (or replace any existing value) at time.
remove_point_at(self, time : integer
)
Removes a point at the given time. Point must exist.
Local Structs
SampleStepperModulationDevice.Point
Properties
time : integer
Range: (1 - envelope.length)
value : number
Range: (0.0 - 1.0)
scaling : number
Range: (-1.0 - 1.0)
renoise.SampleVelocityTrackingModulationDevice
- Constants
- Properties
- name :
string
- short_name :
string
- display_name :
string
- display_name_observable :
renoise.Document.Observable
- enabled :
boolean
- enabled_observable :
renoise.Document.Observable
- is_active :
boolean
- is_active_observable :
renoise.Document.Observable
- is_maximized :
boolean
- is_maximized_observable :
renoise.Document.Observable
- target :
renoise.SampleModulationDevice.TargetType
- operator :
renoise.SampleModulationDevice.OperatorType
- operator_observable :
renoise.Document.Observable
- bipolar :
boolean
- bipolar_observable :
renoise.Document.Observable
- tempo_sync_switching_allowed :
boolean
- tempo_synced :
boolean
- tempo_synced_observable :
renoise.Document.Observable
- is_active_parameter :
renoise.DeviceParameter
- parameters :
renoise.DeviceParameter
[] - mode :
renoise.SampleVelocityTrackingModulationDevice.Mode
- mode_observable :
renoise.Document.Observable
- min :
renoise.DeviceParameter
- max :
renoise.DeviceParameter
- name :
- Functions
Constants
Mode
{ MODE_CLAMP: integer = 1, MODE_SCALE: integer = 2, }
Properties
name : string
READ-ONLY Fixed name of the device.
short_name : string
READ-ONLY
display_name : string
Configurable device display name.
display_name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
enabled : boolean
enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active : boolean
not active = bypassed
is_active_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_maximized : boolean
Maximize state in modulation chain.
is_maximized_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
target : renoise.SampleModulationDevice.TargetType
READ-ONLY Where the modulation gets applied (Volume, Pan, Pitch, Cutoff, Resonance).
operator : renoise.SampleModulationDevice.OperatorType
Modulation operator: how the device applies.
operator_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
bipolar : boolean
Modulation polarity: when bipolar, the device applies it's values in a -1 to 1 range, when unipolar in a 0 to 1 range.
bipolar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tempo_sync_switching_allowed : boolean
READ-ONLY When true, the device has one of more time parameters, which can be switched to operate in synced or unsynced mode. see also field tempo_synced.
tempo_synced : boolean
When true and the device supports sync switching the device operates in wall-clock (ms) instead of beat times. see also property 'tempo_sync_switching_allowed'
tempo_synced_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
is_active_parameter : renoise.DeviceParameter
READ-ONLY Generic access to all parameters of this device.
parameters : renoise.DeviceParameter
[]
READ-ONLY
mode : renoise.SampleVelocityTrackingModulationDevice.Mode
Mode.
mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
min : renoise.DeviceParameter
with range (0-127)
max : renoise.DeviceParameter
with range (0-127)
Functions
init(self)
Reset the device to its default state.
copy_from(self, other_device : renoise.SampleModulationDevice
)
Copy a device's state from another device. 'other_device' must be of the same type.
parameter(self, index : integer
)
Access to a single parameter by index. Use properties 'parameters' to iterate over all parameters and to query the parameter count.
renoise.ScriptingTool
The scripting tool interface allows your tool to interact with Renoise by injecting or creating menu entries and keybindings into Renoise; or by attaching it to some common tool related notifiers.
- Properties
- bundle_path :
string
- tool_finished_loading_observable :
renoise.Document.Observable
- tool_will_unload_observable :
renoise.Document.Observable
- app_became_active_observable :
renoise.Document.Observable
- app_resigned_active_observable :
renoise.Document.Observable
- app_idle_observable :
renoise.Document.Observable
- app_release_document_observable :
renoise.Document.Observable
- app_new_document_observable :
renoise.Document.Observable
- app_saved_document_observable :
renoise.Document.Observable
- preferences :
renoise.Document.DocumentNode
- bundle_path :
- Functions
- has_menu_entry(self, entry_name :
string
) - add_menu_entry(self, entry :
ToolMenuEntry
) - remove_menu_entry(self, entry_name :
string
) - has_keybinding(self, keybinding_name :
string
) - add_keybinding(self, keybinding :
ToolKeybindingEntry
) - remove_keybinding(self, keybinding_name :
string
) - has_midi_mapping(self, midi_mapping_name :
string
) - add_midi_mapping(self, midi_mapping :
ToolMidiMappingEntry
) - remove_midi_mapping(self, midi_mapping_name :
string
) - has_file_import_hook(self, category :
FileHookCategory
, extensions_table :string
[]) - add_file_import_hook(self, file_import_hook :
ToolFileImportHook
) - remove_file_import_hook(self, category :
FileHookCategory
, extensions_table :string
[]) - has_timer(self, timer :
TimerFunction
) - add_timer(self, timer :
TimerFunction
, interval_in_ms :number
) - remove_timer(self, timer :
TimerFunction
)
- has_menu_entry(self, entry_name :
- Local Structs
- ToolFileImportHook
- ToolKeybindingEntry
- ToolMenuEntry
- ToolMidiMappingEntry
Properties
bundle_path : string
READ_ONLY Full absolute path and name to your tool's bundle directory.
tool_finished_loading_observable : renoise.Document.Observable
Invoked when the tool finished loading/initializing and no errors happened. When the tool has preferences, they are loaded here as well when the notification fires, but 'renoise.song()' may not yet be available.
See also 'renoise.tool().app_new_document_observable'.
tool_will_unload_observable : renoise.Document.Observable
Invoked right before a tool gets unloaded: either because it got disabled, reloaded or the application exists. You can cleanup resources or connections to other devices here if necessary.
app_became_active_observable : renoise.Document.Observable
Invoked as soon as the application becomes the foreground window. For example, when you ATL-TAB to it, or activate it with the mouse from another app to Renoise.
app_resigned_active_observable : renoise.Document.Observable
Invoked as soon as the application looses focus and another app becomes the foreground window.
app_idle_observable : renoise.Document.Observable
Invoked periodically in the background, more often when the work load is low, less often when Renoise's work load is high. The exact interval is undefined and can not be relied on, but will be around 10 times per sec. You can do stuff in the background without blocking the application here. Be gentle and don't do CPU heavy stuff please!
app_release_document_observable : renoise.Document.Observable
Invoked each time before a new document gets created or loaded: this is the last time renoise.song() still points to the old song before a new one arrives. You can explicitly release notifiers to the old document here, or do your own housekeeping. Also called right before the application exits.
app_new_document_observable : renoise.Document.Observable
Invoked each time a new document (song) is created or loaded. In other words: each time the result of renoise.song() is changed. Also called when the script gets reloaded (only happens with the auto_reload debugging tools), in order to connect the new script instance to the already running document.
app_saved_document_observable : renoise.Document.Observable
Invoked each time the app's document (song) is successfully saved. renoise.song().file_name will point to the filename that it was saved to.
preferences : renoise.Document.DocumentNode
Get or set an optional renoise.Document.DocumentNode object, which will be used as set of persistent "options" or preferences for your tool. By default nil. When set, the assigned document object will automatically be loaded and saved by Renoise, to retain the tools state. The preference XML file is saved/loaded within the tool bundle as "com.example.your_tool.xrnx/preferences.xml".
examples:
-- create a document via "Document.create" my_options = renoise.Document.create("ScriptingToolPreferences") { some_option = true, some_value = "string_value" } -- or create a document by inheriting from renoise.Document.DocumentNode class "ExampleToolPreferences"(renoise.Document.DocumentNode) function ExampleToolPreferences:__init() renoise.Document.DocumentNode.__init(self) self:add_property("some_option", true) self:add_property("some_value", "string_value") end my_options = ExampleToolPreferences() -- values can be accessed (read, written) via my_options.some_option.value = false -- also notifiers can be added to listen to changes to the values -- done by you, or after new values got loaded or a view changed the value: my_options.some_option:add_notifier(function() end) -- and assign it: renoise.tool().preferences = my_options -- 'my_options' will be loaded/saved automatically with the tool now
Functions
has_menu_entry(self, entry_name : string
)
->
boolean
Returns true if the given entry already exists, otherwise false.
add_menu_entry(self, entry : ToolMenuEntry
)
Add a new menu entry.
remove_menu_entry(self, entry_name : string
)
Remove a previously added menu entry by specifying its full name.
has_keybinding(self, keybinding_name : string
)
->
boolean
Returns true when the given keybinging already exists, otherwise false.
add_keybinding(self, keybinding : ToolKeybindingEntry
)
Register key bindings somewhere in Renoise's existing set of bindings.
remove_keybinding(self, keybinding_name : string
)
Remove a previously added key binding by specifying its name and path.
has_midi_mapping(self, midi_mapping_name : string
)
->
boolean
Returns true when the given mapping already exists, otherwise false.
add_midi_mapping(self, midi_mapping : ToolMidiMappingEntry
)
Add a new midi_mapping entry.
remove_midi_mapping(self, midi_mapping_name : string
)
Remove a previously added midi mapping by specifying its name.
has_file_import_hook(self, category : FileHookCategory
, extensions_table : string
[])
->
boolean
Returns true when the given hook already exists, otherwise false.
category: | "song" | "instrument" | "effect chain" | "effect preset" | "modulation set" | "phrase" | "sample" | "theme"
add_file_import_hook(self, file_import_hook : ToolFileImportHook
)
Add a new file import hook as described above.
remove_file_import_hook(self, category : FileHookCategory
, extensions_table : string
[])
Remove a previously added file import hook by specifying its category and extension(s)
category: | "song" | "instrument" | "effect chain" | "effect preset" | "modulation set" | "phrase" | "sample" | "theme"
has_timer(self, timer : TimerFunction
)
->
boolean
Returns true when the given function or method was registered as a timer.
add_timer(self, timer : TimerFunction
, interval_in_ms : number
)
Register a timer function or table with a function and context (a method) that periodically gets called by the
app_idle_observable
for your tool.Modal dialogs will avoid that timers are called. To create a one-shot timer, simply call remove_timer at the end of your timer function.
interval_in_ms
must be > 0. The exact interval your function is called will vary a bit, depending on workload; e.g. when enough CPU time is available the rounding error will be around +/- 5 ms.
remove_timer(self, timer : TimerFunction
)
Remove a previously registered timer.
Local Structs
ToolFileImportHook
Add support for new filetypes in Renoise. Registered file types will show up in Renoise's disk browser and can also be loaded by drag and dropping the files onto the Renoise window. When adding hooks for files which Renoise already supports, your tool's import functions will override the internal import functions.
Always load the file into the currently selected component, like 'renoise.song().selected_track','selected_instrument','selected_sample'.
Preloading/prehearing sample files is not supported via tools.
Properties
category : FileHookCategory
In which disk browser category the file type shows up. One of
extensions : string
[]
A list of strings, file extensions, that will invoke your hook, like for example {"txt", "s_wave"}
invoke : (file_name : string
) ->
boolean
function that is called to do the import. return true when the import succeeded, else false.
ToolKeybindingEntry
Register tool key bindings somewhere in Renoise's existing set of bindings.
Please note: there's no way to define default keyboard shortcuts for your entries. Users manually have to bind them in the keyboard prefs pane. As soon as they do, they'll get saved just like any other key binding in Renoise.
Properties
name : string
The scope, name and category of the key binding use the form:
$scope:$topic_name:$binding_name
:
$scope
is where the shortcut will be applied, just like those in the categories list for the keyboard assignment preference pane. Your key binding will only fire, when the scope is currently focused, except it's the global scope one. Using an unavailable scope will not fire an error, instead it will render the binding useless. It will be listed and mappable, but never be invoked.
$topic_name
is useful when grouping entries in the key assignment pane. Use "tool" if you can't come up with something meaningful.
$binding_name
is the name of the binding.-Currently available scopes are:
+ "Global" + "Automation" + "Disk Browser" + "DSPs Chain" + "Instrument Box" + "Mixer" + "Pattern Editor" + "Pattern Matrix" + "Pattern Sequencer" + "Sample Editor" + "Sample FX Mixer" + "Sample Keyzones" + "Sample Modulation Matrix"
invoke : (repeated : boolean
)
A function that is called as soon as the mapped key is pressed. The callback parameter "repeated", indicates if its a virtual key repeat.
ToolMenuEntry
Defines a menu entry somewhere in Renoise's existing context menus or the global app menu. Insertion can be done during script initialization, but can also be done dynamically later on.
You can place your entries in any context menu or any window menu in Renoise. To do so, use one of the specified categories in its name:
+ "Window Menu" -- Renoise icon menu in the window caption on Windows/Linux + "Main Menu:XXX" (with XXX = ":File", ":Edit", ":View", ":Tools" or ":Help") -- Main menu + "Scripting Menu:XXX" (with XXX = ":File" or ":Tools") -- Scripting Editor & Terminal + "Disk Browser Directories" + "Disk Browser Files" + "Instrument Box" + "Pattern Sequencer" + "Pattern Editor" + "Pattern Matrix" + "Pattern Matrix Header" + "Phrase Editor" + "Phrase Mappings" + "Phrase Grid" + "Sample Navigator" + "Sample Editor" + "Sample Editor Ruler" + "Sample Editor Slice Markers" + "Sample List" + "Sample Mappings" + "Sample FX Mixer" + "Sample Modulation Matrix" + "Mixer" + "Master Spectrum" + "Track Automation" + "Track Automation List" + "DSP Chain" + "DSP Chain List" + "DSP Device" + "DSP Device Header" + "DSP Device Automation" + "Modulation Set" + "Modulation Set List" + "Tool Browser" + "Script File Browser" + "Script File Tabs"
Separating entries: To divide entries into groups (separate entries with a line), prepend one or more dashes to the name, like "--- Main Menu:Tools:My Tool Group Starts Here"
Properties
name : string
Name and 'path' of the entry as shown in the global menus or context menus to the user.
invoke : fun()
A function that is called as soon as the entry is clicked
active : () ->
boolean
A function that should return true or false. When returning false, the action will not be invoked and will be "greyed out" in menus. This function is always called before "invoke", and every time prior to a menu becoming visible.
selected : () ->
boolean
A function that should return true or false. When returning true, the entry will be marked as "this is a selected option"
ToolMidiMappingEntry
Extend Renoise's default MIDI mapping set, or add custom MIDI mappings for your tool.
A tool's MIDI mapping can be used just like the regular mappings in Renoise: Either by manually looking its up the mapping in the MIDI mapping list, then binding it to a MIDI message, or when your tool has a custom GUI, specifying the mapping via a control's
control.midi_mapping
property. Such controls will then get highlighted as soon as the MIDI mapping dialog is opened. Then, users simply click on the highlighted control to map MIDI messages.
Properties
name : string
The group, name of the midi mapping; as visible to the user.
The scope, name and category of the midi mapping use the form:
$topic_name:$optional_sub_topic_name:$name
:
$topic_name
and$optional_sub_topic_name
will create new groups in the list of MIDI mappings, as seen in Renoise's MIDI mapping dialog. If you can't come up with a meaningful string, use your tool's name as topic name.Existing global mappings from Renoise can be overridden. In this case the original mappings are no longer called, only your tool's mapping.
invoke : (message : renoise.ScriptingTool.MidiMessage
)
A function that is called to handle a bound MIDI message.
Local Aliases
FileHookCategory
"effect chain"
| "effect preset"
| "instrument"
| "modulation set"
| "phrase"
| "sample"
| "song"
| "theme"
FileHookCategory: | "song" | "instrument" | "effect chain" | "effect preset" | "modulation set" | "phrase" | "sample" | "theme"
TimerFunction
fun()
renoise.ScriptingTool.MidiMessage
MIDI message as passed to the
invoke
callback in tool midi_mappings.
Properties
int_value : integer
?
Range: (0S - 127) for abs values, Range: (-63 - 63) for relative values valid when
is_rel_value()
oris_abs_value()
returns true, else undefined
boolean_value : boolean
?
valid [true OR false] when
is_switch()
returns true, else undefined
Functions
is_trigger(self)
returns if action should be invoked
is_switch(self)
check if the boolean_value property is valid
is_rel_value(self)
->
boolean
check if the int_value property is valid
is_abs_value(self)
->
boolean
check if the int_value property is valid
renoise.Socket
Interfaces for built-in socket support for Lua scripts in Renoise.
Right now UDP and TCP protocols are supported. The class interfaces for UDP and TCP sockets behave exactly the same. That is, they don't depend on the protocol, so both are easily interchangeable when needed.
Constants
Protocol
{ PROTOCOL_TCP: integer = 1, PROTOCOL_UDP: integer = 2, }
Functions
create_server(server_address : string
, server_port : integer
, protocol : renoise.Socket.Protocol
?
)
->
renoise.Socket.SocketServer
?
, string
?
Creates a connected UPD or TCP server object. Use "localhost" to use your system's default network address. Protocol can be
renoise.Socket.PROTOCOL_TCP
orrenoise.Socket.PROTOCOL_UDP
(by default TCP). When instantiation and connection succeed, a valid server object is returned, otherwise "error" is set and the server object is nil. Using the create function with no server_address allows you to create a server which allows connections to any address (for example localhost and some IP)
create_client(server_address : string
, server_port : integer
, protocol : renoise.Socket.Protocol
?
, timeout : integer
?
)
->
client : renoise.Socket.SocketClient
?
, error : string
?
Create a connected UPD or TCP client.
protocol
can berenoise.Socket.PROTOCOL_TCP
orrenoise.Socket.PROTOCOL_UDP
(by default TCP).timeout
is the time in ms to wait until the connection is established (1000 ms by default). When instantiation and connection succeed, a valid client object is returned, otherwise "error" is set and the client object is nil
renoise.Socket.SocketBase
SocketBase is the base class for socket clients and servers. All SocketBase properties and functions are available for servers and clients.
Properties
is_open : boolean
READ-ONLY Returns true when the socket object is valid and connected. Sockets can manually be closed (see socket:close()). Client sockets can also actively be closed/refused by the server. In this case the client:receive() calls will fail and return an error.
local_address : string
READ-ONLY The socket's resolved local address (for example "127.0.0.1" when a socket is bound to "localhost")
local_port : integer
READ-ONLY The socket's local port number, as specified when instantiated.
Functions
close(self)
Closes the socket connection and releases all resources. This will make the socket useless, so any properties, calls to the socket will result in errors. Can be useful to explicitly release a connection without waiting for the dead object to be garbage collected, or if you want to actively refuse a connection.
renoise.Socket.SocketClient
A SocketClient can connect to other socket servers and send and receive data from them on request. Connections to a server can not change, they are specified when constructing a client. You can not reconnect a client; create a new client instance instead.
Socket clients in Renoise do block with timeouts to receive messages, and assume that you only expect a response from a server after having sent something to it (i.e.: GET HTTP). To constantly poll a connection to a server, for example in idle timers, specify a timeout of 0 in "receive(message, 0)". This will only check if there are any pending messages from the server and read them. If there are no pending messages it will not block or timeout.
Properties
is_open : boolean
READ-ONLY Returns true when the socket object is valid and connected. Sockets can manually be closed (see socket:close()). Client sockets can also actively be closed/refused by the server. In this case the client:receive() calls will fail and return an error.
local_address : string
READ-ONLY The socket's resolved local address (for example "127.0.0.1" when a socket is bound to "localhost")
local_port : integer
READ-ONLY The socket's local port number, as specified when instantiated.
peer_address : string
READ-ONLY Address of the socket's peer, the socket address this client is connected to.
peer_port : integer
READ-ONLY Port of the socket's peer, the socket this client is connected to.
Functions
close(self)
Closes the socket connection and releases all resources. This will make the socket useless, so any properties, calls to the socket will result in errors. Can be useful to explicitly release a connection without waiting for the dead object to be garbage collected, or if you want to actively refuse a connection.
send(self, message : string
)
->
success : boolean
, error : string
?
Send a message string (or OSC messages or bundles) to the connected server. When sending fails, "success" return value will be false and "error_message" is set, describing the error in a human readable format. NB: when using TCP instead of UDP as protocol for OSC messages, !no! SLIP encoding and no size prefixing of the passed OSC data will be done here. So, when necessary, do this manually by your own please.
receive(self, mode : SocketReceiveMode
, timeout_ms : number
)
->
success : boolean
?
, error : string
?
Receive a message string from the the connected server with the given timeout in milliseconds. Mode can be one of "*line", "*all" or a number > 0, like Lua's io.read. \param timeout can be 0, which is useful for receive("*all"). This will only check and read pending data from the sockets queue.
mode "*line": Will receive new data from the server or flush pending data that makes up a "line": a string that ends with a newline. remaining data is kept buffered for upcoming receive calls and any kind of newlines are supported. The returned line will not contain the newline characters.
mode "*all": Reads all pending data from the peer socket and also flushes internal buffers from previous receive line/byte calls (when present). This will NOT read the entire requested content, but only the current buffer that is queued for the local socket from the peer. To read an entire HTTP page or file you may have to call receive("*all") multiple times until you got all you expect to get.
mode "number > 0": Tries reading \param NumberOfBytes of data from the peer. Note that the timeout may be applied more than once, if more than one socket read is needed to receive the requested block.
When receiving fails or times-out, the returned message will be nil and error_message is set. The error message is "timeout" on timeouts, "disconnected" when the server actively refused/disconnected your client. Any other errors are system dependent, and should only be used for display purposes.
Once you get an error from receive, and this error is not a "timeout", the socket will already be closed and thus must be recreated in order to retry communication with the server. Any attempt to use a closed socket will fire a runtime error.
mode: | "*line" | "*all"
Local Aliases
SocketReceiveMode
integer
| "*all"
| "*line"
SocketReceiveMode: | "*line" | "*all"
renoise.Socket.SocketServer
A SocketServer handles one or more clients in the background, interacts only with callbacks from connected clients. This background polling can be start and stop on request.
The socket server interface in Renoise is asynchronous (callback based), which means server calls never block or wait, but are served in the background. As soon a connection is established or messages arrive, a set of specified callbacks are invoked to respond to messages.
Properties
is_open : boolean
READ-ONLY Returns true when the socket object is valid and connected. Sockets can manually be closed (see socket:close()). Client sockets can also actively be closed/refused by the server. In this case the client:receive() calls will fail and return an error.
local_address : string
READ-ONLY The socket's resolved local address (for example "127.0.0.1" when a socket is bound to "localhost")
local_port : integer
READ-ONLY The socket's local port number, as specified when instantiated.
is_running : boolean
READ-ONLY true while the server is running, else false.
Functions
close(self)
Closes the socket connection and releases all resources. This will make the socket useless, so any properties, calls to the socket will result in errors. Can be useful to explicitly release a connection without waiting for the dead object to be garbage collected, or if you want to actively refuse a connection.
run(self, notifier_table : NotifierClass
| NotifierTable
)
Start running the server by specifying a class or table which defines the callback functions for the server.
stop(self)
Stop a running server.
wait(self, timeout : number
)
Suspends the calling thread by the given timeout, and calls the server's callback methods as soon as something has happened in the server while waiting. Should be avoided whenever possible.
Local Structs
NotifierClass
Custom notifier class for
renoise.Socket.SocketServer:run
. Note: You must pass an instance of a class, like server_socket:run(MyNotifier())All callback properties are optional. So you can, for example, skip specifying "socket_accepted" if you have no use for this.
examples:
class "MyNotifier" function MyNotifier:__init() -- could pass a server ref or something else here, or simply do nothing end function MyNotifier:socket_error(error_message) -- do something with the error message end function MyNotifier:socket_accepted(socket) -- FOR TCP CONNECTIONS ONLY: do something with socket end function MyNotifier:socket_message(socket, message) -- do something with socket and message end
Properties
socket_error : (self : any
, error_message : string
)?
socket_accepted : (self : any
, socket : renoise.Socket.SocketClient
)?
socket_message : (self : any
, socket : renoise.Socket.SocketClient
, message : string
)?
NotifierTable
Notifier table for
renoise.Socket.SocketServer:run
.All callback properties are optional. So you can, for example, skip specifying "socket_accepted" if you have no use for this.
examples:
{ socket_error = function(error_message) -- do something with the error message end, socket_accepted = function(client) -- FOR TCP CONNECTIONS ONLY: do something with client end, socket_message = function(client, message) -- do something with client and message end }
Properties
socket_error : (error_message : string
)?
An error happened in the servers background thread.
socket_accepted : (client : renoise.Socket.SocketClient
)?
FOR TCP CONNECTIONS ONLY: called as soon as a new client connected to your server. The passed socket is a ready to use socket object, representing a connection to the new socket.
socket_message : (client : renoise.Socket.SocketClient
, message : string
)?
A message was received from a client: The passed socket is a ready to use connection for TCP connections. For UDP, a "dummy" socket is passed, which can only be used to query the peer address and port -> socket.port and socket.address
renoise.Song
Renoise's main document - the song.
- Constants
- Properties
- file_name :
string
- artist :
string
- artist_observable :
renoise.Document.Observable
- name :
string
- name_observable :
renoise.Document.Observable
- comments :
string
[] - comments_observable :
renoise.Document.ObservableList
- comments_assignment_observable :
renoise.Document.Observable
- show_comments_after_loading :
boolean
- show_comments_after_loading_observable :
renoise.Document.Observable
- tool_data :
string``?
- rendering :
boolean
- rendering_progress :
number
- transport :
renoise.Transport
- sequencer :
renoise.PatternSequencer
- pattern_iterator :
renoise.PatternIterator
- sequencer_track_count :
integer
- send_track_count :
integer
- instruments :
renoise.Instrument
[] - instruments_observable :
renoise.Document.ObservableList
- patterns :
renoise.Pattern
[] - patterns_observable :
renoise.Document.ObservableList
- tracks :
renoise.Track
[] - tracks_observable :
renoise.Document.ObservableList
- selected_instrument :
renoise.Instrument
- selected_instrument_observable :
renoise.Document.Observable
- selected_instrument_index :
integer
- selected_instrument_index_observable :
renoise.Document.Observable
- selected_phrase :
renoise.InstrumentPhrase
- selected_phrase_observable :
renoise.Document.Observable
- selected_phrase_index :
integer
- selected_sample :
renoise.Sample
- selected_sample_observable :
renoise.Document.Observable
- selected_sample_index :
integer
- selected_sample_modulation_set :
renoise.SampleModulationSet
- selected_sample_modulation_set_observable :
renoise.Document.Observable
- selected_sample_modulation_set_index :
integer
- selected_sample_device_chain :
renoise.SampleDeviceChain
- selected_sample_device_chain_observable :
renoise.Document.Observable
- selected_sample_device_chain_index :
integer
- selected_sample_device :
renoise.AudioDevice
- selected_sample_device_observable :
renoise.Document.Observable
- selected_sample_device_index :
integer
- selected_track :
renoise.Track
- selected_track_observable :
renoise.Document.Observable
- selected_track_index :
integer
- selected_track_index_observable :
renoise.Document.Observable
- selected_track_device :
renoise.AudioDevice
- selected_track_device_observable :
renoise.Document.Observable
- selected_track_device_index :
integer
- selected_device :
renoise.AudioDevice
- selected_device_observable :
renoise.Document.Observable
- selected_device_index :
integer
- selected_parameter :
renoise.DeviceParameter
- selected_parameter_observable :
renoise.Document.Observable
- selected_automation_parameter :
renoise.DeviceParameter
- selected_automation_parameter_observable :
renoise.Document.Observable
- selected_automation_device :
renoise.AudioDevice
- selected_automation_device_observable :
renoise.Document.Observable
- selected_pattern :
renoise.Pattern
- selected_pattern_observable :
renoise.Document.Observable
- selected_pattern_index :
integer
- selected_pattern_index_observable :
renoise.Document.Observable
- selected_pattern_track :
renoise.PatternTrack
- selected_pattern_track_observable :
renoise.Document.Observable
- selected_sequence_index :
integer
- selected_sequence_index_observable :
renoise.Document.Observable
- selected_line :
renoise.PatternLine
- selected_line_index :
integer
- selected_note_column :
renoise.NoteColumn
- selected_note_column_index :
integer
- selected_effect_column :
renoise.EffectColumn
- selected_effect_column_index :
integer
- selected_sub_column_type :
renoise.Song.SubColumnType
- selection_in_pattern :
PatternSelection``?
- selection_in_phrase :
PhraseSelection``?
- file_name :
- Functions
- can_undo(self)
- undo(self)
- can_redo(self)
- redo(self)
- describe_undo(self, description :
any
) - insert_track_at(self, index :
integer
) - delete_track_at(self, index :
integer
) - swap_tracks_at(self, index1 :
integer
, index2 :integer
) - track(self, index :
integer
) - select_previous_track(self)
- select_next_track(self)
- insert_group_at(self, index :
integer
) - add_track_to_group(self, track_index :
integer
, group_index :integer
) - remove_track_from_group(self, track_index :
integer
) - delete_group_at(self, group_index :
integer
) - insert_instrument_at(self, index :
integer
) - delete_instrument_at(self, index :
integer
) - swap_instruments_at(self, index1 :
integer
, index2 :integer
) - instrument(self, index :
integer
) - capture_instrument_from_pattern(self)
- capture_nearest_instrument_from_pattern(self)
- pattern(self, index :
integer
) - cancel_rendering(self)
- render(self, options :
RenderOptions
, filename :string
, rendering_done_callback : fun()) - load_midi_mappings(self, filename :
string
) - save_midi_mappings(self, filename :
string
) - clear_midi_mappings(self)
- Local Structs
- PatternSelection
- PhraseSelection
- RenderOptions
Constants
SubColumnType
{ SUB_COLUMN_NOTE: integer = 1, SUB_COLUMN_INSTRUMENT: integer = 2, SUB_COLUMN_VOLUME: integer = 3, SUB_COLUMN_PANNING: integer = 4, SUB_COLUMN_DELAY: integer = 5, SUB_COLUMN_SAMPLE_EFFECT_NUMBER: integer = 6, SUB_COLUMN_SAMPLE_EFFECT_AMOUNT: integer = 7, SUB_COLUMN_EFFECT_NUMBER: integer = 8, SUB_COLUMN_EFFECT_AMOUNT: integer = 9, }
MAX_NUMBER_OF_INSTRUMENTS : integer
Properties
file_name : string
READ-ONLY When the song is loaded from or saved to a file, the absolute path and name to the XRNS file, otherwise an empty string.
artist : string
Song Comments
artist_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
name : string
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
comments : string
[]
Note: All property tables of basic types in the API are temporary copies. In other words
comments = { "Hello", "World" }
will work,comments[1] = "Hello"; renoise.song().comments[2] = "World"
will not work.
comments_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
comments_assignment_observable : renoise.Document.Observable
READ-ONLY Notifier is called as soon as any paragraph in the comments change.
show_comments_after_loading : boolean
Set this to true to show the comments dialog after loading a song
show_comments_after_loading_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tool_data : string
?
Inject/fetch custom XRNX scripting tool data into the song. Can only be called from scripts that are running in Renoise scripting tool bundles; attempts to access the data from e.g. the scripting terminal will result in an error. Returns nil when no data is present.
Each tool gets it's own data slot in the song, which is resolved by the tool's bundle id, so this data is unique for every tool and persistent across tools with the same bundle id (but possibly different versions). If you want to store renoise.Document data in here, you can use the renoise.Document's 'to_string' and 'from_string' functions to serialize the data. Alternatively, write your own serializers for your custom data.
rendering : boolean
READ-ONLY True while rendering is in progress.
rendering_progress : number
Range: (0.0 - 1.0)
transport : renoise.Transport
READ-ONLY
sequencer : renoise.PatternSequencer
READ-ONLY
pattern_iterator : renoise.PatternIterator
READ-ONLY
sequencer_track_count : integer
READ-ONLY number of normal playback tracks (non-master or sends) in song.
send_track_count : integer
-READ-ONLY number of send tracks in song.
instruments : renoise.Instrument
[]
READ-ONLY Instrument, Pattern, and Track arrays
instruments_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
patterns : renoise.Pattern
[]
patterns_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
tracks : renoise.Track
[]
tracks_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
selected_instrument : renoise.Instrument
READ-ONLY Selected in the instrument box.
selected_instrument_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_instrument_index : integer
selected_instrument_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_phrase : renoise.InstrumentPhrase
READ-ONLY Currently selected phrase the instrument's phrase map piano view.
selected_phrase_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_phrase_index : integer
selected_sample : renoise.Sample
READ-ONLY Selected in the instrument's sample list. Only nil when no samples are present in the selected instrument.
selected_sample_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_sample_index : integer
selected_sample_modulation_set : renoise.SampleModulationSet
READ-ONLY Selected in the instrument's modulation view.
selected_sample_modulation_set_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_sample_modulation_set_index : integer
selected_sample_device_chain : renoise.SampleDeviceChain
READ-ONLY Selected in the instrument's effects view.
selected_sample_device_chain_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_sample_device_chain_index : integer
selected_sample_device : renoise.AudioDevice
READ-ONLY Selected in the sample effect mixer.
selected_sample_device_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_sample_device_index : integer
selected_track : renoise.Track
READ-ONLY Selected in the pattern editor or mixer.
selected_track_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_track_index : integer
selected_track_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_track_device : renoise.AudioDevice
READ-ONLY Selected in the track DSP chain editor.
selected_track_device_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_track_device_index : integer
selected_device : renoise.AudioDevice
selected_device_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_device_index : integer
selected_parameter : renoise.DeviceParameter
selected_parameter_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_automation_parameter : renoise.DeviceParameter
Selected parameter in the automation editor. When setting a new parameter, parameter must be automateable and must be one of the currently selected track device chain.
selected_automation_parameter_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_automation_device : renoise.AudioDevice
READ-ONLY parent device of 'selected_automation_parameter'. not settable.
selected_automation_device_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_pattern : renoise.Pattern
READ-ONLY The currently edited pattern.
selected_pattern_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_pattern_index : integer
READ-ONLY The currently edited pattern index.
selected_pattern_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_pattern_track : renoise.PatternTrack
READ-ONLY The currently edited pattern track object. and selected_track_observable for notifications.
selected_pattern_track_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_sequence_index : integer
The currently edited sequence position.
selected_sequence_index_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
selected_line : renoise.PatternLine
READ-ONLY The currently edited line in the edited pattern.
selected_line_index : integer
selected_note_column : renoise.NoteColumn
READ-ONLY The currently edited column in the selected line in the edited sequence/pattern. Nil when an effect column is selected.
selected_note_column_index : integer
selected_effect_column : renoise.EffectColumn
READ-ONLY The currently edited column in the selected line in the edited sequence/pattern. Nil when a note column is selected.
selected_effect_column_index : integer
selected_sub_column_type : renoise.Song.SubColumnType
READ-ONLY The currently edited sub column type within the selected note/effect column.
selection_in_pattern : PatternSelection
?
Read/write access to the selection in the pattern editor.
Line indexes are valid from 1 to patterns[].number_of_lines Track indexes are valid from 1 to #tracks Column indexes are valid from 1 to (tracks[].visible_note_columns + tracks[].visible_effect_columns)
When setting the selection, all members are optional. Combining them in various different ways will affect how specific the selection is. When
selection_in_pattern
returns nil or is set to nil, no selection is present.examples:
renoise.song().selection_in_pattern = {} --> clear renoise.song().selection_in_pattern = { start_line = 1, end_line = 4 } --> select line 1 to 4, first to last track renoise.song().selection_in_pattern = { start_line = 1, start_track = 1, end_line = 4, end_track = 1 } --> select line 1 to 4, in the first track only
selection_in_phrase : PhraseSelection
?
Same as
selection_in_pattern
but for the currently selected phrase (if any).
Functions
can_undo(self)
->
boolean
Test if something in the song can be undone.
undo(self)
Undo the last performed action. Will do nothing if nothing can be undone.
can_redo(self)
->
boolean
Test if something in the song can be redone.
redo(self)
Redo a previously undo action. Will do nothing if nothing can be redone.
describe_undo(self, description : any
)
When modifying the song, Renoise will automatically add descriptions for undo/redo by looking at what first changed (a track was inserted, a pattern line changed, and so on). When the song is changed from an action in a menu entry callback, the menu entry's label will automatically be used for the undo description. If those auto-generated names do not work for you, or you want to use something more descriptive, you can (!before changing anything in the song!) give your changes a custom undo description (like: "Generate Synth Sample")
insert_track_at(self, index : integer
)
Insert a new track at the given index. Inserting a track behind or at the Master Track's index will create a Send Track. Otherwise, a regular track is created.
delete_track_at(self, index : integer
)
Delete an existing track. The Master track can not be deleted, but all Sends can. Renoise needs at least one regular track to work, thus trying to delete all regular tracks will fire an error.
swap_tracks_at(self, index1 : integer
, index2 : integer
)
Swap the positions of two tracks. A Send can only be swapped with a Send track and a regular track can only be swapped with another regular track. The Master can not be swapped at all.
track(self, index : integer
)
Access to a single track by index. Use properties 'tracks' to iterate over all tracks and to query the track count.
select_previous_track(self)
Set the selected track to prev relative to the current track. Takes care of skipping over hidden tracks and wrapping around at the edges.
select_next_track(self)
Set the selected track to next relative to the current track. Takes care of skipping over hidden tracks and wrapping around at the edges.
insert_group_at(self, index : integer
)
Insert a new group track at the given index. Group tracks can only be inserted before the Master track.
add_track_to_group(self, track_index : integer
, group_index : integer
)
Add track at track_index to group at group_index by first moving it to the right spot to the left of the group track, and then adding it. If group_index is not a group track, a new group track will be created and both tracks will be added to it.
remove_track_from_group(self, track_index : integer
)
Removes track from its immediate parent group and places it outside it to the left. Can only be called for tracks that are actually part of a group.
delete_group_at(self, group_index : integer
)
Delete the group with the given index and all its member tracks. Index must be that of a group or a track that is a member of a group.
insert_instrument_at(self, index : integer
)
Insert a new instrument at the given index. This will remap all existing notes in all patterns, if needed, and also update all other instrument links in the song. Can't have more than MAX_NUMBER_OF_INSTRUMENTS in a song.
delete_instrument_at(self, index : integer
)
Delete an existing instrument at the given index. Renoise needs at least one instrument, thus trying to completely remove all instruments is not allowed. This will remap all existing notes in all patterns and update all other instrument links in the song.
swap_instruments_at(self, index1 : integer
, index2 : integer
)
Swap the position of two instruments. Will remap all existing notes in all patterns and update all other instrument links in the song.
instrument(self, index : integer
)
Access to a single instrument by index. Use properties 'instruments' to iterate over all instruments and to query the instrument count.
capture_instrument_from_pattern(self)
Captures the current instrument (selects the instrument) from the current note column at the current cursor pos. Changes the selected instrument accordingly, but does not return the result. When no instrument is present at the current cursor pos, nothing will be done.
capture_nearest_instrument_from_pattern(self)
Tries to captures the nearest instrument from the current pattern track, starting to look at the cursor pos, then advancing until an instrument is found. Changes the selected instrument accordingly, but does not return the result. When no instruments (notes) are present in the current pattern track, nothing will be done.
pattern(self, index : integer
)
Access to a single pattern by index. Use properties 'patterns' to iterate over all patterns and to query the pattern count.
cancel_rendering(self)
When rendering (see rendering, renoise.song().rendering_progress), the current render process is canceled. Otherwise, nothing is done.
render(self, options : RenderOptions
, filename : string
, rendering_done_callback : fun())
->
success : boolean
, error : string
Start rendering a section of the song or the whole song to a WAV file.
Rendering job will be done in the background and the call will return back immediately, but the Renoise GUI will be blocked during rendering. The passed
rendering_done_callback
function is called as soon as rendering is done, e.g. successfully completed.While rendering, the rendering status can be polled with the
song().rendering
andsong().rendering_progress
properties, for example, in idle notifier loops. If starting the rendering process fails (because of file IO errors for example), the render function will return false and the error message is set as the second return value. On success, only a singletrue
value is returned.To render only specific tracks or columns, mute the undesired tracks/columns before starting to render.
Parameter
file_name
must point to a valid, maybe already existing file. If it already exists, the file will be silently overwritten. The renderer will automatically add a ".wav" extension to the file_name, if missing.Parameter
rendering_done_callback
is ONLY called when rendering has succeeded. You can do something with the file you've passed to the renderer here, like for example loading the file into a sample buffer.
load_midi_mappings(self, filename : string
)
->
success : boolean
, error : string
Load all global MIDI mappings in the song into a XRNM file. Returns true when loading/saving succeeded, else false and the error message.
save_midi_mappings(self, filename : string
)
->
success : boolean
, error : string
Save all global MIDI mappings in the song into a XRNM file. Returns true when loading/saving succeeded, else false and the error message.
clear_midi_mappings(self)
clear all MIDI mappings in the song
Local Structs
PatternSelection
Selection range in the current pattern
Properties
start_line : integer
?
Start pattern line index
start_track : integer
?
Start track index
start_column : integer
?
Start column index within start_track
end_line : integer
?
End pattern line index
end_track : integer
?
End track index
end_column : integer
?
End column index within end_track
PhraseSelection
Selection range in the current phrase
Properties
start_line : integer
?
Start pattern line index
start_column : integer
?
Start column index within start_track
end_line : integer
?
End pattern line index
end_column : integer
?
End column index within end_track
RenderOptions
Properties
start_pos : renoise.SongPos
by default the song start.
end_pos : renoise.SongPos
by default the song end.
sample_rate : 192000
| 22050
| 44100
| 48000
| 88200
| 96000
by default the players current rate.
bit_depth : 16
| 24
| 32
by default 32.
interpolation : "default"
| "precise"
by default "default".
priority : "high"
| "low"
| "realtime"
by default "high".
renoise.SongPos
Helper class used in Transport and Song, representing a position in the song.
Properties
sequence : integer
Position in the pattern sequence.
line : integer
Position in the pattern at the given pattern sequence.
renoise.Track
Track component of a Renoise song.
- Constants
- Properties
- type :
renoise.Track.TrackType
- name :
string
- name_observable :
renoise.Document.Observable
- color :
RGBColor
- color_observable :
renoise.Document.Observable
- color_blend :
integer
- color_blend_observable :
renoise.Document.Observable
- mute_state :
renoise.Track.MuteState
- mute_state_observable :
renoise.Document.Observable
- solo_state :
boolean
- solo_state_observable :
renoise.Document.Observable
- prefx_volume :
renoise.DeviceParameter
- prefx_panning :
renoise.DeviceParameter
- prefx_width :
renoise.DeviceParameter
- postfx_volume :
renoise.DeviceParameter
- postfx_panning :
renoise.DeviceParameter
- collapsed :
boolean
- collapsed_observable :
renoise.Document.Observable
- group_parent :
renoise.GroupTrack
- available_output_routings :
string
[] - output_routing :
string
- output_routing_observable :
renoise.Document.Observable
- output_delay :
number
- output_delay_observable :
renoise.Document.Observable
- max_effect_columns :
integer
- min_effect_columns :
integer
- max_note_columns :
integer
- min_note_columns :
integer
- visible_effect_columns :
integer
- visible_effect_columns_observable :
renoise.Document.Observable
- visible_note_columns :
integer
- visible_note_columns_observable :
renoise.Document.Observable
- volume_column_visible :
boolean
- volume_column_visible_observable :
renoise.Document.Observable
- panning_column_visible :
boolean
- panning_column_visible_observable :
renoise.Document.Observable
- delay_column_visible :
boolean
- delay_column_visible_observable :
renoise.Document.Observable
- sample_effects_column_visible :
boolean
- sample_effects_column_visible_observable :
renoise.Document.Observable
- available_devices :
string
[] - available_device_infos :
AudioDeviceInfo
[] - devices :
renoise.AudioDevice
[] - devices_observable :
renoise.Document.ObservableList
- type :
- Functions
- insert_device_at(self, device_path :
string
, device_index :integer
) - delete_device_at(self, device_index :
any
) - swap_devices_at(self, device_index1 :
integer
, device_index2 :integer
) - device(self, device_index :
integer
) - mute(self)
- unmute(self)
- solo(self)
- column_is_muted(self, column_index :
integer
) - column_is_muted_observable(self, column_index :
integer
) - set_column_is_muted(self, column_index :
integer
, muted :boolean
) - column_name(self, column_index :
integer
) - column_name_observable(self, column_index :
integer
) - set_column_name(self, column_index :
integer
, name :string
) - swap_note_columns_at(self, column_index1 :
integer
, column_index2 :integer
) - swap_effect_columns_at(self, column_index1 :
integer
, column_index2 :integer
)
- insert_device_at(self, device_path :
- Local Structs
- AudioDeviceInfo
Constants
TrackType
{ TRACK_TYPE_SEQUENCER: integer = 1, TRACK_TYPE_MASTER: integer = 2, TRACK_TYPE_SEND: integer = 3, TRACK_TYPE_GROUP: integer = 4, }
MuteState
{ MUTE_STATE_ACTIVE: integer = 1, MUTE_STATE_OFF: integer = 2, MUTE_STATE_MUTED: integer = 3, }
Properties
type : renoise.Track.TrackType
READ-ONLY
name : string
Name, as visible in track headers
name_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
color : RGBColor
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
color_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
color_blend : integer
Range: (0 - 100) Color blend in percent
color_blend_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
mute_state : renoise.Track.MuteState
Mute and solo states. Not available for the master track.
mute_state_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
solo_state : boolean
solo_state_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
prefx_volume : renoise.DeviceParameter
READ-ONLY
prefx_panning : renoise.DeviceParameter
READ-ONLY
prefx_width : renoise.DeviceParameter
READ-ONLY
postfx_volume : renoise.DeviceParameter
READ-ONLY
postfx_panning : renoise.DeviceParameter
READ-ONLY
collapsed : boolean
Collapsed/expanded visual appearance.
collapsed_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
group_parent : renoise.GroupTrack
READ-ONLY
available_output_routings : string
[]
READ-ONLY
output_routing : string
One of
available_output_routings
output_routing_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
output_delay : number
Range: (-100.0-100.0) in ms
output_delay_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
max_effect_columns : integer
READ-ONLY 8 OR 0 depending on the track type
min_effect_columns : integer
READ-ONLY 1 OR 0 depending on the track type
max_note_columns : integer
READ-ONLY 12 OR 0 depending on the track type
min_note_columns : integer
READ-ONLY 1 OR 0 depending on the track type
visible_effect_columns : integer
1-8 OR 0-8, depending on the track type
visible_effect_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
visible_note_columns : integer
0 OR 1-12, depending on the track type
visible_note_columns_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
volume_column_visible : boolean
volume_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
panning_column_visible : boolean
panning_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
delay_column_visible : boolean
delay_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
sample_effects_column_visible : boolean
sample_effects_column_visible_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
available_devices : string
[]
READ-ONLY FX devices this track can handle.
available_device_infos : AudioDeviceInfo
[]
READ-ONLY Array of tables containing information about the devices.
devices : renoise.AudioDevice
[]
READ-ONLY List of audio DSP FX.
devices_observable : renoise.Document.ObservableList
Track changes to document lists by attaching listener functions to it. NB: Notifiers will not broadcast changes made to list items, but only changes to the lists layout (items got added, removed, swapped).
Functions
insert_device_at(self, device_path : string
, device_index : integer
)
Insert a new device at the given position.
device_path
must be one ofrenoise.Track.available_devices
.
delete_device_at(self, device_index : any
)
Delete an existing device in a track. The mixer device at index 1 can not be deleted from any track.
swap_devices_at(self, device_index1 : integer
, device_index2 : integer
)
Swap the positions of two devices in the device chain. The mixer device at index 1 can not be swapped or moved.
device(self, device_index : integer
)
Access to a single device by index. Use property
devices
to iterate over all devices and to query the device count.
mute(self)
Uses default mute state from the prefs. Not for the master track.
unmute(self)
solo(self)
column_is_muted(self, column_index : integer
)
->
boolean
Note column mutes. Only valid within (1-track.max_note_columns)
column_is_muted_observable(self, column_index : integer
)
set_column_is_muted(self, column_index : integer
, muted : boolean
)
column_name(self, column_index : integer
)
->
string
Note column names. Only valid within (1-track.max_note_columns)
column_name_observable(self, column_index : integer
)
set_column_name(self, column_index : integer
, name : string
)
swap_note_columns_at(self, column_index1 : integer
, column_index2 : integer
)
Swap the positions of two note or effect columns within a track.
swap_effect_columns_at(self, column_index1 : integer
, column_index2 : integer
)
Local Structs
AudioDeviceInfo
Audio device info
Properties
path : string
The device's path used by
renoise.Track:insert_device_at
name : string
The device's name
short_name : string
The device's name as displayed in shortened lists
favorite_name : string
The device's name as displayed in favorites
is_favorite : boolean
true if the device is a favorite
is_bridged : boolean
true if the device is a bridged plugin
Local Aliases
RGBColor
{ 1 : integer
, 2 : integer
, 3 : integer
}
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
renoise.Transport
Transport component of the Renoise song.
- Constants
- Properties
- playing :
boolean
- playing_observable :
renoise.Document.Observable
- timing_model :
renoise.Transport.TimingModel
- bpm :
number
- bpm_observable :
renoise.Document.Observable
- lpb :
integer
- lpb_observable :
renoise.Document.Observable
- tpl :
integer
- tpl_observable :
renoise.Document.Observable
- playback_pos :
renoise.SongPos
- playback_pos_beats :
number
- edit_pos :
renoise.SongPos
- edit_pos_beats :
number
- song_length :
renoise.SongPos
- song_length_beats :
number
- loop_start :
renoise.SongPos
- loop_end :
renoise.SongPos
- loop_range :
renoise.SongPos
[] - loop_start_beats :
number
- loop_end_beats :
number
- loop_range_beats :
number
[] - loop_sequence_start :
integer
- loop_sequence_end :
integer
- loop_sequence_range :
integer
[] - loop_pattern :
boolean
- loop_pattern_observable :
renoise.Document.Observable
- loop_block_enabled :
boolean
- loop_block_start_pos :
renoise.SongPos
- loop_block_range_coeff :
integer
- edit_mode :
boolean
- edit_mode_observable :
renoise.Document.Observable
- edit_step :
integer
- edit_step_observable :
renoise.Document.Observable
- octave :
integer
- octave_observable :
renoise.Document.Observable
- metronome_enabled :
boolean
- metronome_enabled_observable :
renoise.Document.Observable
- metronome_beats_per_bar :
integer
- metronome_beats_per_bar_observable :
renoise.Document.Observable
- metronome_lines_per_beat :
integer
- metronome_lines_per_beat_observable :
renoise.Document.Observable
- metronome_precount_enabled :
boolean
- metronome_precount_enabled_observable :
renoise.Document.Observable
- metronome_precount_bars :
integer
- metronome_precount_bars_observable :
renoise.Document.Observable
- record_quantize_enabled :
boolean
- record_quantize_enabled_observable :
renoise.Document.Observable
- record_quantize_lines :
integer
- record_quantize_lines_observable :
renoise.Document.Observable
- record_parameter_mode :
renoise.Transport.RecordParameterMode
- record_parameter_mode_observable :
renoise.Document.Observable
- follow_player :
boolean
- follow_player_observable :
renoise.Document.Observable
- wrapped_pattern_edit :
boolean
- wrapped_pattern_edit_observable :
renoise.Document.Observable
- single_track_edit_mode :
boolean
- single_track_edit_mode_observable :
renoise.Document.Observable
- groove_enabled :
boolean
- groove_enabled_observable :
renoise.Document.Observable
- groove_amounts :
number
[] - groove_assignment_observable :
renoise.Document.Observable
- track_headroom :
number
- track_headroom_observable :
renoise.Document.Observable
- keyboard_velocity_enabled :
boolean
- keyboard_velocity_enabled_observable :
renoise.Document.Observable
- keyboard_velocity :
integer
- keyboard_velocity_observable :
renoise.Document.Observable
- playing :
- Functions
- panic(self)
- start(self, mode :
renoise.Transport.PlayMode
) - start_at(self, line :
integer
) - start_at(self, song_pos :
renoise.SongPos
) - stop(self)
- trigger_sequence(self, sequence_pos :
integer
) - add_scheduled_sequence(self, sequence_pos :
integer
) - set_scheduled_sequence(self, sequence_pos :
integer
) - loop_block_move_forwards(self)
- loop_block_move_backwards(self)
- start_stop_sample_recording(self)
- cancel_sample_recording(self)
Constants
PlayMode
{ PLAYMODE_RESTART_PATTERN: integer = 1, PLAYMODE_CONTINUE_PATTERN: integer = 2, }
RecordParameterMode
{ RECORD_PARAMETER_MODE_PATTERN: integer = 1, RECORD_PARAMETER_MODE_AUTOMATION: integer = 2, }
TimingModel
{ TIMING_MODEL_SPEED: integer = 1, TIMING_MODEL_LPB: integer = 2, }
Properties
playing : boolean
Playing
playing_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
timing_model : renoise.Transport.TimingModel
READ-ONLY Old school speed or new LPB timing used? With
TIMING_MODEL_SPEED
, tpl is used as speed factor. The lpb property is unused then. WithTIMING_MODEL_LPB
, tpl is used as event rate for effects only and lpb defines relationship between pattern lines and beats.
bpm : number
Range: (32 - 999) Beats per Minute
bpm_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
lpb : integer
Range: (1 - 256) Lines per Beat
lpb_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
tpl : integer
Range: (1 - 16) Ticks per Line
tpl_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
playback_pos : renoise.SongPos
Playback position
playback_pos_beats : number
Range: (0 - song_end_beats) Song position in beats
edit_pos : renoise.SongPos
Edit position
edit_pos_beats : number
Range: (0 - song_end_beats) Song position in beats
song_length : renoise.SongPos
READ-ONLY
song_length_beats : number
READ-ONLY
loop_start : renoise.SongPos
READ-ONLY
loop_end : renoise.SongPos
READ-ONLY
loop_range : renoise.SongPos
[]
{loop start, loop end}
loop_start_beats : number
READ-ONLY Range: (0 - song_end_beats)
loop_end_beats : number
READ-ONLY Range: (0 - song_end_beats)
loop_range_beats : number
[]
{loop start beats, loop end beats}
loop_sequence_start : integer
READ-ONLY 0 or Range: (1 - sequence length)
loop_sequence_end : integer
READ-ONLY 0 or Range: (1 - sequence length)
loop_sequence_range : integer
[]
{} or Range(sequence start, sequence end)
loop_pattern : boolean
Pattern Loop On/Off
loop_pattern_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
loop_block_enabled : boolean
Block Loop On/Off
loop_block_start_pos : renoise.SongPos
Start of block loop
loop_block_range_coeff : integer
Range: (2 - 16)
edit_mode : boolean
Edit modes
edit_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
edit_step : integer
Range: (0 - 64)
edit_step_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
octave : integer
Range: (0 - 8)
octave_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
metronome_enabled : boolean
Metronome
metronome_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
metronome_beats_per_bar : integer
Range: (1 - 16)
metronome_beats_per_bar_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
metronome_lines_per_beat : integer
Range: (1 - 256) or 0 = songs current LPB
metronome_lines_per_beat_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
metronome_precount_enabled : boolean
Metronome precount
metronome_precount_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
metronome_precount_bars : integer
Range: (1 - 4)
metronome_precount_bars_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
record_quantize_enabled : boolean
Quantize
record_quantize_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
record_quantize_lines : integer
Range: (1 - 32)
record_quantize_lines_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
record_parameter_mode : renoise.Transport.RecordParameterMode
Record parameter
record_parameter_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
follow_player : boolean
Follow, wrapped pattern, single track modes
follow_player_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
wrapped_pattern_edit : boolean
wrapped_pattern_edit_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
single_track_edit_mode : boolean
single_track_edit_mode_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
groove_enabled : boolean
Groove. (aka Shuffle)
groove_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
groove_amounts : number
[]
table with 4 numbers in Range: (0 - 1)
groove_assignment_observable : renoise.Document.Observable
Attach notifiers that will be called as soon as any groove value changed.
track_headroom : number
Range: (math.db2lin(-12) - math.db2lin(0))
track_headroom_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
keyboard_velocity_enabled : boolean
Computer Keyboard Velocity.
keyboard_velocity_enabled_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
keyboard_velocity : integer
Range: (0 - 127)
keyboard_velocity_observable : renoise.Document.Observable
Track changes to document properties or general states by attaching listener functions to it.
Functions
panic(self)
Panic.
start(self, mode : renoise.Transport.PlayMode
)
Start playing in song or pattern mode.
start_at(self, line : integer
)
Start playing the currently edited pattern at the given line offset
start_at(self, song_pos : renoise.SongPos
)
Start playing a the given renoise.SongPos (sequence pos and line)
stop(self)
Stop playing. When already stopped this just stops all playing notes.
trigger_sequence(self, sequence_pos : integer
)
Immediately start playing at the given sequence position.
add_scheduled_sequence(self, sequence_pos : integer
)
Append the sequence to the scheduled sequence list. Scheduled playback positions will apply as soon as the currently playing pattern play to end.
set_scheduled_sequence(self, sequence_pos : integer
)
Replace the scheduled sequence list with the given sequence.
loop_block_move_forwards(self)
Move the block loop one segment forwards, when possible.
loop_block_move_backwards(self)
Move the block loop one segment backwards, when possible.
start_stop_sample_recording(self)
Start a new sample recording when the sample dialog is visible, otherwise stop and finish it.
cancel_sample_recording(self)
Cancel a currently running sample recording when the sample dialog is visible, otherwise do nothing.
renoise.ViewBuilder
Class which is used to construct new views. All view properties can optionally be in-lined in a passed construction table:
local vb = renoise.ViewBuilder() -- create a new ViewBuilder vb:button { text = "ButtonText" } -- is the same as my_button = vb:button(); my_button.text = "ButtonText"
Besides the listed class properties, you can also specify the following "extra" properties in the passed table:
id = "SomeString": Can be use to resolve the view later on, e.g.
vb.views.SomeString
orvb.views["SomeString"]
Nested child views: Add child views to the currently specified view.
examples:
Creates a column view with
margin = 1
and adds two text views to the column.vb:column { margin = 1, vb:text { text = "Text1" }, vb:text { text = "Text1" } }
- Constants
- Properties
- Functions
- column(self, properties :
RackViewProperties
) - row(self, properties :
RackViewProperties
) - horizontal_aligner(self, properties :
AlignerViewProperties
) - vertical_aligner(self, properties :
AlignerViewProperties
) - space(self, properties :
ViewProperties
) - text(self, properties :
TextViewProperties``?
) - multiline_text(self, properties :
MultilineTextViewProperties``?
) - textfield(self, properties :
TextFieldProperties``?
) - multiline_textfield(self, properties :
MultilineTextFieldProperties``?
) - bitmap(self, properties :
BitmapViewProperties``?
) - button(self, properties :
ButtonProperties``?
) - checkbox(self, properties :
CheckBoxProperties``?
) - switch(self, properties :
ButtonSwitchProperties``?
) - popup(self, properties :
PopUpMenuProperties``?
) - chooser(self, properties :
ChooserProperties``?
) - valuebox(self, properties :
ValueBoxProperties``?
) - value(self, properties :
ValueViewProperties``?
) - valuefield(self, properties :
ValueFieldProperties``?
) - slider(self, properties :
SliderProperties``?
) - minislider(self, properties :
MiniSliderProperties``?
) - rotary(self, properties :
RotaryEncoderProperties``?
) - xypad(self, properties :
XYPadProperties``?
)
- column(self, properties :
- Local Structs
- AlignerViewProperties
- BitmapViewProperties
- ButtonProperties
- Properties
- text :
ButtonLabel``?
- bitmap :
ButtonBitmapPath``?
- color :
ButtonColor``?
- notifier :
ButtonNotifier``?
- pressed :
ButtonNotifier``?
- released :
ButtonNotifier``?
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- text :
- Properties
- ButtonSwitchProperties
- CheckBoxProperties
- ChooserProperties
- MiniSliderProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- bind :
ViewNumberObservable``?
- value :
SliderNumberValue``?
- notifier :
NumberValueNotifier``?
- min :
SliderMinValue``?
- max :
SliderMaxValue``?
- default :
SliderDefaultValue``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- active :
- Properties
- MultilineTextFieldProperties
- Properties
- bind :
ViewStringListObservable``?
- active :
TextActive``?
- value :
TextMultilineString``?
- notifier :
StringChangeNotifier``?
- text :
TextValueAlias``?
- paragraphs :
TextParagraphs``?
- font :
TextFontStyle``?
- style :
TextBackgroundStyle``?
- edit_mode :
TextEditMode``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- bind :
- Properties
- MultilineTextViewProperties
- PopUpMenuProperties
- RackViewProperties
- RotaryEncoderProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- bind :
ViewNumberObservable``?
- value :
SliderNumberValue``?
- notifier :
NumberValueNotifier``?
- min :
SliderMinValue``?
- max :
SliderMaxValue``?
- default :
SliderDefaultValue``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- active :
- Properties
- SliderProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- bind :
ViewNumberObservable``?
- value :
SliderNumberValue``?
- notifier :
NumberValueNotifier``?
- min :
SliderMinValue``?
- max :
SliderMaxValue``?
- steps :
SliderStepAmounts``?
- default :
SliderDefaultValue``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- active :
- Properties
- TextFieldProperties
- Properties
- bind :
ViewStringObservable``?
- active :
TextActive``?
- value :
TextValue``?
- notifier :
StringChangeNotifier``?
- text :
TextValueAlias``?
- align :
TextAlignment``?
- edit_mode :
TextEditMode``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- bind :
- Properties
- TextViewProperties
- ValueBoxProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- bind :
ViewNumberObservable``?
- value :
SliderNumberValue``?
- notifier :
NumberValueNotifier``?
- min :
ValueBoxMinValue``?
- max :
ValueBoxMaxValue``?
- steps :
SliderStepAmounts``?
- tostring :
PairedShowNumberAsString``?
- tonumber :
PairedParseStringAsNumber``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- active :
- Properties
- ValueFieldProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- bind :
ViewNumberObservable``?
- value :
SliderNumberValue``?
- notifier :
NumberValueNotifier``?
- min :
SliderMinValue``?
- max :
SliderMaxValue``?
- align :
TextAlignment``?
- tostring :
PairedShowNumberAsString``?
- tonumber :
PairedParseStringAsNumber``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- active :
- Properties
- ValueViewProperties
- ViewProperties
- XYPadProperties
- Properties
- active :
ControlActive``?
- midi_mapping :
ControlMidiMappingString``?
- id :
ViewId``?
- width :
ViewDimension``?
- height :
ViewDimension``?
- visible :
ViewVisibility``?
- tooltip :
ViewTooltip``?
- bind :
XYPadObservables``?
- value :
XYPadValues``?
- snapback :
XYPadSnapbackValues``?
- notifier :
XYValueNotifier``?
- min :
XYPadMinValues``?
- max :
XYPadMaxValues``?
- active :
- Local Aliases
- AlignerMode
- BitmapImagePath
- BitmapMode
- BitmapPath
- BooleanValueNotifierFunction
- BooleanValueNotifierMemberFunction
- BooleanValueNotifierMethod1
- BooleanValueNotifierMethod2
- ButtonBitmapPath
- ButtonColor
- ButtonLabel
- ButtonNotifier
- CheckBoxBoolean
- CheckBoxBooleanNotifier
- ControlActive
- ControlMidiMappingString
- IntegerNotifier
- IntegerValueNotifierFunction
- IntegerValueNotifierMemberFunction
- IntegerValueNotifierMethod1
- IntegerValueNotifierMethod2
- ItemLabels
- ListElementAdded
- ListElementChange
- ListElementRemoved
- ListElementsSwapped
- ListNotifierFunction
- ListNotifierMemberContext
- NotifierFunction
- NotifierMemberContext
- NotifierMemberFunction
- NotifierMethod1
- NotifierMethod2
- NumberValueNotifier
- NumberValueNotifierFunction
- NumberValueNotifierMemberFunction
- NumberValueNotifierMethod1
- NumberValueNotifierMethod2
- PairedParseStringAsNumber
- PairedShowNumberAsString
- PopupItemLabels
- RGBColor
- RackMargin
- RackSpacing
- RackUniformity
- SelectedItem
- ShowNumberAsString
- SliderDefaultValue
- SliderMaxValue
- SliderMinValue
- SliderNumberValue
- SliderStepAmounts
- StringChangeNotifier
- StringValueNotifierFunction
- StringValueNotifierMemberFunction
- StringValueNotifierMethod1
- StringValueNotifierMethod2
- TextActive
- TextAlignment
- TextBackgroundStyle
- TextEditMode
- TextFontStyle
- TextMultilineString
- TextParagraphs
- TextSingleLineString
- TextStyle
- TextValue
- TextValueAlias
- ValueBoxMaxValue
- ValueBoxMinValue
- ViewBackgroundStyle
- ViewBooleanObservable
- ViewDimension
- ViewId
- ViewNumberObservable
- ViewStringListObservable
- ViewStringObservable
- ViewTooltip
- ViewVisibility
- XYPadMaxValues
- XYPadMinValues
- XYPadObservables
- XYPadSnapbackValues
- XYPadValues
- XYValueNotifier
- XYValueNotifierFunction
- XYValueNotifierMemberFunction
- XYValueNotifierMethod1
- XYValueNotifierMethod2
- Properties
Constants
DEFAULT_CONTROL_MARGIN : integer
The default margin for all control views
DEFAULT_CONTROL_SPACING : integer
The default spacing for all control views
DEFAULT_CONTROL_HEIGHT : integer
The default height for control views
DEFAULT_MINI_CONTROL_HEIGHT : integer
The default height for mini-sliders
DEFAULT_DIALOG_MARGIN : integer
The default margin for dialogs
DEFAULT_DIALOG_SPACING : integer
The default spacing for dialogs
DEFAULT_DIALOG_BUTTON_HEIGHT : integer
The default height for buttons
Properties
views : table<string
, renoise.Views.View
>
Table of views, which got registered via the "id" property View id is the table key, the table's value is the view's object.
examples:
vb:text { id="my_view", text="some_text"} vb.views.my_view.visible = false --or vb.views["my_view"].visible = false
Functions
column(self, properties : RackViewProperties
)
You can add nested child views when constructing a column or row by including them in the constructor table in the views property.
examples:
vb:column { margin = 1, vb:text { text = "Text1" }, vb:text { text = "Text2" } }
row(self, properties : RackViewProperties
)
You can add nested child views when constructing a column or row by including them in the constructor table in the views property.
examples:
vb:column { margin = 1, vb:text { text = "Text1" }, vb:text { text = "Text2" } }
horizontal_aligner(self, properties : AlignerViewProperties
)
You can add nested child views when constructing aligners by including them in the constructor table.
examples:
vb:horizontal_aligner { mode = "center", vb:text { text = "Text1" }, vb:text { text = "Text2" } }
vertical_aligner(self, properties : AlignerViewProperties
)
You can add nested child views when constructing aligners by including them in the constructor table.
examples:
vb:horizontal_aligner { mode = "center", vb:text { text = "Text1" }, vb:text { text = "Text2" } }
space(self, properties : ViewProperties
)
You can create an empty space in layouts with a space.
examples:
--Empty space in layouts vb:row { vb:button { text = "Some Button" }, vb:space { -- extra spacing between buttons width = 8 }, vb:button { text = "Another Button" }, }
text(self, properties : TextViewProperties
?
)
See: renoise.Views.Text
multiline_text(self, properties : MultilineTextViewProperties
?
)
textfield(self, properties : TextFieldProperties
?
)
multiline_textfield(self, properties : MultilineTextFieldProperties
?
)
->
renoise.Views.MultiLineTextField
bitmap(self, properties : BitmapViewProperties
?
)
See: renoise.Views.Bitmap
button(self, properties : ButtonProperties
?
)
See: renoise.Views.Button
checkbox(self, properties : CheckBoxProperties
?
)
switch(self, properties : ButtonSwitchProperties
?
)
See: renoise.Views.Switch
popup(self, properties : PopUpMenuProperties
?
)
See: renoise.Views.Popup
chooser(self, properties : ChooserProperties
?
)
valuebox(self, properties : ValueBoxProperties
?
)
value(self, properties : ValueViewProperties
?
)
See: renoise.Views.Value
valuefield(self, properties : ValueFieldProperties
?
)
slider(self, properties : SliderProperties
?
)
See: renoise.Views.Slider
minislider(self, properties : MiniSliderProperties
?
)
rotary(self, properties : RotaryEncoderProperties
?
)
xypad(self, properties : XYPadProperties
?
)
See: renoise.Views.XYPad
Local Structs
AlignerViewProperties
Properties
margin : RackMargin
?
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
spacing : RackSpacing
?
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
mode : AlignerMode
?
- Default: "left" (for horizontal_aligner) "top" (for vertical_aligner)
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
BitmapViewProperties
Properties
mode : BitmapMode
?
Setup how the bitmap should be drawn, recolored. Available modes are:
bitmap : BitmapPath
?
Supported bitmap file formats are *.bmp, *.png or *.tif (no transparency).
notifier : ButtonNotifier
?
A click notifier
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ButtonProperties
Properties
text : ButtonLabel
?
The text label of the button
- Default: ""
bitmap : ButtonBitmapPath
?
If set, existing text is removed and the loaded image is displayed instead. Supported bitmap file formats are ".bmp", ".png" and ".tiff". Colors in bitmaps will be overridden by the button's theme color, using black as the transparent color for BMPs and TIFFS, and the alpha channel for PNGs. All other colors are mapped to the theme color according to their grey value, so plain white is the target theme color, and all other colors blend into the button's background color of the theme.
color : ButtonColor
?
When set, the unpressed button's background will be drawn in the specified color. A text color is automatically selected unless explicitly set, to make sure it's always visible. Set color {0,0,0} to enable the theme colors for the button again.
notifier : ButtonNotifier
?
A click notifier
pressed : ButtonNotifier
?
A click notifier
released : ButtonNotifier
?
A click notifier
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ButtonSwitchProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SelectedItem
?
The currently selected item's index
notifier : IntegerNotifier
?
Set up a notifier that will be called whenever a new item is picked
items : ItemLabels
?
A list of buttons labels to show in order. Must have more than one item.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
CheckBoxProperties
Properties
bind : ViewBooleanObservable
?
Bind the view's value to a renoise.Document.ObservableBoolean object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : CheckBoxBoolean
?
The current state of the checkbox, expressed as boolean.
- Default: false
notifier : CheckBoxBooleanNotifier
?
A notifier for when the checkbox is toggled
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ChooserProperties
Properties
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SelectedItem
?
The currently selected item's index
notifier : IntegerNotifier
?
Set up a notifier that will be called whenever a new item is picked
items : ItemLabels
?
A list of buttons labels to show in order. Must have more than one item.
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
MiniSliderProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
min : SliderMinValue
?
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
?
The maximum value that can be set using the view
- Default: 1.0
default : SliderDefaultValue
?
The default value that will be re-applied on double-click
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
MultilineTextFieldProperties
Properties
bind : ViewStringListObservable
?
Bind the view's value to a renoise.Document.ObservableStringList object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
active : TextActive
?
When false, text is displayed but can not be entered/modified by the user.
- Default: true
value : TextMultilineString
?
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
notifier : StringChangeNotifier
?
Set up a notifier for text changes
text : TextValueAlias
?
Exactly the same as "value"; provided for consistency.
- Default: ""
paragraphs : TextParagraphs
?
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
font : TextFontStyle
?
The style that the text should be displayed with.
style : TextBackgroundStyle
?
Setup the text view's background:
edit_mode : TextEditMode
?
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
MultilineTextViewProperties
Properties
text : TextMultilineString
?
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
paragraphs : TextParagraphs
?
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
font : TextFontStyle
?
The style that the text should be displayed with.
style : TextBackgroundStyle
?
Setup the text view's background:
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
PopUpMenuProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SelectedItem
?
The currently selected item's index
notifier : IntegerNotifier
?
Set up a notifier that will be called whenever a new item is picked
items : PopupItemLabels
?
A list of buttons labels to show in order The list can be empty, then "None" is displayed and the value won't change.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
RackViewProperties
Properties
margin : RackMargin
?
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
spacing : RackSpacing
?
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
style : ViewBackgroundStyle
?
Setup a background style for the view.
uniform : RackUniformity
?
When set to true, all child views in the rack are automatically resized to the max size of all child views (width in ViewBuilder.column, height in ViewBuilder.row). This can be useful to automatically align all sub columns/panels to the same size. Resizing is done automatically, as soon as a child view size changes or new children are added.
- Default: false
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
RotaryEncoderProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
min : SliderMinValue
?
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
?
The maximum value that can be set using the view
- Default: 1.0
default : SliderDefaultValue
?
The default value that will be re-applied on double-click
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
SliderProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
min : SliderMinValue
?
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
?
The maximum value that can be set using the view
- Default: 1.0
steps : SliderStepAmounts
?
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
default : SliderDefaultValue
?
The default value that will be re-applied on double-click
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
TextFieldProperties
Properties
bind : ViewStringObservable
?
Bind the view's value to a renoise.Document.ObservableString object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
active : TextActive
?
When false, text is displayed but can not be entered/modified by the user.
- Default: true
value : TextValue
?
The currently shown text. The text will not be updated when editing, rather only after editing is complete (return is pressed, or focus is lost).
- Default: ""
notifier : StringChangeNotifier
?
Set up a notifier for text changes
text : TextValueAlias
?
Exactly the same as "value"; provided for consistency.
- Default: ""
align : TextAlignment
?
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
edit_mode : TextEditMode
?
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
TextViewProperties
Properties
text : TextSingleLineString
?
The text that should be displayed. Setting a new text will resize the view in order to make the text fully visible (expanding only).
- Default: ""
font : TextFontStyle
?
The style that the text should be displayed with.
style : TextStyle
?
Get/set the color style the text should be displayed with.
align : TextAlignment
?
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ValueBoxProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
min : ValueBoxMinValue
?
The minimum value that can be set using the view
- Default: 0
max : ValueBoxMaxValue
?
The maximum value that can be set using the view
- Default: 100
steps : SliderStepAmounts
?
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
tostring : PairedShowNumberAsString
?
Make sure to also set
tonumber
if you set this.
tonumber : PairedParseStringAsNumber
?
Make sure to also set
tostring
if you set this.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ValueFieldProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
min : SliderMinValue
?
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
?
The maximum value that can be set using the view
- Default: 1.0
align : TextAlignment
?
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
tostring : PairedShowNumberAsString
?
Make sure to also set
tonumber
if you set this.
tonumber : PairedParseStringAsNumber
?
Make sure to also set
tostring
if you set this.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ValueViewProperties
Properties
bind : ViewNumberObservable
?
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
value : SliderNumberValue
?
The current value of the view
notifier : NumberValueNotifier
?
Set up a value notifier that will be called whenever the value changes
align : TextAlignment
?
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
font : TextFontStyle
?
The style that the text should be displayed with.
tostring : ShowNumberAsString
?
Set a custom rule on how a number value should be displayed. Useful for showing units like decibel or note values etc. If none are set, a default string/number conversion is done, which simply shows the number with 3 digits after the decimal point. Note: When the callback fails with an error, it will be disabled to avoid a flood of error messages.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewProperties
Base for all View constructor tables in the viewbuilder.
Properties
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
XYPadProperties
Properties
active : ControlActive
?
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
?
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
id : ViewId
?
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
width : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
?
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
visible : ViewVisibility
?
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
tooltip : ViewTooltip
?
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
bind : XYPadObservables
?
Bind the view's value to a pair of renoise.Document.ObservableNumber objects. Automatically keep both values in sync. Will change the Observables' values as soon as the view's value changes, and change the view's values as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object. Just like in the other XYPad properties, a table with the fields X and Y is expected here and not a single value. So you have to bind two ObservableNumber object to the pad.
value : XYPadValues
?
A table of the XYPad's current values on each axis
snapback : XYPadSnapbackValues
?
A table of snapback values for each axis When snapback is enabled, the pad will revert its values to the specified snapback values as soon as the mouse button is released in the pad. When disabled, releasing the mouse button will not change the value. You can disable snapback at runtime by setting it to nil or an empty table.
notifier : XYValueNotifier
?
Set up a value notifier function that will be used whenever the pad's values change
min : XYPadMinValues
?
A table of allowed minimum values for each axis
- Default: {x: 0.0, y: 0.0}
max : XYPadMaxValues
?
A table of allowed maximum values for each axis
- Default: {x: 1.0, y: 1.0}
Local Aliases
AlignerMode
"bottom"
| "center"
| "distribute"
| "justify"
| "left"
| "right"
| "top"
-- * Default: "left" (for horizontal_aligner) "top" (for vertical_aligner) AlignerMode: | "left" -- align from left to right (for horizontal_aligner only) | "right" -- align from right to left (for horizontal_aligner only) | "top" -- align from top to bottom (for vertical_aligner only) | "bottom" -- align from bottom to top (for vertical_aligner only) | "center" -- center all views | "justify" -- keep outer views at the borders, distribute the rest | "distribute" -- equally distributes views over the aligners width/height
BitmapImagePath
You can load an image from your tool's directory, or use one from Renoise's built-in icons.
- For the built-in icons, use "Icons/ArrowRight.bmp"
- For custom images, use a path relative to your tool's root folder.
For example "Images/MyBitmap.bmp" will load the image from "com.me.MyTool.xrnx/Images/MyBitmap.bmp".
If your custom path matches a built-in icon's (like "Icons/ArrowRight.bmp"), your image will be loaded instead of the one from Renoise.If you want to support high DPI UI scaling with your bitmaps like the built-in Icons, include high resolution versions with their filenames ending with "@4x"
The following rules will be used when loading bitmaps
- When UI scaling is 100%, only the base bitmaps are used.
- When UI scaling is 125%, the base bitmaps are used, except if there is a
BitmapName@x1.25.bmp
variant.- For all other UI scaling > 125% the "@4x" variants are used and downscaled as needed, except when there is an exact match for the current UI scaling factor (e.g.
BitmapName@1.5x.bmp
for 150%)
BitmapMode
"body_color"
| "button_color"
| "main_color"
| "plain"
| "transparent"
-- Setup how the bitmap should be drawn, recolored. Available modes are: BitmapMode: | "plain" -- bitmap is drawn as is, no recoloring is done (Default) | "transparent" -- same as plain, but black pixels will be fully transparent | "button_color" -- recolor the bitmap, using the theme's button color | "body_color" -- same as 'button_back' but with body text/back color | "main_color" -- same as 'button_back' but with main text/back colors
BitmapPath
Supported bitmap file formats are *.bmp, *.png or *.tif (no transparency).
BooleanValueNotifierFunction
(value : boolean
)
BooleanValueNotifierMemberFunction
(self : NotifierMemberContext
, value : boolean
)
BooleanValueNotifierMethod1
{ 1 : NotifierMemberContext
, 2 : BooleanValueNotifierMemberFunction
}
BooleanValueNotifierMethod2
{ 1 : BooleanValueNotifierMemberFunction
, 2 : NotifierMemberContext
}
ButtonBitmapPath
If set, existing text is removed and the loaded image is displayed instead. Supported bitmap file formats are ".bmp", ".png" and ".tiff". Colors in bitmaps will be overridden by the button's theme color, using black as the transparent color for BMPs and TIFFS, and the alpha channel for PNGs. All other colors are mapped to the theme color according to their grey value, so plain white is the target theme color, and all other colors blend into the button's background color of the theme.
ButtonColor
When set, the unpressed button's background will be drawn in the specified color. A text color is automatically selected unless explicitly set, to make sure it's always visible. Set color {0,0,0} to enable the theme colors for the button again.
ButtonLabel
The text label of the button
- Default: ""
ButtonNotifier
NotifierFunction
| NotifierMethod1
| NotifierMethod2
A click notifier
CheckBoxBoolean
The current state of the checkbox, expressed as boolean.
- Default: false
CheckBoxBooleanNotifier
BooleanValueNotifierFunction
| BooleanValueNotifierMethod1
| BooleanValueNotifierMethod2
A notifier for when the checkbox is toggled
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
IntegerNotifier
IntegerValueNotifierFunction
| IntegerValueNotifierMethod1
| IntegerValueNotifierMethod2
Set up a notifier that will be called whenever a new item is picked
IntegerValueNotifierFunction
(value : integer
)
IntegerValueNotifierMemberFunction
(self : NotifierMemberContext
, value : integer
)
IntegerValueNotifierMethod1
{ 1 : NotifierMemberContext
, 2 : IntegerValueNotifierMemberFunction
}
IntegerValueNotifierMethod2
{ 1 : IntegerValueNotifierMemberFunction
, 2 : NotifierMemberContext
}
ItemLabels
string
[]
A list of buttons labels to show in order. Must have more than one item.
ListElementAdded
{ index : integer
, type : "insert"
}
ListElementChange
ListElementAdded
| ListElementRemoved
| ListElementsSwapped
ListElementRemoved
{ index : integer
, type : "removed"
}
ListElementsSwapped
{ index1 : integer
, index2 : integer
, type : "swapped"
}
ListNotifierFunction
(change : ListElementChange
)
ListNotifierMemberContext
NotifierFunction
fun()
NotifierMemberContext
NotifierMemberFunction
(self : NotifierMemberContext
)
NotifierMethod1
{ 1 : NotifierMemberContext
, 2 : NotifierMemberFunction
}
NotifierMethod2
{ 1 : NotifierMemberFunction
, 2 : NotifierMemberContext
}
NumberValueNotifier
NumberValueNotifierFunction
| NumberValueNotifierMethod1
| NumberValueNotifierMethod2
Set up a value notifier that will be called whenever the value changes
NumberValueNotifierFunction
(value : number
)
NumberValueNotifierMemberFunction
(self : NotifierMemberContext
, value : number
)
NumberValueNotifierMethod1
{ 1 : NotifierMemberContext
, 2 : NumberValueNotifierMemberFunction
}
NumberValueNotifierMethod2
{ 1 : NumberValueNotifierMemberFunction
, 2 : NotifierMemberContext
}
PairedParseStringAsNumber
Make sure to also set
tostring
if you set this.
PairedShowNumberAsString
Make sure to also set
tonumber
if you set this.
PopupItemLabels
string
[]
A list of buttons labels to show in order The list can be empty, then "None" is displayed and the value won't change.
RGBColor
{ 1 : integer
, 2 : integer
, 3 : integer
}
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
RackMargin
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
RackSpacing
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
RackUniformity
When set to true, all child views in the rack are automatically resized to the max size of all child views (width in ViewBuilder.column, height in ViewBuilder.row). This can be useful to automatically align all sub columns/panels to the same size. Resizing is done automatically, as soon as a child view size changes or new children are added.
- Default: false
SelectedItem
The currently selected item's index
ShowNumberAsString
Set a custom rule on how a number value should be displayed. Useful for showing units like decibel or note values etc. If none are set, a default string/number conversion is done, which simply shows the number with 3 digits after the decimal point. Note: When the callback fails with an error, it will be disabled to avoid a flood of error messages.
SliderDefaultValue
The default value that will be re-applied on double-click
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
SliderStepAmounts
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
StringChangeNotifier
StringValueNotifierFunction
| StringValueNotifierMethod1
| StringValueNotifierMethod2
Set up a notifier for text changes
StringValueNotifierFunction
(value : string
)
StringValueNotifierMemberFunction
(self : NotifierMemberContext
, value : string
)
StringValueNotifierMethod1
{ 1 : NotifierMemberContext
, 2 : StringValueNotifierMemberFunction
}
StringValueNotifierMethod2
{ 1 : StringValueNotifierMemberFunction
, 2 : NotifierMemberContext
}
TextActive
When false, text is displayed but can not be entered/modified by the user.
- Default: true
TextAlignment
"center"
| "left"
| "right"
-- Setup the text's alignment. Applies only when the view's size is larger than -- the needed size to draw the text TextAlignment: | "left" -- (Default) | "right" -- aligned to the right | "center" -- center text
TextBackgroundStyle
"body"
| "border"
| "strong"
-- Setup the text view's background: TextBackgroundStyle: | "body" -- simple text color with no background | "strong" -- stronger text color with no background | "border" -- text on a bordered background
TextEditMode
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
TextFontStyle
"big"
| "bold"
| "italic"
| "mono"
| "normal"
-- The style that the text should be displayed with. TextFontStyle: | "normal" -- (Default) | "big" -- big text | "bold" -- bold font | "italic" -- italic font | "mono" -- monospace font
TextMultilineString
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
TextParagraphs
string
[]
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
TextSingleLineString
The text that should be displayed. Setting a new text will resize the view in order to make the text fully visible (expanding only).
- Default: ""
TextStyle
"disabled"
| "normal"
| "strong"
-- Get/set the color style the text should be displayed with. TextStyle: | "normal" -- (Default) | "strong" -- highlighted color | "disabled" -- greyed out color
TextValue
The currently shown text. The text will not be updated when editing, rather only after editing is complete (return is pressed, or focus is lost).
- Default: ""
TextValueAlias
Exactly the same as "value"; provided for consistency.
- Default: ""
ValueBoxMaxValue
The maximum value that can be set using the view
- Default: 100
ValueBoxMinValue
The minimum value that can be set using the view
- Default: 0
ViewBackgroundStyle
"body"
| "border"
| "group"
| "invisible"
| "panel"
| "plain"
-- Setup a background style for the view. ViewBackgroundStyle: | "invisible" -- no background (Default) | "plain" -- undecorated, single coloured background | "border" -- same as plain, but with a bold nested border | "body" -- main "background" style, as used in dialog backgrounds | "panel" -- alternative "background" style, beveled | "group" -- background for "nested" groups within body
ViewBooleanObservable
renoise.Document.ObservableBoolean
Bind the view's value to a renoise.Document.ObservableBoolean object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewId
Unique identifier to resolve the view later on in the viewbuilder, e.g.
vb.views.SomeString
orvb.views["Some String"]
View ids must be unique within a single view builder instance.
ViewNumberObservable
renoise.Document.ObservableNumber
Bind the view's value to a renoise.Document.ObservableNumber object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
ViewStringListObservable
renoise.Document.ObservableStringList
Bind the view's value to a renoise.Document.ObservableStringList object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
ViewStringObservable
renoise.Document.ObservableString
Bind the view's value to a renoise.Document.ObservableString object. Automatically keep them in sync. The view will change the Observable value as soon as its value changes and change the view's value as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
XYPadMaxValues
{ x : SliderMaxValue
, y : SliderMaxValue
}
A table of allowed maximum values for each axis
- Default: {x: 1.0, y: 1.0}
XYPadMinValues
{ x : SliderMinValue
, y : SliderMinValue
}
A table of allowed minimum values for each axis
- Default: {x: 0.0, y: 0.0}
XYPadObservables
{ x : renoise.Document.ObservableNumber
, y : renoise.Document.ObservableNumber
}
Bind the view's value to a pair of renoise.Document.ObservableNumber objects. Automatically keep both values in sync. Will change the Observables' values as soon as the view's value changes, and change the view's values as soon as the Observable's value changes. Notifiers can be added to either the view or the Observable object. Just like in the other XYPad properties, a table with the fields X and Y is expected here and not a single value. So you have to bind two ObservableNumber object to the pad.
XYPadSnapbackValues
A table of snapback values for each axis When snapback is enabled, the pad will revert its values to the specified snapback values as soon as the mouse button is released in the pad. When disabled, releasing the mouse button will not change the value. You can disable snapback at runtime by setting it to nil or an empty table.
XYPadValues
{ x : SliderNumberValue
, y : SliderNumberValue
}
A table of the XYPad's current values on each axis
XYValueNotifier
XYValueNotifierFunction
| XYValueNotifierMethod1
| XYValueNotifierMethod2
Set up a value notifier function that will be used whenever the pad's values change
XYValueNotifierFunction
(value : XYPadValues
)
XYValueNotifierMemberFunction
(self : NotifierMemberContext
, value : XYPadValues
)
XYValueNotifierMethod1
{ 1 : NotifierMemberContext
, 2 : XYValueNotifierMemberFunction
}
XYValueNotifierMethod2
{ 1 : XYValueNotifierMemberFunction
, 2 : NotifierMemberContext
}
renoise.Views
Namespace for renoise view widgets.
renoise.Views.Aligner
Just like a Rack, the Aligner shows no content on its own. It just aligns child views vertically or horizontally. As soon as children are added, the Aligner will expand itself to make sure that all children are visible (including spacing & margins). To make use of modes like "center", you manually have to setup a size that is bigger than the sum of the child sizes.
Properties
margin : RackMargin
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
spacing : RackSpacing
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
mode : AlignerMode
- Default: "left" (for horizontal_aligner) "top" (for vertical_aligner)
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
AlignerMode
"bottom"
| "center"
| "distribute"
| "justify"
| "left"
| "right"
| "top"
-- * Default: "left" (for horizontal_aligner) "top" (for vertical_aligner) AlignerMode: | "left" -- align from left to right (for horizontal_aligner only) | "right" -- align from right to left (for horizontal_aligner only) | "top" -- align from top to bottom (for vertical_aligner only) | "bottom" -- align from bottom to top (for vertical_aligner only) | "center" -- center all views | "justify" -- keep outer views at the borders, distribute the rest | "distribute" -- equally distributes views over the aligners width/height
RackMargin
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
RackSpacing
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Bitmap
Draws a bitmap, or a draws a bitmap which acts like a button (as soon as a notifier is specified). The notifier is called when clicking the mouse somewhere on the bitmap. When using a re-colorable style (see 'mode'), the bitmap is automatically recolored to match the current theme's colors. Mouse hover is also enabled when notifiers are present, to show that the bitmap can be clicked.
* *** + * / \ +---+ | O | o +---+ | ||||||||||||
Properties
mode : BitmapMode
Setup how the bitmap should be drawn, recolored. Available modes are:
bitmap : BitmapPath
Supported bitmap file formats are *.bmp, *.png or *.tif (no transparency).
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NotifierFunction
)
Add mouse click notifier
remove_notifier(self, notifier : NotifierFunction
)
Remove mouse click notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
BitmapImagePath
You can load an image from your tool's directory, or use one from Renoise's built-in icons.
- For the built-in icons, use "Icons/ArrowRight.bmp"
- For custom images, use a path relative to your tool's root folder.
For example "Images/MyBitmap.bmp" will load the image from "com.me.MyTool.xrnx/Images/MyBitmap.bmp".
If your custom path matches a built-in icon's (like "Icons/ArrowRight.bmp"), your image will be loaded instead of the one from Renoise.If you want to support high DPI UI scaling with your bitmaps like the built-in Icons, include high resolution versions with their filenames ending with "@4x"
The following rules will be used when loading bitmaps
- When UI scaling is 100%, only the base bitmaps are used.
- When UI scaling is 125%, the base bitmaps are used, except if there is a
BitmapName@x1.25.bmp
variant.- For all other UI scaling > 125% the "@4x" variants are used and downscaled as needed, except when there is an exact match for the current UI scaling factor (e.g.
BitmapName@1.5x.bmp
for 150%)
BitmapMode
"body_color"
| "button_color"
| "main_color"
| "plain"
| "transparent"
-- Setup how the bitmap should be drawn, recolored. Available modes are: BitmapMode: | "plain" -- bitmap is drawn as is, no recoloring is done (Default) | "transparent" -- same as plain, but black pixels will be fully transparent | "button_color" -- recolor the bitmap, using the theme's button color | "body_color" -- same as 'button_back' but with body text/back color | "main_color" -- same as 'button_back' but with main text/back colors
BitmapPath
Supported bitmap file formats are *.bmp, *.png or *.tif (no transparency).
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NotifierFunction
fun()
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Button
A simple button that calls a custom notifier function when clicked. Supports text or bitmap labels.
+--------+ | Button | +--------+
- Properties
- Functions
- add_pressed_notifier(self, notifier :
NotifierFunction
) - add_released_notifier(self, notifier :
NotifierFunction
) - remove_pressed_notifier(self, notifier :
NotifierFunction
) - remove_released_notifier(self, notifier :
NotifierFunction
) - add_child(self, child :
renoise.Views.View
) - remove_child(self, child :
renoise.Views.View
)
- add_pressed_notifier(self, notifier :
- Local Aliases
Properties
text : ButtonLabel
The text label of the button
- Default: ""
bitmap : ButtonBitmapPath
If set, existing text is removed and the loaded image is displayed instead. Supported bitmap file formats are ".bmp", ".png" and ".tiff". Colors in bitmaps will be overridden by the button's theme color, using black as the transparent color for BMPs and TIFFS, and the alpha channel for PNGs. All other colors are mapped to the theme color according to their grey value, so plain white is the target theme color, and all other colors blend into the button's background color of the theme.
color : ButtonColor
When set, the unpressed button's background will be drawn in the specified color. A text color is automatically selected unless explicitly set, to make sure it's always visible. Set color {0,0,0} to enable the theme colors for the button again.
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_pressed_notifier(self, notifier : NotifierFunction
)
Add/remove button hit/release notifier functions. When a "pressed" notifier is set, the release notifier is guaranteed to be called as soon as the mouse is released, either over your button or anywhere else. When a "release" notifier is set, it is only called when the mouse button is pressed !and! released over your button.
add_released_notifier(self, notifier : NotifierFunction
)
remove_pressed_notifier(self, notifier : NotifierFunction
)
remove_released_notifier(self, notifier : NotifierFunction
)
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
BitmapImagePath
You can load an image from your tool's directory, or use one from Renoise's built-in icons.
- For the built-in icons, use "Icons/ArrowRight.bmp"
- For custom images, use a path relative to your tool's root folder.
For example "Images/MyBitmap.bmp" will load the image from "com.me.MyTool.xrnx/Images/MyBitmap.bmp".
If your custom path matches a built-in icon's (like "Icons/ArrowRight.bmp"), your image will be loaded instead of the one from Renoise.If you want to support high DPI UI scaling with your bitmaps like the built-in Icons, include high resolution versions with their filenames ending with "@4x"
The following rules will be used when loading bitmaps
- When UI scaling is 100%, only the base bitmaps are used.
- When UI scaling is 125%, the base bitmaps are used, except if there is a
BitmapName@x1.25.bmp
variant.- For all other UI scaling > 125% the "@4x" variants are used and downscaled as needed, except when there is an exact match for the current UI scaling factor (e.g.
BitmapName@1.5x.bmp
for 150%)
ButtonBitmapPath
If set, existing text is removed and the loaded image is displayed instead. Supported bitmap file formats are ".bmp", ".png" and ".tiff". Colors in bitmaps will be overridden by the button's theme color, using black as the transparent color for BMPs and TIFFS, and the alpha channel for PNGs. All other colors are mapped to the theme color according to their grey value, so plain white is the target theme color, and all other colors blend into the button's background color of the theme.
ButtonColor
When set, the unpressed button's background will be drawn in the specified color. A text color is automatically selected unless explicitly set, to make sure it's always visible. Set color {0,0,0} to enable the theme colors for the button again.
ButtonLabel
The text label of the button
- Default: ""
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NotifierFunction
fun()
RGBColor
{ 1 : integer
, 2 : integer
, 3 : integer
}
A table of 3 bytes (ranging from 0 to 255) representing the red, green and blue channels of a color. {0xFF, 0xFF, 0xFF} is white {165, 73, 35} is the red from the Renoise logo
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.CheckBox
A single button with a checkbox bitmap, which can be used to toggle something on/off.
+----+ | _/ | +----+
Properties
value : CheckBoxBoolean
The current state of the checkbox, expressed as boolean.
- Default: false
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : BooleanValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : BooleanValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
BooleanValueNotifierFunction
(value : boolean
)
CheckBoxBoolean
The current state of the checkbox, expressed as boolean.
- Default: false
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Chooser
A radio button like set of vertically stacked items. Only one value can be selected at a time.
. Item A o Item B . Item C
Properties
items : ItemLabels
A list of buttons labels to show in order. Must have more than one item.
value : SelectedItem
The currently selected item's index
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : IntegerValueNotifierFunction
)
Add index change notifier
remove_notifier(self, notifier : IntegerValueNotifierFunction
)
Remove index change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
IntegerValueNotifierFunction
(value : integer
)
ItemLabels
string
[]
A list of buttons labels to show in order. Must have more than one item.
SelectedItem
The currently selected item's index
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Control
Control is the base class for all views which let the user change a value or some "state" from the UI.
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.MiniSlider
Same as a slider, but without arrow buttons and a really tiny height. Just like the slider, a mini slider can be horizontal or vertical. It will flip its orientation according to the set width and height. By default horizontal.
--------[]
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
min : SliderMinValue
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
default : SliderDefaultValue
The default value that will be re-applied on double-click
value : SliderNumberValue
The current value of the view
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NumberValueNotifierFunction
(value : number
)
SliderDefaultValue
The default value that will be re-applied on double-click
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.MultiLineText
Shows multiple lines of text, auto-formatting and auto-wrapping paragraphs into lines. Size is not automatically set. As soon as the text no longer fits into the view, a vertical scroll bar will be shown.
by the user.
+--------------+-+ | Text, Bla 1 |+| | Text, Bla 2 | | | Text, Bla 3 | | | Text, Bla 4 |+| +--------------+-+
Properties
text : TextMultilineString
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
paragraphs : TextParagraphs
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
font : TextFontStyle
The style that the text should be displayed with.
style : TextBackgroundStyle
Default: "body"
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
scroll_to_last_line(self)
When a scroll bar is visible (needed), scroll the text to show the last line.
scroll_to_first_line(self)
When a scroll bar is visible, scroll the text to show the first line.
add_line(self, text : string
)
Append text to the existing text. Newlines in the text will create new paragraphs, just like in the "text" property.
clear(self)
Clear the whole text, same as multiline_text.text="".
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
TextBackgroundStyle
"body"
| "border"
| "strong"
-- Setup the text view's background: TextBackgroundStyle: | "body" -- simple text color with no background | "strong" -- stronger text color with no background | "border" -- text on a bordered background
TextFontStyle
"big"
| "bold"
| "italic"
| "mono"
| "normal"
-- The style that the text should be displayed with. TextFontStyle: | "normal" -- (Default) | "big" -- big text | "bold" -- bold font | "italic" -- italic font | "mono" -- monospace font
TextMultilineString
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
TextParagraphs
string
[]
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.MultiLineTextField
Shows multiple text lines of text, auto-wrapping paragraphs into lines. The text can be edited by the user.
+--------------------------+-+ | Editable Te|xt. |+| | | | | With multiple paragraphs | | | and auto-wrapping |+| +--------------------------+-+
- Properties
- Functions
- add_notifier(self, notifier :
StringValueNotifierFunction
) - remove_notifier(self, notifier :
StringValueNotifierFunction
) - scroll_to_last_line(self)
- scroll_to_first_line(self)
- add_line(self, text :
string
) - clear(self)
- add_child(self, child :
renoise.Views.View
) - remove_child(self, child :
renoise.Views.View
)
- add_notifier(self, notifier :
- Local Aliases
Properties
active : TextActive
When false, text is displayed but can not be entered/modified by the user.
- Default: true
value : TextMultilineString
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
text : TextValueAlias
Exactly the same as "value"; provided for consistency.
- Default: ""
paragraphs : TextParagraphs
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
font : TextFontStyle
The style that the text should be displayed with.
style : TextBackgroundStyle
Default: "border"
edit_mode : TextEditMode
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : StringValueNotifierFunction
)
Add value change (text change) notifier
remove_notifier(self, notifier : StringValueNotifierFunction
)
Remove value change (text change) notifier
scroll_to_last_line(self)
When a scroll bar is visible, scroll the text to show the last line.
scroll_to_first_line(self)
When a scroll bar is visible, scroll the text to show the first line.
add_line(self, text : string
)
Append a new text to the existing text. Newline characters in the string will create new paragraphs, otherwise a single paragraph is appended.
clear(self)
Clear the whole text.
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
StringValueNotifierFunction
(value : string
)
TextActive
When false, text is displayed but can not be entered/modified by the user.
- Default: true
TextBackgroundStyle
"body"
| "border"
| "strong"
-- Setup the text view's background: TextBackgroundStyle: | "body" -- simple text color with no background | "strong" -- stronger text color with no background | "border" -- text on a bordered background
TextEditMode
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
TextFontStyle
"big"
| "bold"
| "italic"
| "mono"
| "normal"
-- The style that the text should be displayed with. TextFontStyle: | "normal" -- (Default) | "big" -- big text | "bold" -- bold font | "italic" -- italic font | "mono" -- monospace font
TextMultilineString
The text that should be displayed. Newlines (Windows, Mac or Unix styled) in the text can be used to create paragraphs.
TextParagraphs
string
[]
A table of text lines to be used instead of specifying a single text line with newline characters like "text"
- Default: []
TextValueAlias
Exactly the same as "value"; provided for consistency.
- Default: ""
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Popup
A drop-down menu which shows the currently selected value when closed. When clicked, it pops up a list of all available items.
+--------------++---+ | Current Item || ^ | +--------------++---+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
items : PopupItemLabels
A list of buttons labels to show in order The list can be empty, then "None" is displayed and the value won't change.
value : SelectedItem
The currently selected item's index
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : IntegerValueNotifierFunction
)
Add index change notifier
remove_notifier(self, notifier : IntegerValueNotifierFunction
)
Remove index change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
IntegerValueNotifierFunction
(value : integer
)
PopupItemLabels
string
[]
A list of buttons labels to show in order The list can be empty, then "None" is displayed and the value won't change.
SelectedItem
The currently selected item's index
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Rack
A Rack has no content on its own. It only stacks child views. Either vertically (ViewBuilder.column) or horizontally (ViewBuilder.row). It allows you to create view layouts.
Properties
margin : RackMargin
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
spacing : RackSpacing
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
style : ViewBackgroundStyle
Setup a background style for the view.
uniform : RackUniformity
When set to true, all child views in the rack are automatically resized to the max size of all child views (width in ViewBuilder.column, height in ViewBuilder.row). This can be useful to automatically align all sub columns/panels to the same size. Resizing is done automatically, as soon as a child view size changes or new children are added.
- Default: false
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
RackMargin
Set the "borders" of a rack (left, right, top and bottom inclusively)
- Default: 0 (no borders)
RackSpacing
Set the amount stacked child views are separated by (horizontally in rows, vertically in columns).
- Default: 0 (no spacing)
RackUniformity
When set to true, all child views in the rack are automatically resized to the max size of all child views (width in ViewBuilder.column, height in ViewBuilder.row). This can be useful to automatically align all sub columns/panels to the same size. Resizing is done automatically, as soon as a child view size changes or new children are added.
- Default: false
ViewBackgroundStyle
"body"
| "border"
| "group"
| "invisible"
| "panel"
| "plain"
-- Setup a background style for the view. ViewBackgroundStyle: | "invisible" -- no background (Default) | "plain" -- undecorated, single coloured background | "border" -- same as plain, but with a bold nested border | "body" -- main "background" style, as used in dialog backgrounds | "panel" -- alternative "background" style, beveled | "group" -- background for "nested" groups within body
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.RotaryEncoder
A slider which looks like a potentiometer. Note: when changing the size, the minimum of either width or height will be used to draw and control the rotary, therefore you should always set both equally when possible.
+-+ / \ \ | o | \ | / +-+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
min : SliderMinValue
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
default : SliderDefaultValue
The default value that will be re-applied on double-click
value : SliderNumberValue
The current value of the view
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NumberValueNotifierFunction
(value : number
)
SliderDefaultValue
The default value that will be re-applied on double-click
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Slider
A slider with arrow buttons, which shows and allows editing of values in a custom range. A slider can be horizontal or vertical; will flip its orientation according to the set width and height. By default horizontal.
+---+---------------+ |<|>| --------[] | +---+---------------+
- Properties
- active :
ControlActive
- midi_mapping :
ControlMidiMappingString
- min :
SliderMinValue
- max :
SliderMaxValue
- steps :
SliderStepAmounts
- default :
SliderDefaultValue
- value :
SliderNumberValue
- visible :
ViewVisibility
- width :
ViewDimension
- height :
ViewDimension
- tooltip :
ViewTooltip
- views :
renoise.Views.View
[]
- active :
- Functions
- Local Aliases
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
min : SliderMinValue
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
steps : SliderStepAmounts
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
default : SliderDefaultValue
The default value that will be re-applied on double-click
value : SliderNumberValue
The current value of the view
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NumberValueNotifierFunction
(value : number
)
SliderDefaultValue
The default value that will be re-applied on double-click
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
SliderStepAmounts
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Switch
A set of horizontally aligned buttons, where only one button can be enabled at the same time. Select one of multiple choices, indices.
+-----------+------------+----------+ | Button A | +Button+B+ | Button C | +-----------+------------+----------+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
items : ItemLabels
A list of buttons labels to show in order. Must have more than one item.
value : SelectedItem
The currently selected item's index
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : IntegerValueNotifierFunction
)
Add index change notifier
remove_notifier(self, notifier : IntegerValueNotifierFunction
)
Remove index change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
IntegerValueNotifierFunction
(value : integer
)
ItemLabels
string
[]
A list of buttons labels to show in order. Must have more than one item.
SelectedItem
The currently selected item's index
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Text
Shows a "static" text string. Static just means that its not linked, bound to some value and has no notifiers. The text can not be edited by the user. Nevertheless you can of course change the text at run-time with the "text" property.
Text, Bla 1
Properties
text : TextSingleLineString
The text that should be displayed. Setting a new text will resize the view in order to make the text fully visible (expanding only).
- Default: ""
font : TextFontStyle
The style that the text should be displayed with.
style : TextStyle
Get/set the color style the text should be displayed with.
align : TextAlignment
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
TextAlignment
"center"
| "left"
| "right"
-- Setup the text's alignment. Applies only when the view's size is larger than -- the needed size to draw the text TextAlignment: | "left" -- (Default) | "right" -- aligned to the right | "center" -- center text
TextFontStyle
"big"
| "bold"
| "italic"
| "mono"
| "normal"
-- The style that the text should be displayed with. TextFontStyle: | "normal" -- (Default) | "big" -- big text | "bold" -- bold font | "italic" -- italic font | "mono" -- monospace font
TextSingleLineString
The text that should be displayed. Setting a new text will resize the view in order to make the text fully visible (expanding only).
- Default: ""
TextStyle
"disabled"
| "normal"
| "strong"
-- Get/set the color style the text should be displayed with. TextStyle: | "normal" -- (Default) | "strong" -- highlighted color | "disabled" -- greyed out color
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.TextField
Shows a text string that can be clicked and edited by the user.
+----------------+ | Editable Te|xt | +----------------+
Properties
active : TextActive
When false, text is displayed but can not be entered/modified by the user.
- Default: true
value : TextValue
The currently shown text. The text will not be updated when editing, rather only after editing is complete (return is pressed, or focus is lost).
- Default: ""
text : TextValueAlias
Exactly the same as "value"; provided for consistency.
- Default: ""
align : TextAlignment
Only used when not editing.
edit_mode : TextEditMode
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : StringValueNotifierFunction
)
Add value change (text change) notifier
remove_notifier(self, notifier : StringValueNotifierFunction
)
Remove value change (text change) notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
StringValueNotifierFunction
(value : string
)
TextActive
When false, text is displayed but can not be entered/modified by the user.
- Default: true
TextAlignment
"center"
| "left"
| "right"
-- Setup the text's alignment. Applies only when the view's size is larger than -- the needed size to draw the text TextAlignment: | "left" -- (Default) | "right" -- aligned to the right | "center" -- center text
TextEditMode
True when the text field is focused. setting it at run-time programmatically will focus the text field or remove the focus (focus the dialog) accordingly.
- Default: false
TextValue
The currently shown text. The text will not be updated when editing, rather only after editing is complete (return is pressed, or focus is lost).
- Default: ""
TextValueAlias
Exactly the same as "value"; provided for consistency.
- Default: ""
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.Value
A static text view. Shows a string representation of a number and allows custom "number to string" conversion.
+---+-------+ | 12.1 dB | +---+-------+
Properties
value : SliderNumberValue
The current value of the view
font : TextFontStyle
The style that the text should be displayed with.
align : TextAlignment
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
NumberValueNotifierFunction
(value : number
)
SliderNumberValue
The current value of the view
TextAlignment
"center"
| "left"
| "right"
-- Setup the text's alignment. Applies only when the view's size is larger than -- the needed size to draw the text TextAlignment: | "left" -- (Default) | "right" -- aligned to the right | "center" -- center text
TextFontStyle
"big"
| "bold"
| "italic"
| "mono"
| "normal"
-- The style that the text should be displayed with. TextFontStyle: | "normal" -- (Default) | "big" -- big text | "bold" -- bold font | "italic" -- italic font | "mono" -- monospace font
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.ValueBox
A box with arrow buttons and a text field that can be edited by the user. Allows showing and editing natural numbers in a custom range.
+---+-------+ |<|>| 12 | +---+-------+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
min : ValueBoxMinValue
The minimum value that can be set using the view
- Default: 0
max : ValueBoxMaxValue
The maximum value that can be set using the view
- Default: 100
steps : SliderStepAmounts
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
value : SliderNumberValue
The current value of the view
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NumberValueNotifierFunction
(value : number
)
SliderNumberValue
The current value of the view
SliderStepAmounts
A table containing two numbers representing the step amounts for incrementing and decrementing by clicking the <> buttons. The first value is the small step (applied on left clicks) second value is the big step (applied on right clicks)
ValueBoxMaxValue
The maximum value that can be set using the view
- Default: 100
ValueBoxMinValue
The minimum value that can be set using the view
- Default: 0
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.ValueField
A text view, which shows a string representation of a number and allows custom "number to string" conversion. The value's text can be edited by the user.
+---+-------+ | 12.1 dB | +---+-------+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
min : SliderMinValue
The minimum value that can be set using the view
- Default: 0
max : SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
value : SliderNumberValue
The current value of the view
align : TextAlignment
Setup the text's alignment. Applies only when the view's size is larger than the needed size to draw the text
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_notifier(self, notifier : NumberValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : NumberValueNotifierFunction
)
Remove value change notifier
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
NumberValueNotifierFunction
(value : number
)
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
TextAlignment
"center"
| "left"
| "right"
-- Setup the text's alignment. Applies only when the view's size is larger than -- the needed size to draw the text TextAlignment: | "left" -- (Default) | "right" -- aligned to the right | "center" -- center text
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.View
View is the base class for all child views. All View properties can be applied to any of the following specialized views.
Properties
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
Local Aliases
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
renoise.Views.XYPad
A slider like pad which allows for controlling two values at once. By default it freely moves the XY values, but it can also be configured to snap back to a predefined value when releasing the mouse button.
All values, notifiers, current value or min/max properties will act just like a slider or a rotary's properties, but nstead of a single number, a table with the fields
{x = xvalue, y = yvalue}
is expected, returned.+-------+ | o | | + | | | +-------+
Properties
active : ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
midi_mapping : ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
visible : ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
width : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
height : ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
tooltip : ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
views : renoise.Views.View
[]
READ-ONLY Empty for all controls, for layout views this contains the layout child views in the order they got added
min : XYPadMinValues
A table of allowed minimum values for each axis
- Default: {x: 0.0, y: 0.0}
max : XYPadMaxValues
A table of allowed maximum values for each axis
- Default: {x: 1.0, y: 1.0}
value : XYPadValues
A table of the XYPad's current values on each axis
snapback : XYPadSnapbackValues
?
A table of snapback values for each axis When snapback is enabled, the pad will revert its values to the specified snapback values as soon as the mouse button is released in the pad. When disabled, releasing the mouse button will not change the value. You can disable snapback at runtime by setting it to nil or an empty table.
Functions
add_child(self, child : renoise.Views.View
)
Add a new child view to this view.
remove_child(self, child : renoise.Views.View
)
Remove a child view from this view.
add_notifier(self, notifier : XYValueNotifierFunction
)
Add value change notifier
remove_notifier(self, notifier : XYValueNotifierFunction
)
Remove value change notifier
Local Aliases
ControlActive
Instead of making a control invisible, you can also make it inactive. Deactivated controls will still be shown, and will still show their currently assigned values, but will not allow changes. Most controls will display as "grayed out" to visualize the deactivated state.
ControlMidiMappingString
When set, the control will be highlighted when Renoise's MIDI mapping dialog is open. When clicked, it selects the specified string as a MIDI mapping target action. This target acton can either be one of the globally available mappings in Renoise, or those that were created by the tool itself. Target strings are not verified. When they point to nothing, the mapped MIDI message will do nothing and no error is fired.
SliderMaxValue
The maximum value that can be set using the view
- Default: 1.0
SliderMinValue
The minimum value that can be set using the view
- Default: 0
SliderNumberValue
The current value of the view
ViewDimension
The dimensions of a view has to be larger than 0. For nested views you can also specify relative size for example
vb:text { width = "80%"}
. The percentage values are relative to the view's parent size and will automatically update on size changes.
ViewTooltip
A tooltip text that should be shown for this view on mouse hover.
- Default: "" (no tip will be shown)
ViewVisibility
Set visible to false to hide a view (make it invisible without removing it). Please note that view.visible will also return false when any of its parents are invisible (when its implicitly invisible).
- Default: true
XYPadMaxValues
{ x : SliderMaxValue
, y : SliderMaxValue
}
A table of allowed maximum values for each axis
- Default: {x: 1.0, y: 1.0}
XYPadMinValues
{ x : SliderMinValue
, y : SliderMinValue
}
A table of allowed minimum values for each axis
- Default: {x: 0.0, y: 0.0}
XYPadSnapbackValues
A table of snapback values for each axis When snapback is enabled, the pad will revert its values to the specified snapback values as soon as the mouse button is released in the pad. When disabled, releasing the mouse button will not change the value. You can disable snapback at runtime by setting it to nil or an empty table.
XYPadValues
{ x : SliderNumberValue
, y : SliderNumberValue
}
A table of the XYPad's current values on each axis
XYValueNotifierFunction
(value : XYPadValues
)
#Lua Standard Modules
bit
Integer, Bit Operations, provided by http://bitop.luajit.org/
- Functions
- tobit(x :
integer
) - tohex(x :
integer
, n :integer``?
) - bnot(x :
integer
) - band(x1 :
integer
, ...integer
) - bor(x1 :
integer
, ...integer
) - xor(x1 :
integer
, ...integer
) - lshift(x :
integer
, n :integer
) - rshift(x :
integer
, n :integer
) - arshift(x :
integer
, n :integer
) - rol(x :
integer
, n :integer
) - ror(x :
integer
, n :integer
) - bswap(x :
integer
)
- tobit(x :
Functions
tobit(x : integer
)
->
integer
Normalizes a number to the numeric range for bit operations and returns it. This function is usually not needed since all bit operations already normalize all of their input arguments. Check the operational semantics for details.
tohex(x : integer
, n : integer
?
)
Converts its first argument to a hex string. The number of hex digits is given by the absolute value of the optional second argument. Positive numbers between 1 and 8 generate lowercase hex digits. Negative numbers generate uppercase hex digits. Only the least-significant 4*|n| bits are used. The default is to generate 8 lowercase hex digits.
bnot(x : integer
)
->
integer
Returns the bitwise not of its argument.
band(x1 : integer
, ...integer
)
->
integer
Returns the bitwise and of all of its arguments. Note that more than two arguments are allowed.
bor(x1 : integer
, ...integer
)
->
integer
Returns the bitwise or of all of its arguments. Note that more than two arguments are allowed.
xor(x1 : integer
, ...integer
)
->
integer
Returns the bitwise xor of all of its arguments. Note that more than two arguments are allowed.
lshift(x : integer
, n : integer
)
->
integer
Returns the bitwise logical left-shift of its first argument by the number of bits given by the second argument.
Logical shifts treat the first argument as an unsigned number and shift in 0-bits. Arithmetic right-shift treats the most-significant bit as a sign bit and replicates it. Only the lower 5 bits of the shift count are used (reduces to the range [0..31]).
rshift(x : integer
, n : integer
)
->
integer
Returns the bitwise logical right-shift of its first argument by the number of bits given by the second argument.
Logical shifts treat the first argument as an unsigned number and shift in 0-bits. Arithmetic right-shift treats the most-significant bit as a sign bit and replicates it. Only the lower 5 bits of the shift count are used (reduces to the range [0..31]).
arshift(x : integer
, n : integer
)
->
integer
Returns the bitwise arithmetic right-shift of its first argument by the number of bits given by the second argument.
Logical shifts treat the first argument as an unsigned number and shift in 0-bits. Arithmetic right-shift treats the most-significant bit as a sign bit and replicates it. Only the lower 5 bits of the shift count are used (reduces to the range [0..31]).
rol(x : integer
, n : integer
)
->
integer
Returns bitwise left rotation of its first argument by the number of bits given by the second argument. Bits shifted out on one side are shifted back in on the other side.
Only the lower 5 bits of the rotate count are used (reduces to the range [0..31]).
ror(x : integer
, n : integer
)
->
integer
Returns bitwise right rotation of its first argument by the number of bits given by the second argument. Bits shifted out on one side are shifted back in on the other side.
Only the lower 5 bits of the rotate count are used (reduces to the range [0..31]).
bswap(x : integer
)
->
integer
Swaps the bytes of its argument and returns it. This can be used to convert little-endian 32 bit numbers to big-endian 32 bit numbers or vice versa.
debug
Functions
start()
Shortcut to remdebug.session.start(), which starts a debug session: launches the debugger controller and breaks script execution. See "Debugging.md" in the documentation root folder for more info.
stop()
Shortcut to remdebug.session.stop: stops a running debug session
global
Functions
tostring(pattern_line : renoise.PatternLine
)
->
string
Serialize a line.
ripairs(table : <T:table>)
->
fun(table: integer
An iterator like ipairs, but in reverse order.
examples:
t = {"a", "b", "c"} for k,v in ripairs(t) do print(k, v) end -> "3 c, 2 b, 1 a"
objinfo(object : userdata
)
->
string
[]
Return a string which lists properties and methods of class objects.
oprint(object : userdata
)
Dumps properties and methods of class objects (like renoise.app()).
rprint(value : any
)
Recursively dumps a table and all its members to the std out (console). This works for standard Lua types and class objects as well.
class(name : string
)
->
function
Luabind "class" registration. Registers a global class object and returns a closure to optionally set the base class.
See also Luabind class
examples
---@class Animal -- Construct a new animal with the given name. ---@overload fun(string): Animal Animal = {} class 'Animal' ---@param name string function Animal:__init(name) self.name = name self.can_fly = nil end function Animal:show() return ("I am a %s (%s) and I %s fly"):format(self.name, type(self), (self.can_fly and "can fly" or "can not fly")) end -- Mammal class (inherits Animal functions and members) ---@class Mammal : Animal -- Construct a new mamal with the given name. ---@overload fun(string): Mammal Mammal = {} class 'Mammal' (Animal) ---@param name string function Mammal:__init(name) Animal.__init(self, name) self.can_fly = false end -- show() function and base member are available for Mammal too local mamal = Mammal("Cow") mamal:show()
type(value : any
)
->
string
Returns a Renoise class object's type name. For all other types the standard Lua type function is used.
examples:
class "MyClass"; function MyClass:__init() end print(type(MyClass)) -> "MyClass class" print(type(MyClass())) -> "MyClass"
rawequal(obj1 : any
, obj2 : any
)
Also compares object identities of Renoise API class objects. For all other types the standard Lua rawequal function is used.
examples:
print(rawequal(renoise.app(), renoise.app())) --> true print(rawequal(renoise.song().track[1], renoise.song().track[1]) --> true print(rawequal(renoise.song().track[1], renoise.song().track[2]) --> false
io
Functions
exists(filename : string
)
->
boolean
Returns true when a file, folder or link at the given path and name exists
stat(filename : string
)
->
result : Stat
?
, error : string
?
, error_code : integer
?
Returns a table with status info about the file, folder or link at the given path and name, else nil the error and the error code is returned.
chmod(filename : string
, mode : integer
)
->
result : boolean
, error : string
?
, error_code : integer
?
Change permissions of a file, folder or link. mode is a unix permission styled octal number (like 755 - WITHOUT a leading octal 0). Executable, group and others flags are ignored on windows and won't fire errors
Local Structs
Stat
return value for io.stat
Properties
dev : integer
device number of filesystem
ino : integer
inode number
mode : integer
unix styled file permissions
type : "file"
| "directory"
| "link"
| "socket"
| "named pipe"
| "char device"
| "block device"
nlink : integer
number of (hard) links to the file
uid : integer
numeric user ID of file's owner
gid : integer
numeric group ID of file's owner
rdev : integer
the device identifier (special files only)
size : integer
total size of file, in bytes
atime : integer
last access time in seconds since the epoch
mtime : integer
last modify time in seconds since the epoch
ctime : integer
inode change time (NOT creation time!) in seconds
math
Functions
lin2db(n : number
)
->
number
Converts a linear value to a db value. db values will be clipped to math.infdb.
examples:
print(math.lin2db(1.0)) --> 0 print(math.lin2db(0.0)) --> -200 (math.infdb)
db2lin(n : number
)
->
number
Converts a dB value to a linear value.
examples:
print(math.db2lin(math.infdb)) --> 0 print(math.db2lin(6.0)) --> 1.9952623149689
db2fader(min_dB : number
, max_dB : number
, dB_to_convert : number
)
->
number
Converts a dB value to a normalized linear fader value between 0-1 within the given dB range.
examples:
print(math.db2fader(-96, 0, 1)) --> 0 print(math.db2fader(-48, 6, 0)) --> 0.73879611492157
fader2db(min_dB : number
, max_dB : number
, fader_value : number
)
->
number
Converts a normalized linear mixer fader value to a db value within the given dB range.
examples:
print(math.fader2db(-96, 0, 1)) --> 0 print(math.fader2db(-96, 0, 0)) --> -96
os
Functions
platform()
->``"LINUX"
| "MACINTOSH"
| "WINDOWS"
Returns the platform the script is running on:
return #1: | "WINDOWS" | "MACINTOSH" | "LINUX"
currentdir()
->
path : string
Returns the current working dir. Will always be the scripts directory when executing a script from a file
dirnames(path : any
)
->
paths : string
[]
Returns a list of directory names (names, not full paths) for the given parent directory. Passed directory must be valid, or an error will be thrown.
filenames(path : string
, file_extensions : string
[]?
)
->
paths : string
[]
Returns a list file names (names, not full paths) for the given parent directory. Second optional argument is a list of file extensions that should be searched for, like {".wav", ".txt"}. By default all files are matched. The passed directory must be valid, or an error will be thrown.
mkdir(path : string
)
Creates a new directory. mkdir can only create one new sub directory at the same time. If you need to create more than one sub dir, call mkdir multiple times. Returns true if the operation was successful; in case of error, it returns nil plus an error string.
move(src : string
, dest : string
)
Moves a file or a directory from path 'src' to 'dest'. Unlike 'os.rename' this also supports moving a file from one file system to another one. Returns true if the operation was successful; in case of error, it returns nil plus an error string.
tmpname(extension : string
?
)
->
string
Changed in Renoises: Returns a temp directory and name which renoise will clean up on exit.
clock()
->
number
Replaced with a high precision timer (still expressed in milliseconds)
exit()
Will not exit, but fire an error that os.exit() can not be called
table
Functions
create(t : table
?
)
->
table
| tablelib
Create a new, or convert an exiting table to an object that uses the global 'table.XXX' functions as methods, just like strings in Lua do.
examples:
t = table.create(); t:insert("a"); rprint(t) -> [1] = a; t = table.create{1,2,3}; print(t:concat("|")); -> "1|2|3";
is_empty(t : table
)
->
boolean
Returns true when the table is empty, else false and will also work for non indexed tables
examples:
t = {}; print(table.is_empty(t)); -> true; t = {66}; print(table.is_empty(t)); -> false; t = {["a"] = 1}; print(table.is_empty(t)); -> false;
count(t : table
)
Count the number of items of a table, also works for non index based tables (using pairs).
examples:
t = {["a"]=1, ["b"]=1}; print(table.count(t)) --> 2
find(t : table
, value : any
, start_index : integer
?
)
->
key_or_nil : string
| number
?
Find first match of value in the given table, starting from element number start_index.
Returns the first key that matches the value or nilexamples:
t = {"a", "b"}; table.find(t, "a") --> 1 t = {a=1, b=2}; table.find(t, 2) --> "b" t = {"a", "b", "a"}; table.find(t, "a", 2) --> "3" t = {"a", "b"}; table.find(t, "c") --> nil
keys(t : table
)
->
table
Return an indexed table of all keys that are used in the table.
examples:
t = {a="aa", b="bb"}; rprint(table.keys(t)); --> "a", "b" t = {"a", "b"}; rprint(table.keys(t)); --> 1, 2
values(t : table
)
->
table
Return an indexed table of all values that are used in the table
examples:
t = {a="aa", b="bb"}; rprint(table.values(t)); --> "aa", "bb" t = {"a", "b"}; rprint(table.values(t)); --> "a", "b"
copy(t : table
)
->
table
Copy the metatable and all first level elements of the given table into a new table. Use table.rcopy to do a recursive copy of all elements
rcopy(t : table
)
->
table
Deeply copy the metatable and all elements of the given table recursively into a new table - create a clone with unique references.
clear(t : table
)
Recursively clears and removes all table elements.
#Lua Builtin Types
any
A type for a dynamic argument, it can be anything at run-time.
boolean
A built-in type representing a boolean (true or false) value, see details
function
A built-in type representing functions, see details
integer
A helper type that represents whole numbers, a subset of number
lightuserdata
A built-in type representing a pointer, see details
nil
A built-in type representing a non-existant value, see details. When you see
?
at the end of types, it means they can be nil.
number
A built-in type representing floating point numbers, see details
self
A type that represents an instance that you call a function on. When you see a function signature starting with this type, you should use
:
to call the function on the instance, this way you can omit this first argument.local song = renoise.song() local first_pattern = song:pattern(1)
string
A built-in type representing a string of characters, see details
table
A built-in type representing associative arrays, see details
unknown
A dummy type for something that cannot be inferred before run-time.
userdata
A built-in type representing array values, see details.